Mastering Page Object Model in Selenium: Guide for Test Automation
- Gunashree RS
- 7 hours ago
- 8 min read
Introduction to Page Object Model in Selenium
In the ever-evolving world of test automation, maintaining clean, efficient, and reusable code becomes increasingly important as projects grow in complexity. The Page Object Model (POM) has emerged as one of the most effective design patterns for Selenium WebDriver-based automation frameworks, helping teams overcome common challenges in test maintenance and scalability.
Page Object Model is a design pattern that creates an object repository for storing all web elements. It separates test methods from page-specific elements, creating a layer of abstraction that makes test code more maintainable and readable. This approach treats each web page of an application as a separate class file, containing only the corresponding web elements and methods to interact with them.
As automation projects grow larger, the benefits of implementing POM become increasingly evident. Not only does it reduce code duplication, but it also provides a structured approach to organizing test code that aligns with the application's architecture. This makes it easier for teams to collaborate, update tests when the UI changes, and expand test coverage across the application.
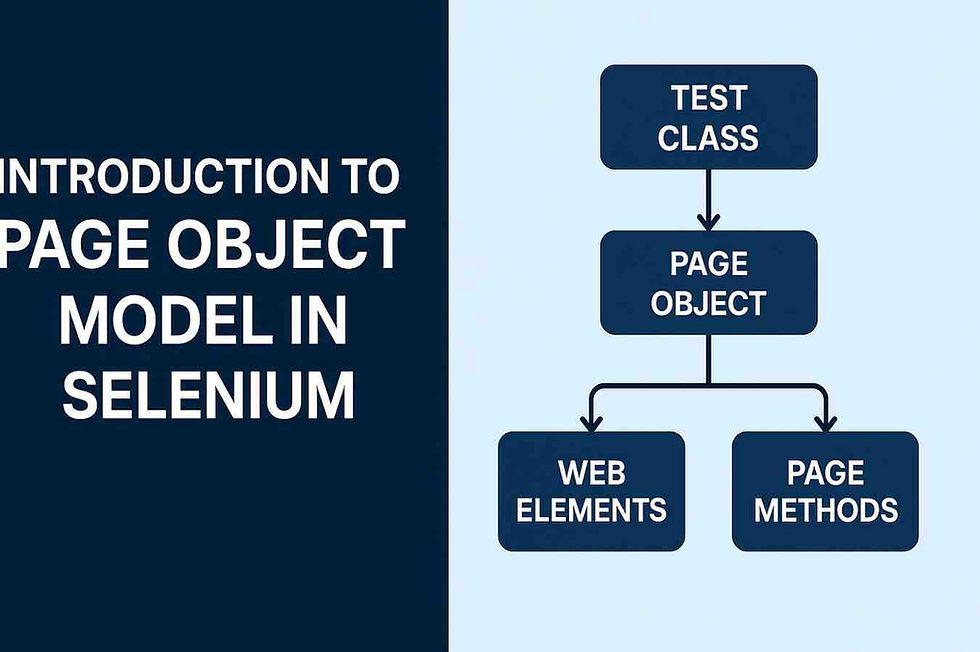
Core Concepts of Page Object Model
The Basic Structure
The fundamental concept behind POM is simple yet powerful: each web page in your application gets its own class. These classes encapsulate the functionality and elements specific to that page. The structure typically includes:
Page Objects: Java classes that represent web pages
Web Elements: Object repositories for UI elements on each page
Page Methods: Functions that perform operations using these elements
Test Classes: Separate classes that use page objects to execute test flows
This separation creates a clean division between test logic and page-specific implementation details. When elements on a page change, you only need to update the corresponding page class, while your test methods remain untouched.
Element Identification and Operations
In a traditional Page Object Model implementation, elements are typically identified using the By locator mechanism:
By Header = By.xpath("//h1");
By getStarted = By.xpath("//*[@id='signupModalButton']");
These elements are then used within methods that perform actions on the page:
public void verifyHeader() {
String getHeaderText = driver.findElement(Header).getText();
assertEquals("Expected Header Text", getHeaderText);
}
public void clickOnGetStarted() {
driver.findElement(getStarted).click();
}
This approach creates a clean API for interacting with web pages, making test scripts more readable and intuitive.
Advantages of Implementing Page Object Model
The adoption of POM offers several significant benefits that impact both the development process and long-term maintenance of test automation code:
1. Enhanced Maintenance
When UI elements change, which happens frequently in modern web development, POM significantly reduces the maintenance burden:
Changes are isolated to specific page classes
Test scripts remain stable despite UI updates
Modifications are made in one place rather than across multiple test cases
For example, if a login button changes from an ID to an XPath selector, you only need to update it in the Login Page class, rather than in every test that performs a login action.
2. Improved Code Reusability
POM promotes efficient code reuse through:
Shared page objects across multiple test cases
Common functionality encapsulated in reusable methods
Standardized approaches to similar interactions
This means that once you've created a page object for your application's login page, any test requiring login functionality can leverage that existing code without duplication.
3. Better Readability and Structure
Test scripts using POM are inherently more readable:
Business logic is separated from technical implementation
Test methods reflect user workflows rather than element manipulation
Clear naming conventions make code self-documenting
Consider the difference between these two approaches:
Without POM:
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password123");
driver.findElement(By.id("loginButton")).click();
With POM:
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername("testuser");
loginPage.enterPassword("password123");
loginPage.clickLoginButton();
The POM version clearly communicates the intent of the code and hides the technical details of element location and interaction.
Implementing Page Object Model in Selenium
Creating a Basic POM Structure
Let's examine how to implement a basic POM structure for a sample application. We'll use a simple website with a home page and a sign-up page as our example.
First, we need to create our page classes:
package browserStackPages;
import static org.testng.Assert.assertEquals;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class BrowserStackHomePage {
WebDriver driver;
By Header = By.xpath("//h1");
By getStarted = By.xpath("//*[@id='signupModalButton']");
public BrowserStackHomePage(WebDriver driver) {
this.driver = driver;
}
public void verifyHeader() {
String getHeaderText = driver.findElement(Header).getText();
assertEquals("App & Browser Testing Made Easy", getHeaderText);
}
public void clickOnGetStarted() {
driver.findElement(getStarted).click();
}
}
package browserStackPages;
import static org.testng.Assert.assertEquals;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class BrowserStackSignUpPage {
WebDriver driver;
By Header = By.xpath("//h1");
By userName = By.xpath("//*[@id='user_full_name']");
By businessEmail = By.xpath("//*[@id='user_email_login']");
By password = By.xpath("//*[@id='user_password']");
public BrowserStackSignUpPage(WebDriver driver) {
this.driver = driver;
}
public void verifyHeader() {
String getHeaderText = driver.findElement(Header).getText().trim();
assertEquals("Create a FREE Account", getHeaderText);
}
public void enterFullName(String arg1) {
driver.findElement(userName).sendKeys(arg1);
}
public void enterBusinessEmail(String arg1) {
driver.findElement(businessEmail).sendKeys(arg1);
}
public void enterPassword(String arg1) {
driver.findElement(password).sendKeys(arg1);
}
}
Next, we create our test class that uses these page objects:
package browserStackSetup;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import browserStackPages.BrowserStackHomePage;
import browserStackPages.BrowserStackSignUpPage;
public class BrowserStackSetup {
String driverPath = "C:\\geckodriver.exe";
WebDriver driver;
BrowserStackHomePage objBrowserStackHomePage;
BrowserStackSignUpPage objBrowserStackSignUpPage;
@BeforeTest
public void setup() {
System.setProperty("webdriver.chrome.driver", "C:\\BrowserStack\\chromedriver.exe");
driver = new ChromeDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.browserstack.com/");
}
@Test(priority = 1)
public void navigate_to_homepage_click_on_getstarted() {
objBrowserStackHomePage = new BrowserStackHomePage(driver);
objBrowserStackHomePage.verifyHeader();
objBrowserStackHomePage.clickOnGetStarted();
}
@Test(priority = 2)
public void enter_userDetails() {
objBrowserStackSignUpPage = new BrowserStackSignUpPage(driver);
objBrowserStackSignUpPage.verifyHeader();
objBrowserStackSignUpPage.enterFullName("TestUser");
objBrowserStackSignUpPage.enterBusinessEmail("TestUser@gmail.com");
objBrowserStackSignUpPage.enterPassword("TestUserPassword");
}
}
Enhancing POM with Page Factory
While basic POM implementation provides significant benefits, Selenium also offers the Page Factory enhancement, which further streamlines element initialization and handling.
What is Page Factory?
Page Factory is a built-in class provided by Selenium WebDriver that optimizes the implementation of the Page Object Model. It introduces several key features:
@FindBy Annotation: A more concise way to locate web elements
Lazy Initialization: Elements are only initialized when they're actually used
InitElements Method: A centralized way to initialize all elements on a page
Page Factory Implementation
Let's refactor our previous example to use Page Factory:
BrowserStackHomePage with Page Factory:
package browserStackPages;
import static org.testng.Assert.assertEquals;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class BrowserStackHomePage {
WebDriver driver;
@FindBy(xpath = "//h1")
WebElement Header;
@FindBy(xpath = "//*[@id='signupModalButton']")
WebElement getStarted;
public BrowserStackHomePage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void verifyHeader() {
String getHeaderText = Header.getText();
assertEquals("App & Browser Testing Made Easy", getHeaderText);
}
public void clickOnGetStarted() {
getStarted.click();
}
}
The key differences with Page Factory are:
Elements are defined as WebElement fields with @FindBy annotations
PageFactory.initElements() initializes all elements
Direct element references replace the driver.findElement() calls
This approach results in cleaner, more maintainable code that achieves the same functionality with less boilerplate.
Comparing Page Object Model and Page Factory
While both approaches implement the same design pattern, they have distinct characteristics:
Feature | Page Object Model | Page Factory |
Element Finding | Using By locators | Using @FindBy annotation |
Initialization | Manual initialization for each element | Batch initialization with initElements() |
Lazy Loading | Not supported | Supported via AjaxElementLocatorFactory |
Code Conciseness | More verbose | More concise |
Flexibility | More control over element finding | More declarative approach |
Both approaches are valid implementations of the Page Object design pattern, and the choice between them often comes down to team preference or specific project requirements.
Best Practices for Page Object Model Implementation
To maximize the benefits of POM in your Selenium projects, consider these best practices:
Keep Page Objects Focused: Each page class should represent a single page or component
Return Page Objects from Methods: When navigation occurs, return the new page object:
public SignUpPage clickSignUp()
{ signUpButton.click();
return new SignUpPage(driver);
}
Abstract Common Functionality: Create base page classes for shared functionality
Use Meaningful Names: Name methods according to business actions rather than technical steps.
Avoid Assertions in Page Objects: Page objects should focus on interactions, while test classes handle assertions.
Beyond Basic POM: Advanced Patterns
As your test automation framework matures, you might explore these advanced patterns that build upon POM:
Fluent Page Object Model
Fluent POM uses method chaining to create more readable test flows:
new LoginPage(driver)
.enterUsername("testuser")
.enterPassword("password123")
.clickLoginButton()
.verifyDashboardIsDisplayed();
Singleton Design Pattern with POM
The Singleton pattern ensures that only one instance of each page object exists throughout the test execution:
public class HomePage {
private static HomePage instance;
private WebDriver driver;
private HomePage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public static HomePage getInstance(WebDriver driver) {
if(instance == null) {
instance = new HomePage(driver);
}
return instance;
}
}
Conclusion
The Page Object Model has become a standard approach for structuring Selenium test automation frameworks, and for good reason. By separating page-specific code from test logic, POM creates more maintainable, readable, and robust test suites that can evolve alongside the application under test.
Whether you choose the traditional POM approach or enhance it with Page Factory, the core principles remain the same: encapsulation, separation of concerns, and creating an abstraction layer that shields test code from implementation details.
As your automation projects grow in complexity, the investment in setting up a proper Page Object Model will pay dividends in reduced maintenance costs, improved code reuse, and more efficient test development.
Key Takeaways
Page Object Model creates separate class files for each web page, containing elements and actions for that page.
POM significantly improves test maintenance by isolating UI changes to specific page classes
Code reusability is enhanced as page objects can be shared across multiple test cases.
Page Factory extends POM with @FindBy annotations and lazy initialization for cleaner code.
Return page objects from methods when navigation occurs to enable method chaining
Fluent POM and Singleton patterns can further enhance your POM implementation.
POM separates what to test (test classes) from how to interact (page objects)
Proper implementation of POM leads to more stable test suites that are easier to maintain
Frequently Asked Questions
What is the difference between Page Object Model and Page Factory in Selenium?
Page Object Model is a design pattern that separates page elements and actions into dedicated classes, while Page Factory is a built-in Selenium class that implements POM with additional features like @FindBy annotations and lazy initialization. Page Factory provides a more optimized way to implement POM.
How do I implement the Page Object Model in Selenium Python?
In Python, you would create separate classes for each page, define elements using either By locators or find_element methods, and create methods for page actions. The structure is similar to Java, though Python doesn't have a direct equivalent to Java's Page Factory.
What are the advantages of using the Page Object Model for test automation?
The key advantages include improved test maintenance (changes are isolated to specific page classes), enhanced code reuse (page objects can be shared across tests), better readability (business logic is separated from technical implementation), and easier collaboration (team members can work on different page objects independently).
Should I use assertions in my Page Object classes?
Best practice suggests keeping assertions out of page object classes. Page objects should focus on interactions and return values that test classes can assert against. This maintains a cleaner separation between the "how" (page objects) and the "what" (test assertions).
How do I handle multiple browsers with the Page Object Model?
The WebDriver instance is typically passed to page object constructors, allowing the same page objects to work with different browser instances. This approach allows tests to run against multiple browsers without changing the page object implementation.
What's the best way to structure a large test automation project using POM?
Large projects benefit from organizing page objects into packages that mirror the application's structure. Consider creating base page classes for common functionality, implementing utility classes for shared operations, and using configuration management to handle environment-specific details.
Comments