VSCode Python Formatter: Guide to Streamlined Code Formatting
- Gunashree RS
- Sep 13, 2024
- 6 min read
Maintaining clean, readable, and consistently styled Python code is critical for any development project. It makes code reviews simpler, prevents unnecessary errors, and enhances collaboration among developers. Visual Studio Code (VSCode), one of the most popular code editors today, supports several Python formatters that ensure your code follows standardized styles.
In this comprehensive guide, we will explore the top Python formatters available in VSCode, how to use them effectively, and the benefits of integrating these tools into your development workflow. Whether you are a beginner or an experienced developer, this guide will help you select and use the best Python formatter for your project.
Why Use a Python Formatter in VSCode?
Code formatting tools are essential for maintaining consistency across large codebases, especially in teams. Without a Python code formatter, developers may end up wasting time on stylistic nitpicks during code reviews or introducing errors due to inconsistent code styles. Code formatters automate the task of aligning the code to a particular style guide, such as PEP 8, the official Python style guide.
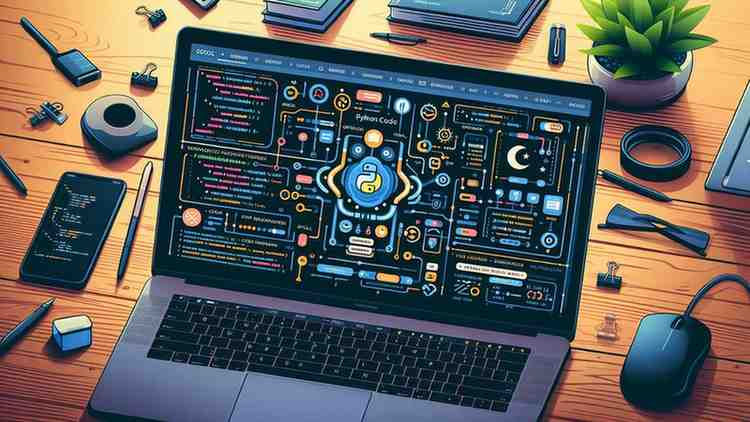
Here are the main reasons why using a Python formatter in VSCode is crucial:
Consistency: All contributors can follow the same style rules without manual enforcement.
Readability: A well-formatted code is easier to understand, which reduces the cognitive load for developers.
Faster code reviews: Automating code formatting minimizes back-and-forth discussions about style in code reviews.
Error prevention: Clean and organized code is less likely to contain bugs.
Time efficiency: Saves time by automating the formatting process instead of relying on manual changes.
How to Install Python Formatters in VSCode
Installing and configuring Python formatters in VSCode is simple and straightforward. Follow these steps to get started:
Install the Python extensionFirst, ensure you have the official Python extension installed in VSCode. This extension provides essential features like IntelliSense, linting, debugging, and support for Python formatters.To install:
Open the Extensions view (Ctrl + Shift + X).
Search for "Python" and select the extension from Microsoft.
Click "Install."
Select a FormatterVSCode supports several Python formatters out of the box, including Black, isort, autopep8, and YAPF. Choose the one that best fits your needs.Once you have the Python extension installed, you'll be prompted to select a formatter the first time you attempt to format a Python file. You can also configure it later in your settings.json file.
Set the Default FormatterTo set the default formatter:
Open the command palette (Ctrl + Shift + P) and type Preferences: Open Settings (JSON).
Add the following lines to configure your preferred formatter:
json
{
"python.formatting.provider": "black",
"editor.formatOnSave": true
}
Replace "black" with "autopep8", "isort", or "yapf" if you choose a different formatter.
Format On SaveFor a seamless experience, enable automatic formatting on save. This will ensure that every time you save a Python file, it gets formatted according to your chosen style.Add the following line to your settings.json file:
json
"editor.formatOnSave": true
Top Python Formatters for VSCode
1. Black
Black has become one of the most popular Python formatters, favored for its strictness, speed, and determinism. It offers an "opinionated" approach, meaning it follows a very strict set of formatting rules, leaving no room for debates on style choices. Black ensures that the code looks consistent across the board, and it is especially popular in large projects with multiple contributors.
Key Features of Black:
Uncompromising: Black doesn't offer options to configure style—this guarantees consistency.
Deterministic: Running Black on the same code multiple times yields the same results.
Fast: It's optimized for performance, even on large codebases.
to Use Black in VSCode:
Install Black via pip:
bash
pip install black
Configure VSCode to use Black:
json
{
"python.formatting.provider": "black",
"editor.formatOnSave": true
}
Run Black manually:
bash
black your_file.py
2. isort
While Black focuses on overall code formatting, isort specializes in sorting Python imports according to the PEP 8 style guide. This tool is perfect for organizing import statements automatically, ensuring they're grouped and ordered logically.
Key Features of isort:
PEP 8 Compliance: Ensures that imports follow the recommended order—standard libraries, third-party libraries, and local imports.
Customizable: You can configure isort to follow specific rules if needed.
Efficient: Quickly sorts imports in both small scripts and large projects.
How to Use isort in VSCode:
Install isort via pip:
bash
pip install isort
Configure VSCode to use isort:
json
{
"python.sortImports.args": ["--profile", "black"],
"editor.formatOnSave": true
}
Run isort manually:
bash
isort your_file.py
3. autopep8
autopep8 is an auto-formatter that adjusts Python code to conform to the PEP 8 style guide. Unlike Black, it allows for some flexibility and customizability. It’s a great tool for those who want to follow PEP 8 while still maintaining some level of control over how their code is formatted.
Key Features of autopep8:
PEP 8 Enforcement: autopep8 focuses specifically on ensuring PEP 8 compliance.
Configurable: Developers can tweak how autopep8 formats code.
Widespread Usage: Widely adopted in many Python projects.
How to Use autopep8 in VSCode:
Install autopep8 via pip:
bash
pip install autopep8
Configure VSCode to use autopep8:
json
{
"python.formatting.provider": "autopep8",
"editor.formatOnSave": true
}
Run autopep8 manually:
bash
autopep8 --in-place your_file.py
4. YAPF
YAPF (Yet Another Python Formatter) focuses on making Python code not only PEP 8 compliant but also visually appealing. It uses a more aggressive formatting algorithm, making decisions based on the structure of the code, even if it doesn’t strictly violate any style rules.
Key Features of YAPF:
Code Prettification: Not just PEP 8 compliance—YAPF aims to make your code look its best.
Highly Customizable: YAPF offers a range of settings to tweak how your code is formatted.
Flexible: Unlike Black, YAPF gives developers more control over the final look of their code.
How to Use YAPF in VSCode:
Install YAPF via pip:
bash
pip install yapf
Configure VSCode to use YAPF:
json
{
"python.formatting.provider": "yapf",
"editor.formatOnSave": true
}
Run YAPF manually:
bash
yapf -i your_file.py
Using Python Formatters with DeepSource in VSCode
DeepSource integrates seamlessly with VSCode to automate code quality checks and formatting. By using Transformers in DeepSource, you can configure your project to automatically run code formatters like Black, isort, and YAPF.
Steps to Configure DeepSource for Python Formatters:
Create a .deepsource.toml file in your project root.
Add Transformers for Black or other formatters:
toml
[[transformers]]
name = "black"
enabled = true
[[transformers]]
name = "isort"
enabled = true
DeepSource will automatically format your code when running static analysis, ensuring both style consistency and high code quality.
Conclusion
Choosing the right Python formatter for VSCode depends on your needs and preferences. Whether you want strict, opinionated formatting with Black or prefer the flexibility of autopep8 or YAPF, VSCode makes it easy to integrate these tools into your workflow. By automating code formatting, you ensure that your Python code stays clean, readable, and consistent across your entire codebase.
Key Takeaways:
Consistency: Code formatters ensure uniform style across teams and projects.
Efficiency: Tools like Black and isort save time by automating code formatting.
Flexibility: Some formatters like autopep8 and YAPF allow more customization.
VSCode Integration: Python formatters can be easily configured in VSCode, with options for automatic formatting on save.
DeepSource Compatibility: Python formatters can be integrated into static analysis workflows using DeepSource.
FAQs
1. How do I change the default Python formatter in VSCode?
You can change the default formatter by modifying the settings.json file. Use the command palette (Ctrl + Shift + P), search for "Preferences: Open Settings (JSON)," and add "python.formatting.provider": "formatter_name" where "formatter_name" is the formatter you wish to use.
2. Can I use multiple Python formatters in VSCode?
Yes, but it is generally not recommended as it can lead to conflicting formatting rules. However, tools like isort can complement Black, as one handles imports, and the other handles general code formatting.
3. Is Black faster than autopep8?
Yes, Black is typically faster than autopep8 and YAPF, especially for large projects, due to its optimized algorithms.
4. What is the most popular Python formatter in VSCode?
Black is currently the most popular Python formatter due to its simplicity, speed, and wide adoption.
5. How do I enable format on save in VSCode?
To enable format on save, open your settings.json file and add the line "editor.formatOnSave": true. This will format your Python code each time you save the file.
6. Is YAPF stricter than Black?
YAPF offers more flexibility compared to Black, which is highly opinionated. YAPF provides options to tweak how your code is formatted, while Black enforces a consistent style without customization.
Yorumlar