Integration vs Unit Test: Guide for Developers
- Gunashree RS
- 2 hours ago
- 7 min read
Introduction to Software Testing Frameworks
Software testing forms the backbone of quality assurance in development cycles. Among the myriad of testing methodologies available, unit testing and integration testing are two fundamental pillars that every developer must thoroughly understand. While both aim to identify bugs and ensure software reliability, they serve distinctly different purposes within the testing pyramid.
Modern development practices like Test-Driven Development (TDD) and Continuous Integration/Continuous Deployment (CI/CD) rely heavily on both testing approaches to deliver robust software solutions. This comprehensive guide will help you navigate the sometimes confusing landscape of integration vs unit test methodologies, providing clear guidance on when and how to implement each approach effectively.
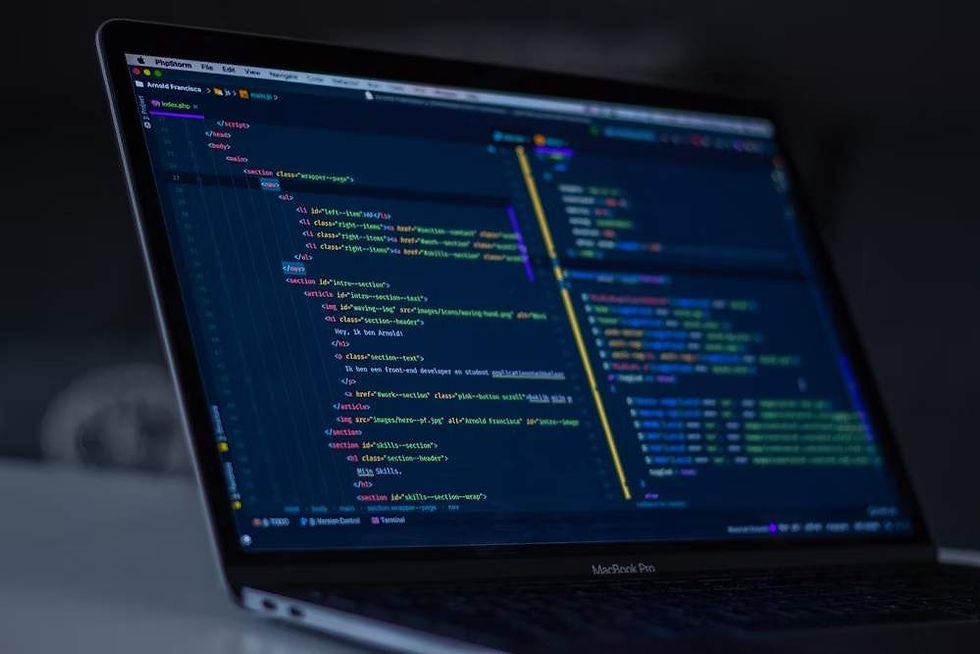
What Are Unit Tests?
Unit tests represent the foundation of the testing pyramid. These focused tests examine individual components of your code in isolation, typically at the function, method, or class level.
Characteristics of Unit Tests:
Scope: Tests the smallest testable parts of an application independently
Isolation: Mocks or stubs external dependencies to ensure true isolation
Speed: Executes very quickly (milliseconds per test)
Complexity: Generally simple to write and maintain
Quantity: Usually, the most numerous tests in a project
Unit tests verify that specific functions produce expected outputs when given certain inputs. They don't validate how components interact with each other or with external systems like databases or APIs.
Example of a Unit Test:
javascript
// Function to test
function add(a, b) {
return a + b;
}
// Unit test for the add function
test('correctly adds two numbers', () => {
expect(add(2, 3)).toBe(5);
expect(add(-1, 1)).toBe(0);
expect(add(0, 0)).toBe(0);
});
This simple test verifies that our add function behaves correctly in isolation, without considering how this function might be used within the broader application context.
What Are Integration Tests?
While unit tests focus on individual components, integration tests examine how these components work together. They verify that different parts of your application cooperate as expected.
Characteristics of Integration Tests:
Scope: Tests interactions between multiple components or systems
Dependencies: Often uses actual dependencies rather than mocks
Speed: Slower than unit tests (seconds or minutes per test)
Complexity: More complex to set up and maintain
Quantity: Fewer than unit tests, but more comprehensive
Integration tests help identify issues that might not be apparent when testing components in isolation, such as improper interface implementations, configuration errors, or performance issues.
Types of Integration Testing:
Top-down integration testing: Testing begins with top-level modules, gradually working down to lower-level modules
Bottom-up integration testing: Testing starts with lower-level modules and progressively integrates higher-level modules
Sandwich/Hybrid integration testing: Combines both top-down and bottom-up approaches
Big bang integration testing: All modules are integrated simultaneously and tested as a unit
Example of an Integration Test:
javascript
// Integration test for user registration flow
test('user registration process', async () => {
// Create a test database connection
const db = connectToTestDatabase();
// Create a user service with real database dependency
const userService = new UserService(db);
const authService = new AuthenticationService(db);
// Test the integration between these services
const newUser = await userService.registerUser({
username: 'testuser',
email: 'test@example.com',
password: 'securepassword123'
});
const authResult = await authService.loginUser({
email: 'test@example.com',
password: 'securepassword123'
});
expect(authResult.success).toBe(true);
expect(authResult.user.id).toBe(newUser.id);
// Clean up
await db.disconnect();
});
This integration test verifies that the user registration and authentication processes work correctly together, including actual database interactions.
Key Differences: Integration vs Unit Test
Understanding the fundamental differences between these testing methodologies helps developers implement the right strategy for their projects.
Aspect | Unit Testing | Integration Testing |
Purpose | Verify that individual components work correctly | Verify that the components work together correctly |
Scope | Single function, method, or class | Multiple components or systems |
Dependencies | Mocked or stubbed | Real or partially mocked |
Execution Speed | Very fast | Slower |
Isolation Level | High | Low to moderate |
Maintenance Cost | Lower | Higher |
Test Setup Complexity | Simple | More complex |
Test Quantity | Many tests | Fewer tests |
Timing in Development | Early (can be written before implementation) | Later (requires implemented components) |
Bug Detection | Logic errors within components | Interface and integration issues |
When to Use Unit Tests
Unit tests are particularly valuable in the following scenarios:
When implementing complex algorithms: Unit tests help verify that the algorithmic logic functions correctly across various inputs and edge cases.
During early development stages: Even before all components are ready, developers can write unit tests to guide implementation.
For critical business logic: Core functionality that must be reliable benefits from thorough unit testing.
When refactoring code: Unit tests provide confidence that changes haven't broken existing functionality.
For code with few external dependencies: Pure functions and self-contained modules are ideal candidates for unit testing.
Unit tests shine when you need rapid feedback during development and want to pinpoint exactly where issues occur.
When to Use Integration Tests
Integration tests become essential in these scenarios:
Testing service interactions: When multiple services must work together correctly, integration tests verify their collaboration.
Data layer operations: Testing database operations, ORM functionality, and data persistence requires integration testing.
API endpoint validation: Ensuring that API endpoints correctly handle requests and responses is a primary integration testing scenario.
Third-party service integrations: When your application interacts with external services, integration tests verify these interactions work properly.
User workflows: Complex user journeys that span multiple components benefit from integration testing.
Integration tests provide confidence that your system works as a cohesive whole, catching issues that unit tests might miss.
Best Practices for Unit Testing
To get the most from your unit testing efforts:
Keep tests truly isolated: Avoid dependencies on external systems, database connections, or file I/O.
Follow the AAA pattern: Arrange (set up test conditions), Act (execute the function being tested), Assert (verify the result).
Test one concept per test: Each test should verify a single behavior or outcome.
Use descriptive test names: Name tests based on what they're verifying, like "shouldReturnFalseWhenInputIsNegative."
Aim for high coverage: While 100% code coverage isn't always necessary, critical paths should be thoroughly tested.
Test edge cases: Include tests for boundary conditions, empty inputs, extremely large values, etc.
Keep tests fast: Unit tests should execute in milliseconds to maintain rapid feedback during development.
Best Practices for Integration Testing
For effective integration testing:
Create a dedicated test environment: Integration tests should run in an environment similar to production but isolated from it.
Use test databases: Avoid testing against production databases; use dedicated test databases that can be reset between test runs.
Manage test data carefully: Create, use, and clean up test data systematically to prevent test interference.
Consider test order: Unlike unit tests, integration tests sometimes depend on execution order.
Balance mocking: Mock external dependencies that are difficult to include in tests (like payment gateways), but use real implementations for components you're directly testing.
Focus on critical paths: Prioritize testing the most important user workflows and system interactions.
Automate cleanup: Ensure tests clean up after themselves to maintain test environment integrity.
Building a Balanced Testing Strategy
The most effective testing strategies combine both unit and integration tests in appropriate proportions. This is often visualized as a testing pyramid:
Base layer: Numerous unit tests covering individual components
Middle layer: Integration tests verifying component interactions
Top layer: End-to-end tests validating complete workflows
Industry best practices suggest aiming for approximately:
70% unit tests
20% integration tests
10% end-to-end or UI tests
This distribution balances thoroughness with maintenance costs and execution speed.
Conclusion
Both integration and unit tests play vital roles in ensuring software quality, but they serve different purposes. Unit tests provide rapid feedback on individual components, while integration tests verify that these components work together correctly. By understanding the strengths and appropriate applications of each testing approach, developers can build more robust, reliable software systems.
The decision between integration vs unit test methodologies isn't an either/or choice—both are essential parts of a comprehensive testing strategy. The key is knowing when and how to apply each approach effectively throughout the development lifecycle.
Key Takeaways
Unit tests focus on individual components in isolation, while integration tests verify that components work together correctly.
Unit tests are faster, simpler, and more numerous than integration tests.
A balanced testing strategy includes both unit and integration tests in appropriate proportions.
Unit tests excel at verifying logical correctness, while integration tests catch interface and configuration issues.
The testing pyramid suggests 70% unit tests, 20% integration tests, and 10% end-to-end tests.
Both testing approaches are complementary rather than competitive.
Unit tests provide rapid feedback during development, while integration tests offer confidence in system cohesion.
Modern CI/CD pipelines benefit from both unit and integration tests at different stages.
Frequently Asked Questions (FAQ)
Can unit tests replace integration tests?
No, unit tests and integration tests serve different purposes. Unit tests verify that individual components work correctly in isolation, while integration tests confirm that these components work together as expected. Both types of tests are necessary for comprehensive quality assurance.
How long should unit tests and integration tests take to run?
Unit tests should be very fast, typically executing in milliseconds. A comprehensive suite of thousands of unit tests should run in just a few minutes. Integration tests are naturally slower, often taking seconds or minutes per test, due to their wider scope and real dependencies.
Should I write unit tests or integration tests first?
Most development methodologies recommend writing unit tests first, as they're faster to implement and provide immediate feedback during development. Integration tests typically follow once individual components are implemented and unit-tested.
What is the right ratio of unit tests to integration tests?
The commonly accepted testing pyramid suggests approximately 70% unit tests, 20% integration tests, and 10% end-to-end or UI tests. However, this ratio may vary based on project requirements and complexity.
How do I handle external dependencies in unit tests?
In unit testing, external dependencies should typically be mocked or stubbed to ensure true isolation. This approach prevents external systems from affecting test results and keeps tests fast and reliable.
Do microservices require more integration testing?
Yes, microservice architectures generally require more extensive integration testing due to their distributed nature and service-to-service communications. Integration tests become crucial for verifying that independently deployed services work together correctly.
How can I make integration tests more reliable?
To improve integration test reliability, use dedicated test environments, manage test data carefully, implement proper cleanup after tests, handle asynchronous operations correctly, and consider using containers or similar technologies to ensure consistent test environments.
What tools are popular for unit and integration testing?
For unit testing: JUnit, NUnit, PyTest, Jest, and Mocha are widely used, depending on the programming language. For integration testing: TestNG, Selenium, Cypress, Postman, and RestAssured are popular choices. CI/CD tools like Jenkins, GitHub Actions, and CircleCI are commonly used to automate both types of tests.
Comments