Window Handling in Selenium: Guide for Test Automation in 2024
- Gunashree RS
- 4 hours ago
- 9 min read
Introduction to Window Handling in Selenium
In today's complex web applications, handling multiple windows and tabs is a common scenario that test automation engineers encounter regularly. Whether it's dealing with pop-ups, new tabs, or separate windows, proper window handling is crucial for creating robust automated tests. Selenium, the industry-standard automation framework, provides powerful capabilities for managing these scenarios effectively.
Window handling in Selenium allows testers to seamlessly navigate between different browser windows during test execution, mimicking real user interactions and ensuring comprehensive testing coverage. Without proper window handling techniques, automated tests can fail when applications open new windows or tabs, creating significant gaps in test coverage.
This comprehensive guide will walk you through everything you need to know about window handling in Selenium - from understanding the core concepts to implementing advanced techniques that will elevate your test automation scripts to professional standards.
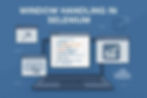
Understanding Window Handles in Selenium
Before diving into implementation details, it's essential to understand what window handles are and how Selenium uses them to identify and interact with multiple browser windows.
What is a Window Handle?
A window handle is a unique identifier that Selenium uses to reference a specific browser window or tab. Think of it as a special address or pointer that allows Selenium to distinguish between different windows during test execution. Each browser window or tab opened during a test session receives a unique alphanumeric ID (the window handle), which remains constant throughout the window's lifecycle.
These handles enable testers to:
Track all open windows during test execution
Switch focus between multiple windows
Perform operations on specific windows
Close particular windows without affecting others
Key Window Handle Methods in Selenium
Selenium's WebDriver provides several methods for working with window handles:
getWindowHandle(): Returns the handle of the current window as a string javaString currentWindowHandle = driver.getWindowHandle();
getWindowHandles(): Returns a set of handles for all open windows/tabs javaSet<String> allWindowHandles = driver.getWindowHandles();
switchTo().window(): Switches WebDriver's focus to a specific window javadriver.switchTo().window(windowHandleString);
Understanding these methods is fundamental to implementing effective window handling strategies in your Selenium tests.
Basic Window Handling Techniques in Selenium
Let's start with the essential techniques every test automation engineer should master for handling multiple windows.
Capturing the Parent Window Handle
When implementing window handling, it's best practice to first capture the parent (original) window handle before any new windows are opened:
java
// Store the handle of the original window
String parentWindowHandle = driver.getWindowHandle();
Storing this reference allows you to return to the original window later in your test script.
Switching Between Multiple Windows
When your application opens a new window, you'll need to switch to it to perform actions. Here's a common pattern for switching to a newly opened window:
java
// Store parent window handle
String parentWindow = driver.getWindowHandle();
// Click on element that opens new window
driver.findElement(By.id("openWindowButton")).click();
// Get all window handles
Set<String> allWindows = driver.getWindowHandles();
// Switch to new window
for (String windowHandle : allWindows) {
if (!windowHandle.equals(parentWindow)) {
driver.switchTo().window(windowHandle);
break;
}
}
// Perform actions in new window
driver.findElement(By.id("elementInNewWindow")).click();
// Switch back to parent window
driver.switchTo().window(parentWindow);
This pattern allows you to:
Identify which window is new
Switch to the new window
Perform necessary actions
Return to the original window
Handling Multiple Child Windows
Sometimes applications open multiple child windows. Here's how to handle such scenarios:
java
// Store parent window handle
String parentWindow = driver.getWindowHandle();
// Action that opens multiple windows
driver.findElement(By.id("openMultipleWindows")).click();
// Get all window handles
Set<String> allWindows = driver.getWindowHandles();
// Iterate through each window
for (String windowHandle : allWindows) {
if (!windowHandle.equals(parentWindow)) {
driver.switchTo().window(windowHandle);
// Identify which specific window this is by title or URL
if (driver.getTitle().contains("Expected Window Title")) {
// Perform actions specific to this window
driver.findElement(By.id("specificElement")).click();
}
}
}
// Return to parent window
driver.switchTo().window(parentWindow);
This approach allows you to identify specific windows by their properties and perform targeted actions.
Advanced Window Handling Strategies
Once you've mastered the basics, these advanced strategies will help you handle complex scenarios more efficiently.
Using Iterator for Window Navigation
Using an Iterator can make window handling code more readable and maintainable:
java
// Store parent window
String parentWindow = driver.getWindowHandle();
// Open new window
driver.findElement(By.id("newWindowButton")).click();
// Get window handles
Set<String> handles = driver.getWindowHandles();
Iterator<String> iterator = handles.iterator();
// Navigate through windows
while (iterator.hasNext()) {
String childWindow = iterator.next();
if (!parentWindow.equals(childWindow)) {
driver.switchTo().window(childWindow);
// Perform actions in child window
System.out.println("Child window title: " + driver.getTitle());
// Close child window (optional)
driver.close();
}
}
// Switch back to parent window
driver.switchTo().window(parentWindow);
This pattern is particularly useful when dealing with an unknown number of windows.
Handling Windows Using Window Properties
Sometimes it's more reliable to identify windows by their properties rather than their position in the set:
java
// Store parent window
String parentWindow = driver.getWindowHandle();
// Open new window
driver.findElement(By.id("newWindowButton")).click();
// Get all handles
Set<String> handles = driver.getWindowHandles();
// Find the desired window by URL or title
for (String handle : handles) {
driver.switchTo().window(handle);
// Switch to window with specific URL
if (driver.getCurrentUrl().contains("expected-url-fragment")) {
// We're in the right window, perform actions
break;
}
}
// Actions in the identified window
driver.findElement(By.id("targetElement")).click();
// Return to parent window
driver.switchTo().window(parentWindow);
This approach is more robust when the order of window opening isn't predictable.
Working with JavaScript Window Handling
In some scenarios, combining Selenium with JavaScript execution can provide more control:
java
// Execute JavaScript to open new window with specific dimensions
((JavascriptExecutor) driver).executeScript("window.open('https://example.com', 'newWindow', 'width=800,height=600');");
// Get all window handles
Set<String> handles = driver.getWindowHandles();
Iterator<String> iterator = handles.iterator();
// Get parent handle
String parentWindow = iterator.next();
// Switch to new window
String childWindow = iterator.next();
driver.switchTo().window(childWindow);
// Perform actions in new window
driver.findElement(By.id("elementInNewWindow")).click();
// Switch back to parent window
driver.switchTo().window(parentWindow);
This technique gives you precise control over the new window's properties.
Common Challenges and Solutions in Window Handling
Even experienced automation engineers encounter challenges with window handling. Here are some common issues and their solutions:
Challenge 1: NoSuchWindowException
This exception occurs when trying to switch to a window that no longer exists.
Solution:
java
try {
driver.switchTo().window(windowHandle);
} catch (NoSuchWindowException e) {
System.out.println("Window no longer exists: " + e.getMessage());
// Handle appropriately - perhaps switch back to parent window
driver.switchTo().window(parentWindowHandle);
}
Challenge 2: Timing Issues with New Windows
New windows may take time to open, causing synchronization problems.
Solution:
java
// Store initial window handles count
int initialHandles = driver.getWindowHandles().size();
// Click button that opens new window
driver.findElement(By.id("openWindowButton")).click();
// Wait for new window to appear
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(driver -> driver.getWindowHandles().size() > initialHandles);
// Now proceed with window handling
Set<String> handles = driver.getWindowHandles();
// Continue with your window handling logic...
Challenge 3: Identifying the Correct Window
When multiple windows open, identifying the correct one can be challenging.
Solution:
java
// Store original window
String originalWindow = driver.getWindowHandle();
// Click to open new window
driver.findElement(By.id("openButton")).click();
// Wait for new window and switch to it
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(driver -> driver.getWindowHandles().size() > 1);
// Switch to new window
for (String windowHandle : driver.getWindowHandles()) {
if (!originalWindow.equals(windowHandle)) {
driver.switchTo().window(windowHandle);
// Verify it's the correct window
wait.until(driver -> driver.getTitle().contains("Expected Title"));
break;
}
}
This combines explicit waits with window property verification for greater reliability.
Best Practices for Window Handling in Selenium
Follow these best practices to ensure your window handling code is robust and maintainable:
Always store the parent window handle at the beginning of your test
java
String parentWindow = driver.getWindowHandle();
Use explicit waits instead of Thread.sleep() when waiting for new windows.
java
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(driver -> driver.getWindowHandles().size() > 1);
Close child windows after finishing operations to avoid resource waste
java
driver.close(); // Closes current window
driver.switchTo().window(parentWindow); // Switch back to parent
Verify window properties after switching to ensure you're in the correct window.
java
// After switching to a window
Assert.assertTrue(driver.getTitle().contains("Expected Title"));
Handle windows by properties (title, URL) rather than by their order in the set.
java
// Find window by URL
for (String handle : driver.getWindowHandles()) {
driver.switchTo().window(handle);
if (driver.getCurrentUrl().contains("expected-url")) {
break; // Found the right window
}
}
Implement proper exception handling for window-related operations.
java
try {
driver.switchTo().window(windowHandle);
} catch (NoSuchWindowException e) {
// Handle the exception appropriately
}
Following these practices will make your window handling code more reliable and easier to maintain.
Real-World Example: E-commerce Testing Scenario
Let's put everything together in a real-world example. This scenario tests a common e-commerce flow where clicking on a product opens details in a new window:
java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.openqa.selenium.support.ui.WebDriverWait;
import java.time.Duration;
import java.util.Set;
public class EcommerceWindowHandlingExample {
public static void main(String[] args) {
// Setup WebDriver
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize();
try {
// Navigate to e-commerce site
driver.get("https://example-ecommerce.com");
// Store parent window handle
String parentWindow = driver.getWindowHandle();
// Search for a product
driver.findElement(By.id("searchBox")).sendKeys("laptop");
driver.findElement(By.id("searchButton")).click();
// Click on the product that opens in a new window
driver.findElement(By.cssSelector(".product-item:first-child")).click();
// Wait for new window to open
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(d -> d.getWindowHandles().size() > 1);
// Switch to product details window
Set<String> handles = driver.getWindowHandles();
for (String handle : handles) {
if (!handle.equals(parentWindow)) {
driver.switchTo().window(handle);
break;
}
}
// Verify we're on the correct product page
wait.until(d -> driver.getTitle().contains("Laptop"));
// Add product to cart
driver.findElement(By.id("addToCartButton")).click();
// Wait for cart confirmation
wait.until(d -> driver.findElement(By.id("cartConfirmation")).isDisplayed());
// Close product window
driver.close();
// Switch back to parent window
driver.switchTo().window(parentWindow);
// Navigate to cart
driver.findElement(By.id("cartIcon")).click();
// Verify product is in cart
wait.until(d -> driver.findElement(By.cssSelector(".cart-item")).isDisplayed());
} finally {
// Clean up
driver.quit();
}
}
}
This example demonstrates a complete test flow including:
Storing the parent window handle
Waiting for new windows
Switching between windows
Performing actions in different windows
Closing child windows
Returning to the parent window
Conclusion
Window handling is an essential skill for Selenium test automation engineers. By understanding window handles and mastering the techniques presented in this guide, you can create robust test scripts that seamlessly navigate between multiple windows and tabs, ensuring thorough testing of your applications.
Remember that effective window handling is all about maintaining control of which window has focus and being able to reliably switch between windows as needed. With practice and by following the best practices outlined here, you can overcome the common challenges and write professional-grade test automation scripts.
As web applications continue to become more complex, strong window handling skills will remain a crucial part of your test automation toolkit, enabling you to create comprehensive test coverage for even the most sophisticated user interfaces.
Key Takeaways
Window handles are unique identifiers that Selenium uses to reference browser windows and tabs.
Always store the parent window handle before opening new windows to ensure you can return to it.
Use getWindowHandle() for the current window and getWindowHandles() for all open windows.
The switchTo().window() method is essential for changing focus between different windows.
Implement explicit waits when dealing with new windows rather than using Thread.sleep()
Identify windows by their properties (title, URL) rather than their position in the set for more reliability.
Close unnecessary windows after use to manage resources efficiently
Implement proper exception handling for window-related operations to make scripts more robust
Combine window handling with JavaScript execution for more control over window behavior
Regular practice with different scenarios will strengthen your window handling skills
Frequently Asked Questions
What is window handling in Selenium?
Window handling in Selenium refers to the techniques and methods used to manage and interact with multiple browser windows or tabs during test automation. It involves capturing window handles (unique identifiers), switching between windows, and performing operations on specific windows. This capability is essential when testing applications that open pop-ups, new tabs, or separate windows during user interactions.
Why do we need window handling in Selenium?
Window handling is necessary because modern web applications frequently open new windows or tabs for various functions like product details, payment gateways, login portals, or documentation. Without proper window handling, Selenium would remain focused on the original window and be unable to interact with elements in the new windows, leading to test failures and incomplete coverage of application functionality.
What is the difference between getWindowHandle() and getWindowHandles() in Selenium?
The getWindowHandle() method returns a string containing the unique identifier (handle) of the currently active browser window or tab. In contrast, getWindowHandles() returns a Set of strings containing the handles of all browser windows/tabs currently open in the WebDriver session. The former gives you the current window's reference, while the latter gives you references to all open windows.
How do I switch back to the parent window after working with a child window?
To switch back to the parent window, you need to store its handle before opening any child windows. Then, after completing operations in the child window, you can use the switchTo().window() method with the stored parent handle:
java
// Store parent window handle
String parentWindow = driver.getWindowHandle();
// After finishing operations in child windowdriver.switchTo().window(parentWindow);
How can I handle browser pop-up windows in Selenium?
Browser pop-up windows can be handled using the window handling techniques. Store the parent window handle, wait for the pop-up to appear (the number of window handles will increase), and switch to the pop-up window using switchTo().window(), perform the necessary actions, close the pop-up if required, and switch back to the parent window. For alert pop-ups (JavaScript alerts), use Selenium's Alert interface instead of window handling.
What exceptions might occur during window handling in Selenium?
Common exceptions during window handling include:
NoSuchWindowException: Occurs when trying to switch to a window that doesn't exist or has been closed
TimeoutException: When using explicit waits, if a new window doesn't appear within the specified timeout
StaleElementReferenceException: Can occur if you try to interact with elements after switching windows and then switching back
Can I handle multiple tabs and windows simultaneously in Selenium?
Selenium can only interact with one window/tab at a time. To work with multiple windows or tabs, you need to switch between them using their window handles. While you can't simultaneously perform actions in different windows, you can sequentially switch between them to perform operations in each one during a test script.
Is there a way to open a new tab programmatically in Selenium?
Yes, you can open a new tab programmatically using JavaScript execution:
java
// Open new tab
((JavascriptExecutor) driver).executeScript("window.open()");
// Switch to the new tab (will be the last handle in the set)
ArrayList<String> tabs = new ArrayList<>(driver.getWindowHandles());
driver.switchTo().window(tabs.get(tabs.size() - 1));
// Navigate to a URL in a new tab
driver.get("https://example.com");