Mastering Python Type Checker: Guide for Developers
- Gunashree RS
- Sep 27, 2024
- 9 min read
Python has long been praised for its simplicity and flexibility, but one aspect that many developers miss is its dynamic typing. While dynamic typing provides flexibility, it also introduces potential pitfalls, like type errors that can go unnoticed until runtime. Enter Python type checkers—tools that allow developers to check for type-related issues before the code is even executed. With static type checking, you can catch type mismatches early, improve code quality, and reduce bugs.
In this comprehensive guide, we’ll cover what Python type checking is, the benefits of using it, popular tools like Mypy, and how to integrate static type checking into your Python projects.
1. What is a Python Type Checker?
A Python type checker is a tool that analyzes your Python code to ensure that the data types specified in the code align with how the values are actually used. Python is a dynamically typed language, meaning you don't have to declare variable types explicitly, but type checkers can enforce type consistency by analyzing your code for type mismatches.
Type checkers help detect issues like:
Variables used with incorrect types
Functions returning unexpected types
Inconsistent use of type hints and annotations
Incompatible assignments or operations based on types
By running a Python-type checker, you can catch potential bugs early, reduce runtime errors, and improve overall code quality.
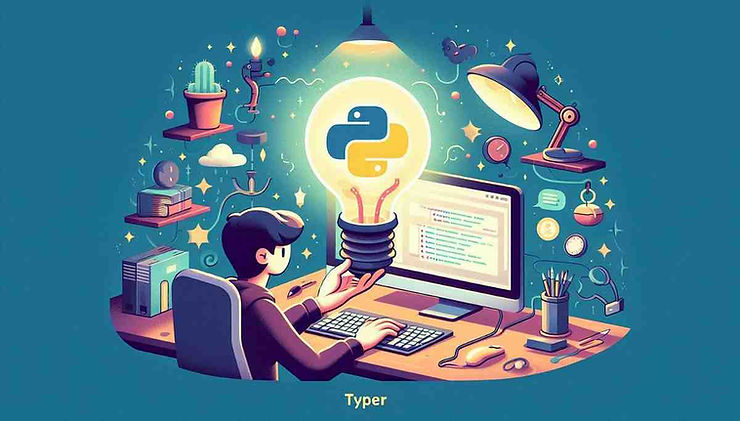
2. Why Use Type Checking in Python?
While Python's dynamic nature is one of its strengths, it also leads to several challenges:
Runtime errors: Type errors are only caught when the erroneous code is executed, making them harder to track down.
Reduced readability: Without type hints, it can be difficult for developers to understand what types a function accepts or returns.
Harder collaboration: Dynamic typing can make large projects harder to maintain, especially when multiple developers are involved.
Type-checking solves many of these problems:
Early error detection: Type checkers catch type mismatches before running the program.
Improved documentation: Type hints make the code easier to read and understand.
Enhanced collaboration: Enforcing type checks ensures that all developers adhere to type consistency, making the codebase easier to manage.
3. Dynamic Typing vs. Static Typing in Python
Python is dynamically typed by design, meaning you don’t have to declare the type of a variable when you create it. The type of a variable is inferred at runtime. For example:
python
x = 10 # x is inferred as an integer
x = "Hello" # Now x is a string
While this flexibility is useful for rapid development, it can lead to errors when types change unexpectedly or when functions receive unexpected input.
Static typing, on the other hand, involves explicitly specifying the type of variables and functions at the time of declaration. With tools like Mypy, you can introduce static type hints in Python to ensure type consistency, like so:
python
def add_numbers(a: int, b: int) -> int:
return a + b
Here, we are explicitly stating that both a and b must be integers, and the function will return an integer. Type checkers enforce these rules.
4. Introduction to Mypy: The Most Popular Python Type Checker
Mypy is the most widely used static type checker for Python. It integrates seamlessly with Python’s type hinting system (introduced in Python 3.5), allowing developers to enforce type safety without changing Python’s dynamic nature.
Key Features of Mypy:
Type checking for functions and variables: Mypy checks if the types of variables and function arguments match the expected types.
Support for Python’s type hinting system: You can add type hints using Python’s built-in syntax and Mypy will validate them.
Detecting incorrect return types: It checks if the return value of functions matches the annotated return type.
Mypy works by analyzing your Python code comparing the actual types of variables and returning values to the expected types as specified by your annotations. If a mismatch is found, Mypy reports it as an error.
5. How to Implement Static Type Checking in Python
Static type checking can be easily added to any Python project. Here’s how to do it:
Step 1: Add-Type Hints
Begin by adding type hints to your Python functions and variables. For example:
python
def greet(name: str) -> str:
return f"Hello, {name}"
In this case, we’re specifying that the name is a string, and the function will return a string.
Step 2: Install Mypy
To install Mypy, run:
bash
pip install mypy
Step 3: Run Mypy
Once Mypy is installed, you can run it against your Python code to perform type checks:
bash
mypy your_script.py
Mypy will report any type of mismatches it finds, allowing you to fix them before running your code.
6. Configuring Mypy for Python Projects
Mypy can be customized to suit your project’s needs. The easiest way to configure it is by adding a mypy.ini file or a section in your setup.cfg.
Here’s an example mypy.ini file:
ini
[mypy]
ignore_missing_imports = True
disallow_untyped_defs = True
warn_unused_ignores = True
This configuration ignores missing imports, disallows untyped function definitions, and warns if any # type: ignore comments are not necessary.
You can also configure Mypy to run as part of your continuous integration (CI) pipeline to enforce type checks automatically.
7. Common Issues Detected by Python Type Checkers
When using a Python type checker like Mypy, there are several common issues it can detect:
1. Type Hint Errors
These occur when the type hints in the function signature don’t match the types actually passed to or returned by the function.
python
def add(a: int, b: int) -> int:
return str(a + b) # Error: return type should be int, but it's str
2. Inconsistent Type Annotations
Mypy can catch inconsistencies between the types of variables within the same scope.
python
x: int = 10
x = "Hello" # Error: incompatible types
3. Invalid Return Types
Mypy checks whether the actual return type of a function matches the type specified in the return annotation.
python
def get_number() -> int:
return "5" # Error: should return int, but returns str
4. Use of Deprecated Type Hints
Type checkers will flag the use of outdated or deprecated type hints and suggest modern alternatives.
8. Silencing Type Check Issues in Python
There may be cases where a type checker flags a false positive or where you want to intentionally bypass type checking for a specific line. Python allows you to silence type checks by using comments like # type: ignore.
For example:
python
def process_data(data: str) -> int:
return int(data) # type: ignore
This tells the type checker to skip checking this particular line. You can also silence specific issues with # skipcq: TYP-001 for greater control.
9. How to Integrate Mypy with Continuous Integration (CI) Pipelines
To maintain code quality, it’s a good idea to integrate Mypy into your CI pipeline. This ensures that type checks are run automatically with each commit, preventing type errors from making it into production.
Here’s an example of how to integrate Mypy with a GitHub Actions CI pipeline:
yaml
name: Python Type Checking
on: [push, pull_request]
jobs:
mypy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.8'
- name: Install dependencies
run: |
pip install mypy
- name: Run Mypy
run: mypy your_project/
This setup ensures that Mypy checks are run whenever a commit or pull request is made, preventing untyped or improperly typed code from being merged.
10. Advanced Type Checking: Type Hints, Generics, and Protocols
Python’s type hinting system is powerful and supports advanced features like Generics, Union types, and Protocols. These allow for more flexible type definitions, especially in libraries and frameworks.
Generics
Generics allow you to create functions and classes that can handle multiple types. For example, a function that works with lists of any type:
python
from typing import TypeVar, List
T = TypeVar('T')
def get_first_item(items: List[T]) -> T:
return items[0]
Union Types
Union types let you specify multiple potential types for a variable or function argument.
python
from typing import Union
def process_value(value: Union[int, str]) -> None:
if isinstance(value, int):
print(f"Processing number: {value}")
else:
print(f"Processing string: {value}")
Protocols
Protocols define a contract that a class must adhere to, even if it doesn’t explicitly inherit from a particular superclass.
11. Best Practices for Type Checking in Large Python Projects
For large projects, type-checking can become complex. Here are some best practices:
Start small: Add type hints gradually, starting with critical functions.
Use Optional[]: Clearly define when variables can be None using Optional.
Keep type hints up to date: As your project evolves, ensure that type hints reflect the current state of the code.
Avoid over-complication: While Python’s type system is flexible, overusing advanced types like Union or Generics can make the code harder to read.
12. Static Type Checking with DeepSource
DeepSource recently announced the integration of static type checking for Python, powered by Mypy. With a simple configuration change in the .deepsource.toml file, developers can leverage DeepSource to automate type-checking as part of their code analysis workflow.
Here’s how to enable static type checking on DeepSource:
toml
version = 1
[[analyzers]]
name = "python"
enabled = true
[analyzers.meta]
type_checker = "mypy"
DeepSource honors the mypy.ini configuration, ensuring that your type-checking settings are consistent across local development and CI environments.
13. How Type Checkers Improve Code Quality and Collaboration
Static type checking offers several long-term benefits for teams:
Reduces bugs: By catching type errors early, developers can avoid costly runtime errors.
Improves documentation: Type hints act as inline documentation, helping new developers quickly understand the codebase.
Encourages consistency: With enforced type hints, teams can adhere to uniform standards, reducing confusion and errors.
Enhances refactoring: Type checkers ensure that refactoring doesn’t introduce unintended type-related bugs, making code maintenance easier.
14. Overcoming Challenges with Python Type Checkers
While type checkers are powerful, they can introduce new challenges:
False positives: Sometimes, type checkers will flag valid code as problematic. Developers need to know when and how to ignore these false positives.
Learning curve: For teams new to type hints, there can be a learning curve. However, once the basics are mastered, type hints greatly improve the coding experience.
Legacy code: Introducing type checking to a legacy codebase can be daunting. Start by adding types to the most critical functions and gradually expand coverage.
15. The Future of Static Type Checking in Python Development
As Python continues to evolve, the role of static type checking will grow. Tools like Mypy and DeepSource are pushing the boundaries, making type-checking more accessible and integrated into modern development workflows. We can expect even more advanced type-checking features, better integration with IDEs, and deeper CI/CD integrations in the near future.
Conclusion
The Python type checker has revolutionized the way developers write and maintain Python code. By introducing static type checking with tools like Mypy, developers can catch errors early, enhance code readability, and improve collaboration across teams. Whether you’re working on a small script or a large-scale project, type checkers can streamline your workflow and help you build more reliable, maintainable software.
By integrating static type checking into your CI pipeline, following best practices, and leveraging tools like DeepSource, you can ensure that your Python code remains high-quality and error-free. The future of Python development lies in embracing these powerful tools to deliver better software faster.
Key Takeaways:
Python type checkers enforce type consistency, reducing runtime errors.
Mypy is the most popular static type checker for Python, supporting advanced features like generics and protocols.
Type-checking improves code quality, readability, and collaboration.
Tools like DeepSource automate static type checking, making it easier to integrate into CI pipelines.
Use type hints gradually and consistently to maintain a clean and maintainable codebase.
FAQs
Q1: What is a Python type checker?
A: A Python type checker is a tool that ensures type hints in your code align with the actual data types used, detecting errors before runtime.
Q2: Why should I use static type checking in Python?
A: Static type checking helps catch type-related errors early, improves code documentation, and makes collaboration easier by enforcing type consistency.
Q3: What is Mypy?
A: Mypy is a static type checker for Python that checks for type errors in code annotated with type hints.
Q4: How do I silence a false positive in Mypy?
A: You can silence specific lines in Mypy by using the # type: ignore comment or # skipcq: TYP-001.
Q5: Can I integrate Mypy into my CI pipeline?
A: Yes, Mypy can be integrated into CI pipelines like GitHub Actions to enforce type checks on every commit.
Q6: What’s the difference between dynamic and static typing?
A: Dynamic typing infers types at runtime, while static typing involves explicitly declaring types and checking them before runtime.
Q7: What are type hints in Python?
A: Type hints are annotations that specify the expected data types of variables, function arguments, and return values in Python code.
Q8: Can I use Mypy with a legacy codebase?
A: Yes, you can gradually introduce type-checking to a legacy codebase by adding type hints to critical functions and expanding from there.