Fluent Wait in Selenium: Master Dynamic Element Handling for Robust Automation
- Gunashree RS
- 21 hours ago
- 6 min read
Your Ultimate Guide to Fluent Wait in Selenium
When developing automated tests with Selenium WebDriver, one of the most common challenges is handling elements that don't load immediately. Whether due to AJAX calls, JavaScript rendering, or network delays, elements often require varying amounts of time to become available for interaction. This is where the Fluent Wait mechanism in Selenium becomes invaluable.
Selenium offers several waiting strategies to address these timing issues, but Fluent Wait stands out as the most flexible and powerful option. Unlike its counterparts, Fluent Wait allows testers to customize polling intervals, ignore specific exceptions, and define precise conditions for element readiness. This level of control makes it essential for creating stable, reliable automation frameworks.
In this comprehensive guide, we'll explore everything you need to know about implementing Fluent Wait effectively, including practical code examples, best practices, and common pitfalls to avoid.
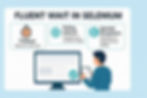
Understanding Wait Mechanisms in Selenium
Before diving deep into Fluent Wait, it's important to understand the wait mechanisms available in Selenium and how they differ from each other:
Types of Waits in Selenium
Implicit Wait: Sets a global timeout for all element searches.
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
Explicit Wait: Waits for a specific condition to be true before proceeding.
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("element")));
Fluent Wait: A more customizable form of explicit wait that allows setting polling intervals and ignoring specific exceptions.
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(5))
.ignoring(NoSuchElementException.class);
Limitations of Basic Waits
While Implicit and Explicit waits are useful in many scenarios, they have limitations:
Implicit Wait: Applies the same timeout globally to all elements, which is inefficient when different elements require different wait times.
Explicit Wait: More targeted than Implicit Wait but lacks the fine-grained control over polling frequency and exception handling.
This is precisely where Fluent Wait shines by offering greater flexibility and control.
What Makes Fluent Wait Special?
Fluent Wait is a specialized waiting mechanism that stands out due to its highly customizable nature. It allows you to define:
Maximum wait time: How long to wait before giving up
Polling frequency: How often to check for the condition
Exceptions to ignore: Which exceptions to disregard during the wait period
This combination of features makes it particularly effective for handling unpredictable elements in dynamic web applications.
Key Components of Fluent Wait
Component | Description | Example |
Timeout | Maximum duration to wait for a condition | .withTimeout(Duration.ofSeconds(30)) |
Polling Interval | Frequency of condition checks | .pollingEvery(Duration.ofSeconds(5)) |
Ignored Exceptions | Exceptions to bypass during polling | .ignoring(NoSuchElementException.class) |
Expected Condition | Condition that must be met | wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("example"))) |
Implementing Fluent Wait in Selenium: Practical Examples
Let's explore some practical implementations of Fluent Wait for various scenarios you might encounter in your automation projects.
Example 1: Waiting for Element Visibility
// Import necessary classes
import org.openqa.selenium.*;
import org.openqa.selenium.support.ui.*;
import java.time.Duration;
// Create a Fluent Wait instance
Wait<WebDriver> fluentWait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
// Wait for an element to be visible
WebElement element = fluentWait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElement")));
element.click();
Example 2: Creating Custom Wait Conditions
Sometimes, the built-in ExpectedConditions don't meet your specific requirements. In such cases, you can create custom conditions:
Wait<WebDriver> fluentWait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
// Custom condition: Wait until the element contains specific text
WebElement element = fluentWait.until(driver -> {
WebElement el = driver.findElement(By.id("statusMessage"));
if (el.getText().contains("Ready")) {
return el;
}
return null;
});
Example 3: Handling Multiple Conditions
For complex scenarios where you need to check multiple conditions:
Wait<WebDriver> fluentWait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
// Wait for button to be both visible and enabled
WebElement button = fluentWait.until(driver -> {
WebElement el = driver.findElement(By.id("submitButton"));
if (el.isDisplayed() && el.isEnabled()) {
return el;
}
return null;
});
Best Practices for Using Fluent Wait Effectively
To get the most out of Fluent Wait in your Selenium projects, consider the following best practices:
Use appropriate timeouts: Set realistic maximum wait times based on your application's behavior.
Choose optimal polling intervals: Balance between responsiveness and resource utilization.
Too frequent: Unnecessary CPU usage
Too infrequent: Could delay test execution
Be selective with ignored exceptions: Only ignore exceptions that are expected during the waiting period.
Create reusable wait methods: Implement utility methods for common wait patterns to reduce code duplication.
public WebElement waitForElementToBeClickable(By locator) {
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class);
return wait.until(ExpectedConditions.elementToBeClickable(locator));
}
Add meaningful error messages: Customize error messages to make debugging easier.
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(30))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(NoSuchElementException.class)
.withMessage("Element with ID 'submitButton' was not clickable after 30 seconds");
Common Pitfalls and How to Avoid Them
Even with Fluent Wait, there are several pitfalls that can affect your test stability:
1. Ignoring Too Many Exceptions
While it's useful to ignore certain exceptions like NoSuchElementException, ignoring too many can mask real issues in your application or test scripts.
Solution: Only ignore exceptions that are expected during normal operation of your application.
2. Setting Unrealistic Timeouts
Setting timeouts that are too short can lead to flaky tests, while timeouts that are too long can unnecessarily slow down your test suite.
Solution: Monitor your application's performance under different conditions and set timeouts accordingly.
3. Not Handling StaleElementReferenceException
Elements can become stale when the DOM is updated after the element is found but before it's interacted with.
Solution: Implement retry mechanisms for operations that might encounter stale elements.
public void clickWithRetry(By locator, int maxRetries) {
int attempts = 0;
while (attempts < maxRetries) {
try {
Wait<WebDriver> wait = new FluentWait<>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofSeconds(1));
WebElement element = wait.until(ExpectedConditions.elementToBeClickable(locator));
element.click();
return;
} catch (StaleElementReferenceException e) {
attempts++;
if (attempts == maxRetries) {
throw e;
}
}
}
}
When to Choose Fluent Wait Over Other Wait Types
Fluent Wait is particularly valuable in the following scenarios:
Dynamic content loading: When elements appear at unpredictable times due to AJAX or JavaScript execution.
Network variability: When testing applications that might experience network latency.
Complex conditions: When you need to wait for complex conditions that aren't covered by standard expected conditions.
Resource-intensive applications: When you want to reduce the frequency of DOM checks to minimize resource usage.
However, for simpler scenarios where elements consistently appear after a fixed delay, Explicit Wait might be sufficient and more straightforward to implement.
Conclusion
Fluent Wait is a powerful tool in the Selenium WebDriver arsenal that provides unparalleled flexibility for handling dynamic elements. By allowing customization of timeout durations, polling intervals, and exception handling, it enables testers to create more robust and reliable automation scripts.
Mastering Fluent Wait is essential for developing stable test automation frameworks, especially when dealing with modern web applications that rely heavily on asynchronous operations and dynamic content loading. By following the best practices and examples outlined in this guide, you'll be well-equipped to tackle even the most challenging timing issues in your Selenium projects.
Key Takeaways
Fluent Wait provides fine-grained control over waiting conditions with customizable timeouts and polling intervals.
Custom conditions can be created for complex scenarios beyond standard ExpectedConditions
Proper exception handling is crucial for reliable test automation
Reusable wait utility methods improve code maintainability
Balancing timeout durations and polling frequencies is essential for optimal test performance
Fluent Wait is ideal for dynamic web applications with unpredictable element loading times
FAQ: Common Questions About Fluent Wait in Selenium
What is the difference between Explicit Wait and Fluent Wait in Selenium?
Explicit Wait is a type of wait that pauses execution until a specific condition is met or the timeout is reached. Fluent Wait is an extension of Explicit Wait that adds customizable polling intervals and the ability to ignore specific exceptions during the
wait period.
Can Fluent Wait replace Implicit Wait entirely?
Yes, in most cases, Fluent Wait can replace Implicit Wait with more precise control. However, some testers use a minimal Implicit Wait as a safety net while primarily relying on Fluent Wait for specific elements.
How do I determine the optimal polling interval for Fluent Wait?
The optimal polling interval depends on your application's behavior. For most web applications, polling every 500ms to 2 seconds offers a good balance between responsiveness and resource usage.
Can Fluent Wait be used with all browsers supported by Selenium?
Yes, Fluent Wait works consistently across all browsers supported by Selenium WebDriver, including Chrome, Firefox, Edge, and Safari.
Is it possible to wait for multiple elements with a single Fluent Wait?
Yes, you can create custom conditions that check for the presence or state of multiple elements before returning.
How can I debug issues with Fluent Wait when elements aren't being detected?
Add logging within custom wait conditions, use the withMessage() method to provide descriptive timeout messages, and consider taking screenshots at regular intervals during the wait period.
Does Fluent Wait consume more resources than other wait types?
When configured with appropriate polling intervals, Fluent Wait can actually be more resource-efficient than other wait types that might check conditions more frequently.
Can Fluent Wait be used in conjunction with Page Object Model?
Absolutely! Fluent Wait integrates perfectly with the Page Object Model pattern, typically implemented as utility methods within page objects or in a separate wait utilities class.
Article Sources
Selenium Official Documentation - Waits
Test Automation University - Advanced Selenium WebDriver Techniques
Stack Overflow - Thread on Fluent Wait Best Practices
GitHub - Selenium Wait Patterns Repository
BrowserStack Documentation - Handling Synchronization in Selenium
JavaDocs - FluentWait Class Documentation