The demand for mobile applications has never been higher. With over 3 billion active Android devices worldwide, Android apps are a key part of the global tech ecosystem. Whether you're a seasoned developer or a complete beginner, learning how to write an Android app can be a rewarding skill. From games and utilities to social media apps, the possibilities for what you can build are endless.
This detailed guide will take you through each step of creating your first Android app—from setting up your development environment to deploying your app on the Google Play Store. You don't need to be an expert to get started; with the right tools and a bit of patience, you'll be well on your way to publishing your first Android app.
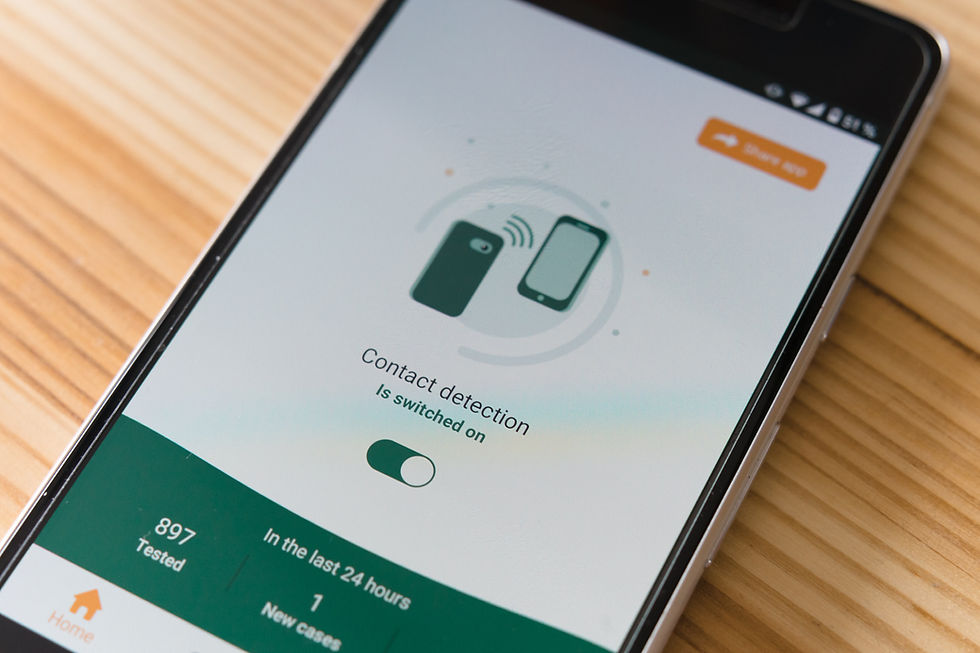
1. Why Learn to Write an Android App?
Android apps power more than just smartphones. They run on smart TVs, watches, tablets, and even cars. Android's open ecosystem and widespread adoption make it an attractive platform for developers. Learning to build Android apps gives you the potential to:
Enter a growing job market: Android developers are in demand.
Build your own business: Launch your apps and monetize them on the Google Play Store.
Solve real-world problems: Create apps that impact millions of users.
2. Tools You Need to Get Started
Android Studio
The official Integrated Development Environment (IDE) for Android development is Android Studio. It’s a complete toolkit that allows you to design, code, test, and debug Android apps. Android Studio supports both Java and Kotlin (Google’s recommended language for Android development).
Java or Kotlin: Programming Languages
Java has long been the standard language for Android development, while Kotlin has recently been adopted as the preferred language by Google. Both are fully supported in Android Studio, but Kotlin offers more modern features like null safety and less boilerplate code, making it a great choice for new developers.
Android SDK and Emulator Setup
You’ll need the Android Software Development Kit (SDK) to write and run your Android apps. The SDK includes the tools necessary for building Android applications and an emulator to test them on virtual devices.
3. Step-by-Step Guide to Writing Your First Android App
Setting Up Android Studio
Start by downloading and installing Android Studio from the official Android Developer website. Once installed:
Launch Android Studio and choose "Start a new Android Studio project."
Select an "Empty Activity" as the base template for your app.
Configure your project by providing an app name, choosing Kotlin or Java, and setting a minimum API level (Android version) that your app will support.
Creating Your First Android Project
Once your project is created, Android Studio will generate several folders and files for you:
MainActivity.java/Kotlin: The entry point of your application.
activity_main.xml: Defines the UI layout of your app.
AndroidManifest.xml: Declares essential information about your app, such as permissions and activities.
Understanding Android Components (Activities, Views, and Layouts)
Activities: Represent a single screen with a user interface. MainActivity is the default activity for most apps.
Views: Basic building blocks for the UI, like buttons, text fields, and images.
Layouts: Determine how views are arranged on the screen. ConstraintLayout is commonly used for flexible and responsive designs.
Writing Simple Code for Your App
Here's a basic example of adding a button in MainActivity.java and setting up a click listener:
java
Button myButton = findViewById(R.id.my_button);
myButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button click
Toast.makeText(MainActivity.this, "Button clicked!", Toast.LENGTH_SHORT).show();
}
});
This simple code displays a Toast message when the button is clicked.
4. Understanding Android Manifest and Permissions
The AndroidManifest.xml file is crucial because it defines your app's components and permissions. For example, if your app needs to access the internet, you’ll need to declare that in the manifest:
xml
<uses-permission android:name="android.permission.INTERNET"/>
5. Testing Your Android App
Using Android Emulator
The Android Emulator allows you to simulate different devices, including phones and tablets, to test your app's behavior across a variety of configurations.
Testing on Real Devices
While emulators are useful, nothing beats real-world testing. You can connect an Android device via USB, enable USB Debugging in the developer options, and test directly on the device.
Debugging and Logging
Use Android Studio’s Logcat for debugging. Logcat displays logs from the device, allowing you to track issues in real time. You can insert log messages in your code using:
java
Log.d("MainActivity", "Button was clicked!");
6. Implementing Key Features in Your App
Adding User Input Forms
User input is vital for many apps. Here's an example of capturing text input from an EditText field:
java
EditText userInput = findViewById(R.id.editText);
String inputText = userInput.getText().toString();
Handling User Navigation (Fragments and Activities)
Android apps often contain multiple screens. You can navigate between screens using Intents:
java
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
startActivity(intent);
Connecting to a Database (SQLite and Room)
If your app needs to store data locally, you can use SQLite or Google's Room library. Room abstracts SQLite's complexity and offers an easier interface for database interactions.
Working with APIs
Many modern apps fetch data from web services. You can use Retrofit or Volley to handle network requests in Android. For instance, here's a sample Retrofit setup:
java
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
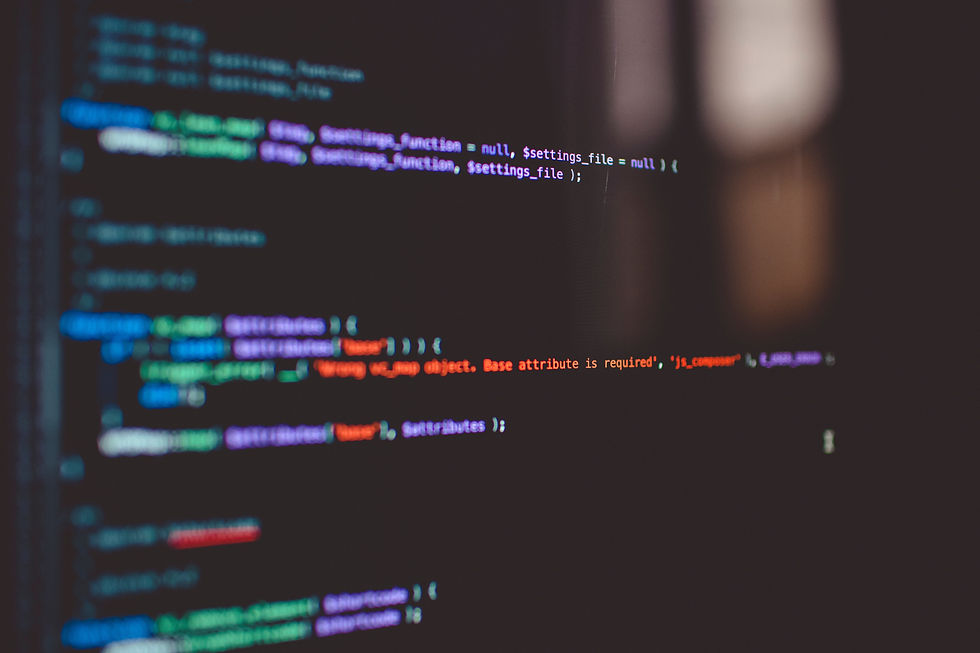
7. Designing User Interfaces
Using XML for Layouts
Android Studio uses XML to design app layouts. Here's a simple example of a LinearLayout with a button and a text view:
xml
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, Android!" />
<Button
android:id="@+id/my_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
</LinearLayout>
Material Design Principles
Material Design is a design language developed by Google. It emphasizes clean, minimal interfaces with natural motion and depth. You can implement Material Design in your Android app by using the Material Components Library.
Customizing Themes and Colors
Android provides a powerful theming system. You can define your app's theme in styles.xml and customize colors, fonts, and other UI elements.
8. How to Monetize Your Android App
In-App Purchases
In-app purchases allow users to buy additional features within your app. Android provides an In-App Billing API to facilitate this.
Ads Integration
You can integrate ads using Google AdMob. Displaying banner ads or interstitial ads can generate revenue from your free app.
Paid Apps
If your app offers premium features, you can list it as a paid app in the Google Play Store.
9. Deploying Your App to the Google Play Store
Preparing Your App for Release
Before releasing your app, you need to prepare it by signing your APK and optimizing your code for performance and size.
Generating a Signed APK
You can generate a signed APK directly from Android Studio:
Click on Build > Generate Signed Bundle / APK.
Follow the prompts to sign your app with a key.
Submitting to Google Play
To submit your app:
Sign in to Google Play Console.
Create a new app listing and upload the signed APK.
Complete the app details and pricing information, and submit it for review.
10. Maintaining and Updating Your App
After your app is live, you'll need to monitor user feedback, fix bugs, and release updates. Google Play Console offers detailed analytics to help you understand your app’s performance.
11. Conclusion
Learning how to write an Android app is both challenging and rewarding. With the right tools, proper planning, and this comprehensive guide, you’ll be well on your way to developing apps that could reach millions of users worldwide. Start small, experiment, and most importantly, keep learning. Android development is a journey, but with each step, you'll gain the skills and confidence to build something amazing.
FAQs
Q1: Do I need to know Java to write Android apps?
No, you can write Android apps in either Java or Kotlin. Kotlin is now the preferred language for Android development.
Q2: How long does it take to develop an Android app?
The time required depends on the app’s complexity. A simple app can take a few days to weeks, while a more complex app could take months.
Q3: Can I test my Android app without a physical device?
Yes, Android Studio includes an emulator that lets you test your app on a variety of virtual devices.
Q4: How can I monetize my Android app?
You can monetize your app through in-app purchases, ads, or by offering it as a paid app on the Google Play Store.
Q5: How do I ensure my app works on different screen sizes?
Use responsive layouts like ConstraintLayout and test your app on different screen sizes using the Android emulator.
Q6: Do I need a Google Play Developer account to publish apps?
Yes, you need to create a Google Play Developer account, which has a one-time registration fee of $25.
Key Takeaways
Android development can be done using Java or Kotlin with Android Studio.
Use Android SDK and emulator for testing before deploying on real devices.
The Android Manifest file is crucial for permissions and app configuration.
XML layouts help design the user interface, and Material Design enhances the UX.
Publishing on Google Play requires generating a signed APK and following guidelines for submission.
Monetize your app through in-app purchases, ads, or by offering it as a paid app.
Comments