State Machine with Practical Examples for Bug-Free Code
- Gunashree RS
- Sep 13, 2024
- 8 min read
In software development, writing reliable and bug-free code is a crucial goal. One of the most effective strategies for achieving this is using a state machine. A state machine provides a structured way to model the behavior of your software, ensuring that it can only transition between valid states, thus preventing many common bugs.
But what exactly is a state machine, and how can you use it to write better code?
In this guide, we will walk you through the concept of a state machine, and its importance, and explore detailed state machine examples. By the end of this article, you will have a clear understanding of how state machines work and how you can use them to avoid bugs and streamline your development process.
1. What is a State Machine?
A state machine is a programming model that defines the various states an application can be in and the transitions between these states triggered by events. It is a powerful concept that helps you model the behavior of complex systems in a predictable and controlled manner.
In simple terms, a state machine starts in an initial state, and based on user input or other triggers (called events), it moves or "transitions" to another state. Each state represents a particular condition or mode of operation, and only certain transitions are allowed between states.
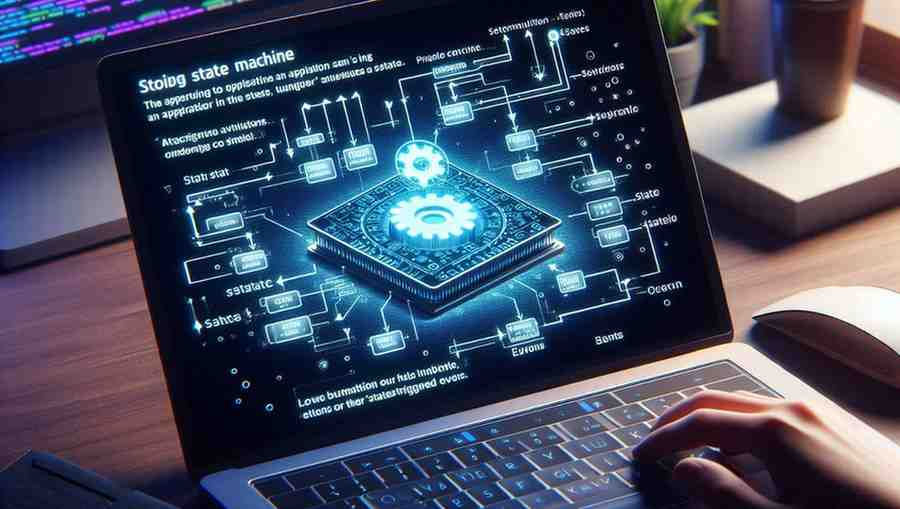
For instance, when you interact with an ATM, you transition from state to state, such as:
Idle (before you insert your card)
Card Inserted (after inserting your card)
Password Verification (after entering your password)
Service Selection (once you’ve successfully logged in)
In the context of a well-designed state machine, the ATM would never allow you to select a service without entering a valid password, thus preventing potential bugs or errors.
2. Why Use a State Machine?
When building software, especially for complex applications, ensuring that every possible state is handled correctly can be challenging. Without a state machine, developers often rely on multiple conditionals (like if statements), which can quickly become hard to maintain and error-prone.
Benefits of Using a State Machine:
Predictable Transitions: A state machine ensures that your application only transitions between valid states, which reduces the risk of bugs caused by unexpected states.
Clear Structure: State machines give your code a well-defined structure that is easier to understand, maintain, and extend.
Improved Testing: Because state transitions are well-defined, it becomes easier to write tests that cover all possible states and transitions, improving overall test coverage.
Less Code: State machines often result in less complex code, as they remove the need for handling various conditions scattered throughout your codebase.
3. State Machine Example: ATM Machine
One of the most common examples used to explain state machines is the ATM machine. Here’s a breakdown of how an ATM functions as a state machine:
Initial State: Idle
When the ATM is in its initial state, it waits for an input, typically inserting a card. This state is called Idle.
Event: Card Inserted
Once the user inserts their card, the ATM transitions to the next state: Card Inserted. It then prompts the user for a password.
Event: Correct Password Entered
If the user enters the correct password, the ATM moves to a Service Selection state, allowing the user to choose between various services like withdrawing money, checking the account balance, or transferring funds.
Event: Invalid Password Entered
If the password is incorrect, the ATM transitions to an Error State, displaying a message like "Invalid Password. Please try again."
Event: Selecting a Service
Depending on the user’s choice (withdrawal, check balance, etc.), the ATM moves to the appropriate state, executes the service and eventually returns to Idle when the transaction is complete.
This example highlights how state machines help define specific states and control how an application transitions between them based on user actions or inputs.
4. How to Design a State Machine for Bug-Free Code
To design a bug-free state machine, you must think through all possible states and transitions. This ensures that your application will handle every scenario gracefully, without crashing or behaving unpredictably.
4.1 Identify Initial State
The first step in designing a state machine is identifying the initial state of your application. This is the state your system starts in before any interactions occur. For example, in an ATM, the initial state is Idle.
4.2 Identify Events
Next, determine what events will trigger transitions between states. These could be user actions, such as button clicks or form submissions, or other types of input like receiving a signal from another system.
For the ATM example, possible events include:
Inserting a card
Entering a password
Selecting a service
Removing the card
4.3 Determine Transitions
Once you’ve identified the events, map out how your application will transition between states. Ensure that each event is handled correctly and that invalid transitions are prevented.
For instance, if a user selects a service without entering the correct password, the system should either prompt the user to enter the password or return to the initial state.
5. Real-World State Machine Examples
State machines are not limited to theoretical applications. Many real-world systems use state machines to ensure predictable and bug-free behavior. Below are a few practical examples.
5.1 Login Workflow
In most web applications, logging in follows a state machine pattern. Here’s a simple breakdown:
Initial State: Login page
Event: User enters credentials
Transition: If the credentials are correct, transition to Logged In; if incorrect, display an Error State.
Event: If the user has been inactive for a long time, transition to Session Expired.
5.2 E-commerce Order Processing
E-commerce websites rely heavily on state machines to manage order statuses:
Initial State: Order placed
Event: Payment successful
Transition: If payment is confirmed, the order moves to Processing; otherwise, transition to Payment Failed.
Event: Shipment dispatched
Transition: Move to Shipped, and eventually to Delivered once the customer receives the product.
5.3 Elevator Control System
An elevator’s behavior can also be modeled using a state machine:
Initial State: Doors closed
Event: Button pressed (e.g., floor 3)
Transition: The elevator moves to Moving state, then to Arrived at Floor once it reaches floor 3.
Event: Doors opened
Transition: The elevator transitions to the Idle state once the doors are closed.
6. Benefits of Using a State Machine
State machines offer several key benefits for developing software systems:
Controlled Complexity: By dividing an application into states, developers can reduce the complexity of the overall codebase.
Consistency: State machines ensure that transitions happen in a predictable way, preventing unexpected behaviors.
Simplified Debugging: Since all transitions are predefined, it’s easier to debug issues related to unexpected states.
Enhanced Testing: The structured nature of a state machine makes it easier to write unit and integration tests that cover every possible state and transition.
7. Common Mistakes in State Machine Design
While state machines are powerful, they can still lead to problems if not designed correctly. Common mistakes include:
Overcomplicating the State Machine: Trying to cover every possible scenario with a complex web of states can make the system harder to maintain. Focus on key states and transitions.
Not Accounting for Invalid Transitions: Ensure that your application handles invalid transitions gracefully, returning to a known state when something unexpected happens.
Ignoring Error States: Always include error states to handle unexpected inputs or failed operations. This prevents the application from entering undefined states.
8. How State Machines Prevent Bugs
State machines help prevent bugs by enforcing strict rules about how an application transitions between states. By defining a finite set of states and events, developers can avoid many common problems, such as:
Unintended States: State machines ensure that the application can only be in one of the predefined states, reducing the risk of unintended behaviors.
Race Conditions: By controlling transitions, state machines prevent multiple conflicting events from occurring simultaneously.
Logical Errors: Since the transitions are well-defined, state machines reduce the chance of logic errors in how the application responds to events.
9. State Machine Design Best Practices
To make the most out of a state machine, follow these best practices:
Start Simple: Begin by identifying key states and the most common transitions. You can expand later if necessary.
Handle All Events: Ensure that your state machine accounts for all possible events, including unexpected or invalid inputs.
Use Visual Diagrams: When designing complex state machines, create visual diagrams to map out the states and transitions. This helps in better understanding and communication among the team.
Modularize Your States: Break down your states into smaller, more manageable components. This makes your code easier to maintain and test.
10. Conclusion
A state machine is an incredibly valuable tool for writing reliable and bug-free code. By carefully defining the states an application can be in and the events that cause it to transition between states, developers can ensure predictable behavior and reduce the risk of errors. Whether you're building a simple web application or a complex system, using a state machine can help you create clean, maintainable, and bug-free code.
By following best practices and learning from real-world examples like the ATM machine or login workflows, you can apply the power of state machines to your own projects and ensure that your software behaves exactly as expected.
11. Frequently Asked Questions (FAQs)
Q1: What is a state machine in programming?
A state machine is a programming model that defines the various states an application can be in and the transitions between those states triggered by events.
Q2: How can state machines prevent bugs in software?
State machines prevent bugs by enforcing strict rules about how an application transitions between states, ensuring that the application cannot enter an invalid or undefined state.
Q3: Can state machines be used in web applications?
Yes, state machines are commonly used in web applications to manage workflows like login processes, order processing, and form submissions.
Q4: What is the difference between a state and a transition?
A state represents a particular condition or mode of operation in an application, while a transition is the process of moving from one state to another in response to an event.
Q5: Are state machines difficult to implement?
No, state machines are not inherently difficult to implement. By starting with a simple design and gradually adding complexity, you can effectively use state machines in your projects.
Q6: Can state machines handle unexpected events?
Yes, state machines can be designed to handle unexpected or invalid events by transitioning to error states or rejecting the event altogether.
12. Key Takeaways
A state machine models the behavior of an application through states and transitions.
State machines enforce predictable transitions, reducing the risk of bugs and logical errors.
Real-world examples like ATM machines and login workflows demonstrate the effectiveness of state machines.
Best practices for designing state machines include keeping things simple, handling all possible events, and modularizing states.
Implementing state machines in your projects leads to better structure, improved testing, and easier debugging.
Comments