Guide to Selenium Identifiers: Mastering Web Element Location
- Gunashree RS
- May 20
- 8 min read
Introduction to Selenium Identifiers
In the world of test automation, Selenium WebDriver has established itself as the go-to framework for web application testing. At the heart of Selenium's functionality lies its ability to interact with web elements—buttons, text fields, dropdowns, and other HTML components that make up a web page. To interact with these elements, Selenium needs to locate them first, and that's where Selenium identifiers come into play.
Selenium identifiers, also known as locators, are commands that tell WebDriver how to find a particular element within the DOM (Document Object Model) structure of a webpage. Think of them as addresses that pinpoint the exact location of elements you want to interact with during test execution.
Choosing the right locator strategy is crucial for creating robust, maintainable test scripts. Poor locator choices can lead to brittle tests that break easily when the application under test undergoes even minor changes. On the other hand, well-crafted locators make your tests more stable and reliable across different browsers and application versions.
In this comprehensive guide, we'll explore the various types of Selenium identifiers, their pros and cons, best practices for using them, and practical examples to help you master the art of element location in your test automation projects.
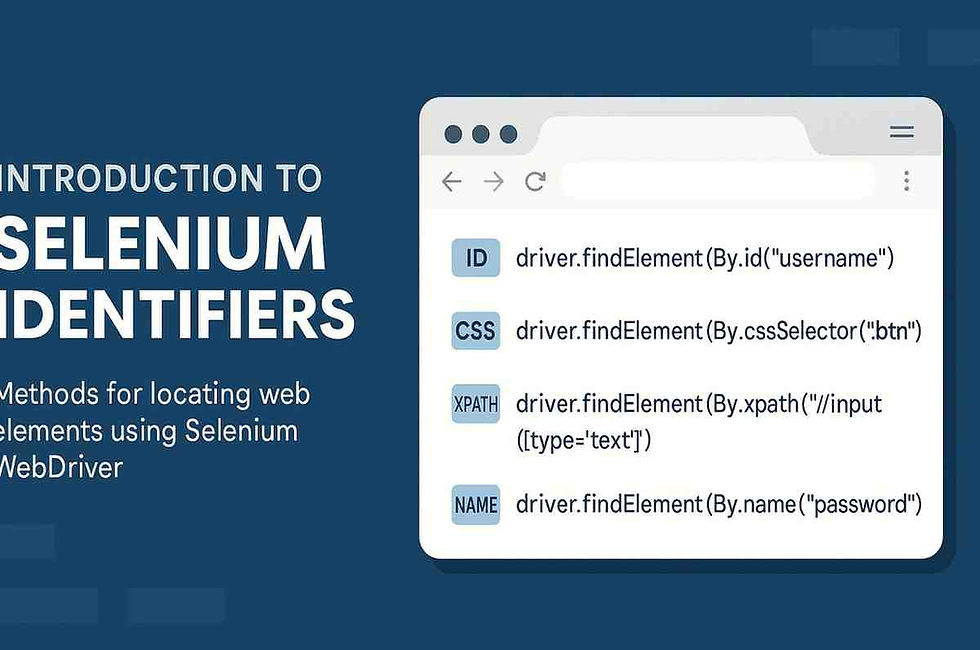
Types of Selenium Identifiers
Selenium WebDriver supports several types of locators, each with its own strengths and ideal use cases. Let's dive into each type to understand how they work and when to use them.
1. ID Locator
The ID locator is the most preferred and efficient way to find elements on a web page. It uses the 'id' attribute of HTML elements, which should be unique within a page.
Syntax:
WebElement element = driver.findElement(By.id("elementId"));
HTML Example:
<input id="username" type="text" name="username">
Advantages:
Fastest locator strategy, as browsers have built-in optimization for ID lookup
Simple and straightforward to use
Less prone to breaking when the page structure changes
Disadvantages:
Not all elements have IDs
In some applications, IDs might be dynamically generated, making them unreliable for testing.
Best For: Elements with stable, unique IDs that don't change between page refreshes or application builds.
2. Name Locator
The name locator finds elements using the 'name' attribute commonly found in form elements.
Syntax:
WebElement element = driver.findElement(By.name("elementName"));
HTML Example:
<input name="password" type="password">
Advantages:
Good for form elements, which typically have name attributes
Reasonably fast performance
Often more stable than some other locators
Disadvantages:
Multiple elements can share the same name
Not all elements have a name attribute
Best For: Form elements like input fields, checkboxes, and radio buttons.
3. Class Name Locator
This locator finds elements based on their CSS class attribute.
Syntax:
WebElement element = driver.findElement(By.className("classname"));
HTML Example:
<button class="btn primary-btn submit-btn">Submit</button>
Advantages:
Useful when elements share a common function or style
Can help identify elements that belong to a specific category
Disadvantages:
Often returns multiple elements, as classes are frequently reused
Cannot use compound classes (spaces in class names)
Best For: Finding a group of similar elements or when other locators aren't available.
4. Tag Name Locator
The tag name locator finds elements by their HTML tag.
Syntax:
WebElement element = driver.findElement(By.tagName("tagname"));
HTML Example:
<h1>Welcome to our website</h1>
Advantages:
Simple to use
Helpful for finding all elements of a specific type
Disadvantages:
Very rarely unique, almost always returns multiple elements
Too generic for precise element location
Best For: Scenarios where you need to find all elements of a particular type, like all links or all buttons, typically used with findElements() rather than findElement().
5. Link Text and Partial Link Text Locators
These locators are specifically for hyperlink elements (a tags).
Syntax:
// Full link text
WebElement element = driver.findElement(By.linkText("Click here"));
// Partial link text
WebElement element = driver.findElement(By.partialLinkText("Click"));
HTML Example:
<a href="https://www.example.com">Click here to visit our website</a>
Advantages:
Intuitive way to find links
Works well for user-focused tests that simulate clicking on visible text
Disadvantages:
Text might change with language localization
Not suitable for non-link elements
Link text might not be unique on a page
Best For: Navigation tests that involve clicking on links with distinct text.
Advanced Selenium Identifiers
While the basic locators cover many scenarios, two advanced locator strategies provide powerful capabilities for finding complex or dynamically generated elements.
1. CSS Selector
CSS Selectors offer a versatile way to locate elements using the same syntax used in CSS stylesheets.
Syntax:
WebElement element = driver.findElement(By.cssSelector("selector"));
Common CSS Selector Patterns:
Pattern | Description | Example |
Selects an element by ID | ||
.class | Selects elements by class | .submit-button |
element | Selects elements by tag name | input |
[attribute=value] | Selects elements with a specific attribute value | [name="password"] |
parent > child | Selects direct child elements | form > input |
ancestor descendant | Selects descendant elements | div span |
:nth-child(n) | Selects the nth child element | li:nth-child(2) |
Advantages:
Faster than XPath in most browsers
More concise syntax
Powerful selection capabilities
Good browser compatibility
Disadvantages:
Cannot traverse up the DOM (select parent from child)
Less readable for complex selections compared to XPath
Best For: General-purpose element location when IDs or names aren't available.
2. XPath
XPath is the most powerful and flexible locator strategy, allowing you to navigate through the entire DOM structure.
Syntax:
WebElement element = driver.findElement(By.xpath("xpathExpression"));
Common XPath Patterns:
Pattern | Description | Example |
//tag | Selects all elements with the given tag | //input |
//tag[@attribute='value'] | Selects elements with a specific attribute value | //input[@id='username'] |
//tag[contains(@attribute,'partial')] | Selects elements containing text in the attribute | //div[contains(@class,'menu')] |
//tag[text()='text'] | Selects elements with exact text | //button[text()='Submit'] |
//tag/parent::* | Selects the parent of an element | //input[@id='email']/parent::div |
//tag/following-sibling::* | Select siblings after the current element | //label/following-sibling::input |
//tag/preceding-sibling::* | Select siblings before the current element | //input/preceding-sibling::label |
Advantages:
Most powerful and flexible locator
Can traverse up, down, and across the DOM
Can locate elements using text content
Can find elements without any attributes
Disadvantages:
Slower performance compared to other locators
More complex syntax
Can break more easily with page structure changes
May have slight browser compatibility differences
Best For: Complex scenarios where other locators aren't sufficient, or when you need to locate elements based on their relationship to other elements.
Selecting the Right Selenium Identifier
Choosing the appropriate locator strategy is crucial for creating robust, maintainable test scripts. Here's a decision flow to help you select the most effective identifier:
ID: If the element has a stable, unique ID attribute, use it
Name: If no suitable ID is available, check for a unique name attribute
CSS Selector: For most other scenarios, use CSS selectors for their balance of power and performance
XPath: Reserved for complex scenarios where other locators aren't sufficient
When evaluating potential locators, consider these factors:
Uniqueness: Will this locator identify exactly one element?
Stability: Will this locator continue to work when the page is updated?
Readability: Is the locator easy for other team members to understand?
Maintenance: How likely is it that this locator will need to be updated?
Best Practices for Using Selenium Identifiers
To create robust, maintainable test automation, follow these best practices when working with Selenium identifiers:
1. Prioritize Stable Attributes
Some attributes are less likely to change during development than others:
Good choices: id, name, stable custom data attributes (data-*)
Avoid when possible: Class names (especially when used for styling), relative positions, and indexes
2. Use Explicit Waits
Don't rely on fixed sleep times; instead, wait for specific elements to appear:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.presenceOfElementLocated(By.id("dynamicElement")));
3. Create a Locator Repository
Implement the Page Object Model pattern to store locators separately from test logic:
public class LoginPage {
private By usernameField = By.id("username");
private By passwordField = By.id("password");
private By loginButton = By.cssSelector(".login-btn");
// Methods that use these locators
}
4. Prefer Relative Locators Over Absolute Paths
When using XPath, prefer relative paths that won't break when the page structure changes:
Avoid: /html/body/div[2]/form/input[3]
Prefer: //form[@id='login-form']//input[@name='password']
5. Combine Multiple Attributes for Greater Specificity
When elements lack unique identifiers, combine attributes for more specific selection:
// CSS Selector example
driver.findElement(By.cssSelector("input[type='submit'][value='Login']"));
// XPath example
driver.findElement(By.xpath("//button[@type='submit' and contains(@class,'primary')]"));
Conclusion
Mastering Selenium identifiers is essential for creating effective, maintainable test automation. While it might be tempting to use whichever locator works first, taking the time to select the optimal identifier strategy pays dividends in test stability and reduced maintenance.
Remember that each type of locator has its strengths and ideal use cases. By understanding these differences and following best practices, you can create test scripts that remain robust even as the application under test evolves.
As you gain experience, you'll develop an intuition for which locator will work best in different situations. Keep experimenting with different approaches, and don't be afraid to refactor your locators as you learn more efficient techniques.
Key Takeaways
ID is the fastest and most reliable locator when available
CSS Selectors offer the best balance of speed and flexibility for most scenarios
XPath is the most powerful, but should be used judiciously due to performance considerations
Create a locator strategy that prioritizes stable, unique attributes
Implement Page Object Model to centralize and maintain locators
Use explicit waits rather than fixed sleeps to handle dynamic elements
Avoid absolute paths and position-based locators that break easily
Combine multiple attributes when no single unique identifier exists
Test your locators across different browsers to ensure compatibility
Regular review and refactoring of locators helps maintain test stability
FAQ About Selenium Identifiers
What is the fastest Selenium locator?
The ID locator is the fastest Selenium locator because browsers have built-in optimization for finding elements by their ID attribute. When elements have unique, stable IDs, this should be your first choice for performance reasons.
How do I find elements that have dynamically generated IDs?
For elements with dynamically generated IDs that change on each page load, look for patterns in the ID (like a prefix that remains constant) and use contains() in XPath or CSS. Alternatively, locate the element using nearby stable elements, its content, or other attributes that don't change.
Which is better: XPath or CSS Selector?
CSS Selectors are generally faster and more browser-compatible than XPath, making them preferable for most scenarios. However, XPath offers unique capabilities like locating elements by text content and traversing upward in the DOM (finding parent elements), which CSS cannot do. Choose based on your specific requirements.
How can I locate elements inside an iframe?
To interact with elements inside an iframe, you must first switch to the iframe context using switchTo().frame() method. You can switch to the frame using its ID, name, index, or by finding the frame WebElement first:
// By ID
driver.switchTo().frame("frameId");
// By WebElement
WebElement frameElement = driver.findElement(By.cssSelector(".frame-class"));
driver.switchTo().frame(frameElement);
What should I do when elements have no unique attributes?
When elements lack unique attributes, consider these approaches:
Use relative locators based on parent or nearby elements
Combine multiple attributes to create uniqueness
Use text content (with XPath)
Talk to developers about adding test-specific attributes like data-testid
Use more complex CSS or XPath that considers the element's position in the DOM structure
How do I handle elements that change their attributes?
For elements with changing attributes, focus on attributes that remain stable or use more flexible locator strategies:
Use contains() to match partial attribute values that don't change
Locate the element based on its relationship to stable elements nearby
Use multiple fallback locators and try them in sequence
Ask developers to add stable attributes specifically for testing purposes
Comments