Selenium Chrome: Guide to Testing Automation
- Gunashree RS
- Aug 29, 2024
- 7 min read
Selenium has long been the go-to tool for web developers and QA engineers for automating browser interactions and testing web applications. With the release of Selenium 4, the framework has become even more powerful, particularly through its enhanced integration with Chrome DevTools. This integration opens up a world of possibilities, allowing you to execute sophisticated testing scenarios, simulate different conditions, and capture crucial metrics with ease.
In this comprehensive guide, we'll dive deep into how you can leverage Selenium Chrome to elevate your automated testing. We’ll explore the new Chrome DevTools APIs in Selenium 4, showcasing how they can be used to simulate device modes, manipulate network conditions, mock geolocations, capture network traffic, and more. By the end of this guide, you'll have a solid understanding of how to use Selenium Chrome to enhance your testing processes, making them more robust, efficient, and insightful.
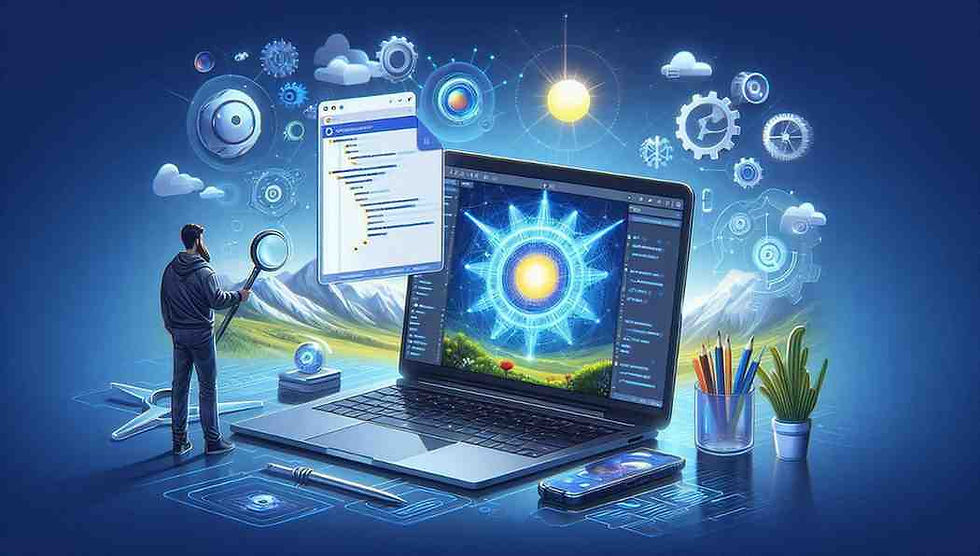
What is Selenium Chrome?
Selenium Chrome refers to the integration of Selenium WebDriver with Google Chrome, allowing developers and testers to automate web applications on the Chrome browser. With the release of Selenium 4, this integration has been significantly enhanced through the addition of native support for Chrome DevTools Protocol (CDP).
Key Features of Selenium Chrome Integration:
Automated Browser Testing: Selenium WebDriver allows you to automate interactions with web elements on Chrome, such as clicking buttons, entering text, and navigating pages.
Chrome DevTools Protocol (CDP) Integration: Selenium 4 introduces support for CDP, enabling testers to access advanced features like network interception, geolocation mocking, and performance metrics collection.
Cross-Browser Testing: Selenium Chrome can be used alongside other browser drivers to perform cross-browser testing, ensuring that web applications work consistently across different browsers.
Enhanced Debugging Capabilities: With access to Chrome DevTools, testers can debug JavaScript, inspect elements in the DOM, and monitor network activity directly within their Selenium tests.
Selenium Chrome provides a powerful and flexible testing environment that can be tailored to meet the specific needs of your project, making it an essential tool for modern web development.
The Role of Chrome DevTools in Selenium Testing
Chrome DevTools is a set of web development tools built directly into Google Chrome and other Chromium-based browsers like Microsoft Edge. These tools allow developers to inspect, debug, and analyze the performance of web applications. With the introduction of CDP in Selenium 4, testers can now harness the power of Chrome DevTools directly within their Selenium scripts.
What Can You Do with Chrome DevTools in Selenium?
Inspect and Modify DOM Elements: You can inspect and modify HTML and CSS elements in real-time to see how changes affect the webpage.
Simulate Mobile Devices: Chrome DevTools allows you to emulate mobile devices, testing how your application responds to different screen sizes and orientations.
Network Throttling: Simulate different network conditions (e.g., 3G, 4G) to test how your application performs under various network speeds.
Geolocation Testing: Mock geolocation to test location-based features of your web application without physically moving to different locations.
Capture and Analyze Network Traffic: Intercept and analyze HTTP requests and responses to understand the behavior of your application and identify potential issues.
By integrating these capabilities with Selenium, you can create more comprehensive and accurate tests, ensuring that your web applications perform optimally in real-world scenarios.
Selenium 4 Chrome DevTools APIs: New Capabilities
Selenium 4 introduces the ChromiumDriver class, which includes methods to access Chrome DevTools directly from your Selenium tests. This integration allows you to execute Chrome DevTools Protocol (CDP) commands, enabling advanced testing scenarios that were previously difficult or impossible to automate.
Key Methods in ChromiumDriver:
getDevTools(): This method returns a DevTools object, allowing you to send CDP commands using the send() method. The commands are wrapped by Selenium APIs, making them easy to use within your tests.
executeCdpCommand(): This method allows you to execute raw CDP commands directly by passing the command name and parameters. It provides more flexibility but requires a deeper understanding of CDP.
Use Cases for Selenium 4 Chrome DevTools APIs
Simulating Device Mode:
Use Case: Test how your web application responds to different screen sizes and device types (e.g., smartphones, tablets).
Example: Using the Emulation.setDeviceMetricsOverride command, you can set custom screen dimensions and device scale factors to simulate different devices.
java
Map<String, Object> deviceMetrics = new HashMap<>();
deviceMetrics.put("width", 600);
deviceMetrics.put("height", 1000);
deviceMetrics.put("mobile", true);
deviceMetrics.put("deviceScaleFactor", 50);
driver.executeCdpCommand("Emulation.setDeviceMetricsOverride", deviceMetrics);
Simulating Network Speed:
Use Case: Test how your web application performs under different network conditions, such as slow 2G connections.
Example: Using the Network.emulateNetworkConditions command, you can simulate various network conditions, including latency and download/upload speeds.
java
devTools.send(Network.emulateNetworkConditions(
false,
20, // latency
20, // download throughput
50, // upload throughput
Optional.of(ConnectionType.CELLULAR2G)
));
Mocking Geolocation:
Use Case: Test location-based features of your web application, such as different offers or currency conversions based on user location.
Example: Using the Emulation.setGeolocationOverride command, you can mock the user's geolocation coordinates.
java
devTools.send(Emulation.setGeolocationOverride(
Optional.of(35.8235), // latitude
Optional.of(-78.8256), // longitude
Optional.of(100) // accuracy
));
Capturing HTTP Requests:
Use Case: Capture and analyze the network traffic generated by your web application to identify potential issues with HTTP requests.
Example: Use the Network.requestWillBeSent event listener to capture HTTP requests.
java
devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty()));
devTools.addListener(Network.requestWillBeSent(), entry -> {
System.out.println("Request URI: " + entry.getRequest().getUrl());
System.out.println("Method: " + entry.getRequest().getMethod());
});
Accessing Console Logs:
Use Case: Capture and analyze console logs to debug issues during test execution.
Example: Use the Log.entryAdded event listener to capture console logs.
java
devTools.send(Log.enable());
devTools.addListener(Log.entryAdded(), logEntry -> {
System.out.println("Log: " + logEntry.getText());
System.out.println("Level: " + logEntry.getLevel());
});
These capabilities make Selenium 4 a more versatile and powerful tool for automated testing, allowing you to simulate real-world conditions and capture detailed insights into your web application's performance.
Implementing Advanced Testing Scenarios with Selenium Chrome
Let’s explore some advanced testing scenarios that demonstrate the full power of Selenium Chrome integration with DevTools.
1. Simulating Device Mode with CDP
In today’s mobile-first world, ensuring that your web application is responsive across all devices is critical. Selenium Chrome allows you to simulate various device metrics, including screen size, orientation, and pixel density, using the Emulation.setDeviceMetricsOverride CDP command.
Example Scenario:
Goal: Test the responsiveness of a web application when viewed on a mobile device with a 600x1000 resolution and a device scale factor of 50.
Code Implementation:
java
Map<String, Object> deviceMetrics = new HashMap<>();
deviceMetrics.put("width", 600);
deviceMetrics.put("height", 1000);
deviceMetrics.put("mobile", true);
deviceMetrics.put("deviceScaleFactor", 50);
driver.executeCdpCommand("Emulation.setDeviceMetricsOverride", deviceMetrics);
driver.get("https://www.example.com");
2. Simulating Network Speed
Web applications must perform well even under poor network conditions. Selenium Chrome allows you to simulate different network speeds and test how your application behaves under conditions like slow 2G connections or intermittent connectivity.
Example Scenario:
Goal: Test the performance of a web application under a simulated 2G network.
Code Implementation:
java
devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty()));
devTools.send(Network.emulateNetworkConditions(
false,
100, // latency
5000, // download throughput
2000, // upload throughput
Optional.of(ConnectionType.CELLULAR2G)
));
driver.get("https://www.example.com");
3. Mocking Geolocation
Many web applications offer features based on the user's location, such as local news, weather, or language settings. With Selenium Chrome, you can mock geolocation to test these features without needing to be in the actual location.
Example Scenario:
Goal: Test a web application’s location-based features by simulating a user in Raleigh, North Carolina.
Code Implementation:
java
devTools.send(Emulation.setGeolocationOverride(
Optional.of(35.7796), // latitude
Optional.of(-78.6382), // longitude
Optional.of(100) // accuracy
));
driver.get("https://www.example.com");
4. Capturing and Analyzing Network Traffic
Understanding how your web application interacts with the server is crucial for optimizing performance and debugging issues. Selenium Chrome allows you to capture and analyze HTTP requests directly from your tests.
Example Scenario:
Goal: Capture all HTTP requests made by a web application during testing.
Code Implementation:
java
devTools.send(Network.enable(Optional.empty(), Optional.empty(), Optional.empty()));
devTools.addListener(Network.requestWillBeSent(), request -> {
System.out.println("Request URL: " + request.getRequest().getUrl());
System.out.println("Request Method: " + request.getRequest().getMethod());
});
driver.get("https://www.example.com");
5. Capturing Console Logs
Console logs provide valuable insights during test execution, especially when debugging complex issues. Selenium Chrome allows you to capture these logs programmatically within your tests.
Example Scenario:
Goal: Capture and analyze console logs during the execution of a web application.
Code Implementation:
java
devTools.send(Log.enable());
devTools.addListener(Log.entryAdded(), logEntry -> {
System.out.println("Log: " + logEntry.getText());
System.out.println("Level: " + logEntry.getLevel());
});
driver.get("https://www.example.com");
Conclusion
Selenium Chrome, enhanced by the new capabilities introduced in Selenium 4, is an incredibly powerful tool for web developers and QA engineers. By integrating Chrome DevTools Protocol, Selenium now allows for advanced testing scenarios that were previously difficult or impossible to automate. Whether you’re simulating different devices, manipulating network conditions, mocking geolocation, capturing network traffic, or accessing console logs, Selenium Chrome provides the tools you need to ensure your web application performs flawlessly across all environments.
With these new features, you can create more comprehensive, accurate, and robust automated tests, ultimately delivering a higher quality product to your users. As web development continues to evolve, mastering Selenium Chrome will be essential for staying ahead of the curve in testing automation.
Key Takeaways
Enhanced Testing Capabilities: Selenium 4 introduces support for Chrome DevTools Protocol, enabling advanced testing scenarios directly from your Selenium scripts.
Device Simulation: Use Selenium Chrome to simulate different devices and screen sizes, ensuring your application is fully responsive.
Network Throttling: Test how your application performs under various network conditions, from slow 2G connections to high-speed broadband.
Geolocation Mocking: Mock geolocation to test location-based features without needing to be in the actual location.
Network Traffic Analysis: Capture and analyze HTTP requests made by your web application to identify potential performance issues.
Console Log Capture: Access and analyze console logs during test execution to debug and troubleshoot issues effectively.
Cross-Browser Compatibility: Selenium Chrome can be used alongside other browser drivers to ensure your web application works consistently across all major browsers.
Robust Automation Tool: Selenium Chrome’s integration with DevTools makes it an indispensable tool for modern web testing, offering unparalleled control and flexibility.
Frequently Asked Questions (FAQs)
1. What is Selenium Chrome?
Selenium Chrome refers to the integration of Selenium WebDriver with the Chrome browser, allowing developers and testers to automate web applications on Chrome.
2. What is Chrome DevTools Protocol (CDP)?
CDP is a set of tools built into Chrome that allows developers to inspect, debug, and analyze web applications. Selenium 4 introduces support for CDP, enabling advanced testing scenarios within Selenium tests.
3. How can I simulate different devices in Selenium Chrome?
You can use the Emulation.setDeviceMetricsOverride CDP command to simulate different screen sizes, orientations, and device types in your Selenium tests.
4. How do I capture network traffic with Selenium Chrome?
Use the Network.enable CDP command to start capturing network traffic, and listen for Network.requestWillBeSent events to analyze HTTP requests made by your web application.
5. Can I mock geolocation in Selenium Chrome?
Yes, you can use the Emulation.setGeolocationOverride CDP command to mock geolocation and test location-based features in your web application.
6. How do I capture console logs in Selenium Chrome?
Enable log capturing with the Log.enable command and add a listener for Log.entryAdded events to capture and analyze console logs during test execution.
7. What are the benefits of using Selenium 4 with Chrome DevTools?
Selenium 4’s integration with Chrome DevTools provides advanced testing capabilities, such as device simulation, network throttling, geolocation mocking, and performance metrics collection.
8. Is Selenium Chrome suitable for cross-browser testing?
Yes, Selenium Chrome can be used alongside other browser drivers like FirefoxDriver and EdgeDriver to perform cross-browser testing and ensure consistent performance across all major browsers.
Comments