Mastering Actions App in Cypress: Guide
- Gunashree RS
- Sep 21, 2024
- 7 min read
Updated: Sep 22, 2024
When testing applications with Cypress, one of the most debated topics in the test automation community is whether to use Page Objects or App Actions. Page Object Model (POM) has been a staple in automation testing frameworks for years, offering a clean way to manage UI interactions. However, with the advent of App Actions, Cypress has empowered testers to optimize workflows by interacting directly with the application state, making some believe that Page Objects is an outdated approach.
In this comprehensive guide, we will dive deep into App Actions in Cypress, compare them with Page Objects, and explore how using App Actions can unlock incredible speed and efficiency in testing. Whether you are new to automation testing or a seasoned professional, understanding the nuances between these two approaches can revolutionize your testing strategy.
What is an Actions App in Cypress?
In the context of Cypress, App Actions refer to a methodology that bypasses direct user interface interactions (like clicking buttons or typing in fields) and instead manipulates the application’s state directly. This is achieved by calling the same internal functions that your app’s front end uses. Cypress operates inside the browser, allowing access to app states, variables, and functions, making it possible to trigger behaviors without having to simulate user interaction.
By leveraging App Actions, testers can avoid unnecessary steps in their tests and jump directly to the application state they want to test, ultimately reducing test execution time.
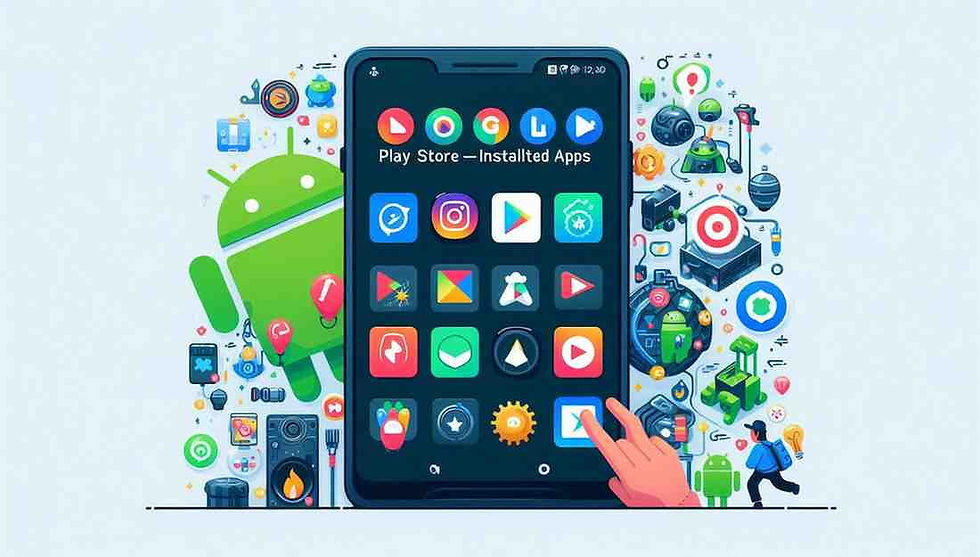
Understanding the Page Object Model in Testing
The Page Object Model (POM) is a widely adopted design pattern in automation testing. It helps manage interactions with web elements by abstracting them into separate files or classes, often referred to as "Page Objects." Each Page Object represents a different page or section of your application, with methods to interact with buttons, fields, and other UI elements.
For example, instead of writing Cypress commands to interact with a login form directly in your test file, you would create a Login page object that contains reusable methods such as openLoginForm() or submitLoginForm(). These methods can be reused across multiple tests, making the code cleaner, more maintainable, and less prone to duplication.
While this pattern has many benefits, it also comes with some limitations in Cypress due to its UI interaction-based design.
App Actions vs. Page Objects: Key Differences
Page Objects
Interaction Focus: Uses Cypress commands like cy.get() and cy.click() to interact with the app’s UI.
Code Reusability: Abstracts UI elements into reusable methods.
Maintenance: Easy to maintain if UI elements change, as updates only need to be made in the Page Object files.
Execution Time: Relatively slower due to the need to simulate real user actions.
App Actions
State Manipulation: Skips UI interaction and directly manipulates the app’s internal state or call functions.
Speed: Faster than Page Objects because there’s no need to interact with the UI unless absolutely necessary.
Reduced Flakiness: Minimizes the chances of issues like missing clicks or slow UI responses affecting tests.
Complexity: Requires more insight into the app’s internal workings, such as knowing which states or functions to call.
Advantages of Using App Actions
Speed and Efficiency: Since App Actions bypass the UI and manipulate the application state directly, tests run faster. This is particularly beneficial when running large test suites or working in a CI/CD pipeline.
Direct Control Over State: App Actions allow testers to set the application state programmatically, avoiding unnecessary steps like navigating through the UI to reach a specific view.
Reduced UI Flakiness: Traditional tests that interact with the UI can be flaky due to timing issues or slow rendering. App Actions reduce these risks by skipping UI interactions altogether.
Simplified Testing for Complex Workflows: For complex apps with multiple layers of user interactions, reaching a specific state via the UI can require many steps. App Actions can simplify these workflows by setting the application to the desired state instantly.
Easier Visual Testing: App Actions are particularly useful when setting up your app for visual testing, where the focus is not on UI behavior but on the appearance of the elements.
Creating App Actions in Cypress
Here’s a step-by-step guide to implementing App Actions in Cypress.
Step 1: Expose Your App to Cypress
To interact with your app's internal state, you need to expose the app's instance to Cypress. In a Vue.js app, for example, you can modify your index.js file to add the following line:
javascript
const app = new Vue({
// app configuration
}).$mount('#app');
window.app = app; // Expose the app to Cypress
This line makes the app instance accessible via the window object, allowing Cypress to interact with it.
Step 2: Create Cypress Custom Commands
You can now create custom commands in Cypress to manipulate the app’s state directly. For example, if your app has a login modal that is toggled by a showLoginModule property, you can write the following command:
javascript
Cypress.Commands.add('openLoginModal', () => {
cy.window().then(({ app }) => {
app.showLoginModule = true; // Set the login modal to open
});
});
This command allows you to open the login modal without clicking any buttons. In your tests, you would use:
javascript
cy.openLoginModal();
Combining Page Objects with App Actions
The beauty of Cypress is its flexibility. You don't have to choose between Page Objects and App Actions—you can combine both depending on your test case. For example:
Use Page Objects for UI interactions that must simulate real user behavior, such as form submissions, navigation, or accessibility testing.
Use App Actions to bypass repetitive or complex UI interactions and directly set the app’s state when you're not testing those specific interactions.
This combination ensures that your tests remain fast, efficient, and easy to maintain.
When to Use App Actions
App Actions are best suited for:
Visual Testing: When you only need the app to be in a specific state for visual testing, App Actions allow you to skip unnecessary UI interactions.
Complex State Management: For apps with complex workflows (e.g., multi-step forms or dashboard settings), App Actions allow you to set the desired state directly without going through every interaction.
Reducing Test Execution Time: If you're running thousands of tests, every second counts. App Actions help reduce test execution time by skipping steps that aren't directly related to the test.
UI Flakiness: When dealing with flaky UI tests that fail due to timing or rendering issues, App Actions can eliminate the need to interact with the UI, making your tests more reliable.
Common Use Cases for App Actions
Form Validation Tests: Instead of filling out a multi-step form each time, use App Actions to jump to the last step where validation occurs.
Role-Based Access Control (RBAC) Testing: Directly set user roles or permissions through App Actions rather than going through the UI.
Testing App States: For features like dark mode, multi-language support, or specific user settings, App Actions allow you to toggle these states programmatically without navigating through multiple menus.
Challenges and Considerations
While App Actions offer numerous benefits, there are some challenges and considerations to keep in mind:
Access to App Internals: You need to have access to the app’s internal functions and state, which may not always be possible depending on the framework or architecture of the application.
Non-Realistic User Scenarios: By skipping UI interactions, you may miss bugs related to the UI, such as buttons being unclickable or form fields not being interactive.
Complexity for Beginners: For testers who are less familiar with the inner workings of the application or the framework (e.g., Vue.js or React), setting up App Actions may be more complex than using Page Objects.
Conclusion
In the world of automation testing, App Actions have emerged as a powerful alternative to the traditional Page Object Model, particularly for testers working with Cypress. By allowing direct manipulation of the app’s state, App Actions help reduce test execution time, eliminate flakiness, and streamline complex workflows. However, both approaches have their strengths, and in many cases, a hybrid solution combining both App Actions and Page Objects is ideal.
Cypress's flexibility allows testers to harness the power of both methodologies, ensuring that their test suites remain fast, reliable, and maintainable. Whether you’re performing UI interactions or visual tests, understanding when to use App Actions can give your testing strategy a significant boost.
Key Takeaways
App Actions allow you to interact directly with an app’s internal state, bypassing the need for UI interaction.
They are faster than traditional Page Objects, making them ideal for large test suites and CI/CD pipelines.
Page Objects are still useful for abstracting common UI interactions and ensuring code reusability.
A hybrid approach, combining both App Actions and Page Objects, offers the best of both worlds.
App Actions help eliminate flakiness caused by UI timing issues and can simplify tests for complex workflows.
Frequently Asked Questions
1. What are App Actions in Cypress?
App Actions in Cypress allow you to interact directly with an application’s internal state or functions, bypassing the UI. This speeds up tests and reduces the risk of UI-related issues.
2. Should I stop using Page Objects?
Not necessarily. Page Objects are still useful for organizing and abstracting UI interactions, especially when testing specific workflows that require real user actions. App Actions and Page Objects can be combined.
3. When should I use App Actions?
App Actions are ideal when you need to set the app to a specific state quickly, such as for visual testing, reducing test execution time, or bypassing complex workflows.
4. How do I expose my app to Cypress for App Actions?
You can expose your app by attaching the app instance to the window object, allowing Cypress to access the app’s state and internal functions.
5. Can I use both Page Objects and App Actions together?
Yes! You can combine both approaches in your test suite, using Page Objects for UI interactions and App Actions to directly manipulate the app’s state where appropriate.
6. Do App Actions work with all frameworks?
Yes, App Actions work with most modern JavaScript frameworks like React, Vue, and Angular, as long as you can expose the app instance to Cypress.
Comments