Implicit Wait in Selenium: Guide to Automated Testing Delays 2025
- Gunashree RS
- 6 hours ago
- 7 min read
Web automation testing has revolutionized how development teams ensure application quality, but it comes with unique challenges. One of the most frustrating issues testers face is dealing with dynamic web elements that load at unpredictable speeds. This is where implicit wait in Selenium becomes your secret weapon—a powerful feature that can transform unreliable, flaky tests into robust, dependable automation scripts.
If you've ever experienced tests that pass one minute and fail the next due to timing issues, you're not alone. Modern web applications are increasingly dynamic, with AJAX calls, progressive loading, and complex JavaScript interactions that can make element timing unpredictable. Understanding and properly implementing implicit wait in Selenium is crucial for any serious test automation effort.
This comprehensive guide will walk you through everything you need to know about implicit wait in Selenium, from basic concepts to advanced implementation strategies that will make your automation framework more reliable and maintainable.
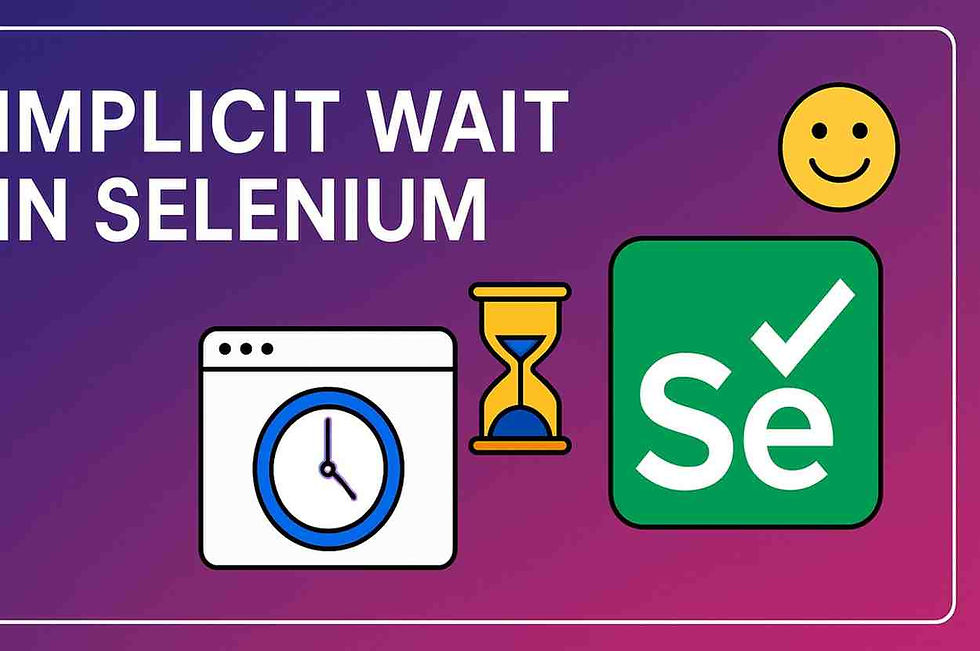
Understanding the Fundamentals of Selenium Waits
Before diving deep into implicit wait specifically, it's essential to understand why waits are necessary in the first place. When Selenium executes test scripts, it operates at machine speed—much faster than web pages can load or elements can appear. This speed mismatch creates a fundamental problem: your test script might try to interact with elements that haven't finished loading yet.
The Core Problem: Speed Mismatch
Consider this scenario: your test script clicks a button that triggers an AJAX request to load new content. Without proper waits, Selenium might immediately try to interact with the new content before it has fully loaded, resulting in a
NoSuchElementException or other failures. This isn't a bug in your application—it's simply the reality of how web applications work.
Types of Waits in Selenium
Selenium provides two primary wait mechanisms:
Implicit Wait: A global setting that applies to all element lookups throughout your test session
Explicit Wait: Targeted waits for specific conditions on particular elements
Each approach has its place in a well-designed automation framework, and understanding when to use each is crucial for building reliable tests.
Deep Dive into Implicit Wait in Selenium
Implicit wait in Selenium is essentially a global timeout setting that tells the WebDriver to wait for a specified amount of time when searching for elements. Once set, this wait applies to all subsequent element lookup operations throughout the entire test session.
How Implicit Wait Functions
When you set an implicit wait, Selenium doesn't immediately throw a NoSuchElementException if an element isn't found. Instead, it continuously polls the DOM for the specified duration, checking if the element has appeared. Only if the element remains absent after the timeout period will Selenium throw an exception.
This polling mechanism is particularly effective for handling:
Elements that load after page initialization
Dynamic content generated by JavaScript
Components that appear after AJAX requests
Progressive page loading scenarios
Key Characteristics of Implicit Wait
Global Application: Unlike explicit waits that target specific elements, implicit wait applies to every element lookup operation in your test session.
One-Time Configuration: You typically set implicit wait once at the beginning of your test session, and it remains active until you modify or end the session.
Automatic Polling: Selenium automatically handles the polling logic, repeatedly checking for elements at regular intervals.
Exception Handling: If elements aren't found within the specified timeout, Selenium throws appropriate exceptions that your test framework can catch and handle.
Implementation Guide: Setting Up Implicit Wait
Basic Syntax and Configuration
The syntax for implementing implicit wait varies slightly between programming languages, but the concept remains consistent. Here's how to set it up in the most common languages:
Java Implementation:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import java.time.Duration;
public class ImplicitWaitExample {
public static void main(String[] args) {
WebDriver driver = new ChromeDriver();
// Set implicit wait to 15 seconds
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(15));
// Navigate to your application
driver.get("https://example.com");
// All subsequent element lookups will use the 15-second wait
driver.findElement(By.id("dynamic-element")).click();
driver.quit();
}
}
Python Implementation:
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
# Set implicit wait to 15 seconds
driver.implicitly_wait(15)
# Navigate to your application
driver.get("https://example.com")
# All element lookups will now wait up to 15 seconds
driver.find_element(By.ID, "dynamic-element").click()
driver.quit()
Advanced Configuration Options
Modern Selenium versions offer more granular control over timeout settings:
Timeout Type | Purpose | Typical Duration |
Implicit Wait | Element lookup timeout | 10-30 seconds |
Page Load Timeout | Complete page loading | 30-60 seconds |
Script Timeout | JavaScript execution | 30-60 seconds |
// Comprehensive timeout configuration
driver.manage().timeouts()
.implicitlyWait(Duration.ofSeconds(20))
.pageLoadTimeout(Duration.ofSeconds(60))
.scriptTimeout(Duration.ofSeconds(30));
Best Practices for Implicit Wait Implementation
Choosing Optimal Timeout Values
Selecting the right timeout duration is crucial for balancing test reliability with execution speed. Consider these factors:
Application Characteristics:
Network-dependent applications may need longer timeouts
Local applications typically require shorter waits
Applications with heavy JavaScript processing need moderate timeouts
Test Environment:
Development environments may be slower than production
CI/CD pipelines might have resource constraints
Cloud-based testing platforms may have variable performance
Recommended Timeout Ranges
Environment Type | Recommended Timeout | Rationale |
Local Development | 10-15 seconds | Fast feedback loop |
Staging/QA | 15-20 seconds | Realistic conditions |
Production Testing | 20-30 seconds | Account for real-world delays |
CI/CD Pipeline | 15-25 seconds | Balance speed and reliability |
Common Implementation Mistakes
Setting Excessive Timeouts: While longer timeouts might seem safer, they can significantly slow down test execution when elements genuinely don't exist.
Forgetting to Set Timeouts: Many test failures occur because developers forget to implement any wait strategy, leading to race conditions.
Mixing Wait Strategies Incorrectly: Combining implicit and explicit waits can lead to unexpected behavior and longer-than-intended wait times.
Implicit Wait vs. Explicit Wait: When to Use Each
Understanding the differences between implicit and explicit waits is crucial for building effective automation frameworks:
Implicit Wait Advantages
Simplicity: Set once and forget
Global Coverage: Applies to all element lookups
Consistency: Uniform behavior across your test suite
Reduced Code: No need to specify waits for each element
Explicit Wait Advantages
Precision: Wait for specific conditions
Flexibility: Different timeouts for different elements
Condition-Based: Wait for visibility, clickability, text content, etc.
Better Control: More granular timeout management
Hybrid Approach Strategy
Many successful automation frameworks use a combination approach:
Set a reasonable implicit wait (10-15 seconds) as a safety net
Use explicit waits for critical or slow-loading elements
Implement custom wait conditions for complex scenarios
Troubleshooting Common Implicit Wait Issues
Performance Optimization Strategies
Minimize Unnecessary Waits: Avoid setting implicit waits longer than necessary, as they can significantly impact test execution time.
Monitor Test Execution Times: Track how implicit wait settings affect your overall test suite performance.
Use Explicit Waits for Critical Elements: For elements that require specific conditions (like visibility or clickability), explicit waits are more appropriate.
Debugging Techniques
When tests fail due to timing issues:
Increase Logging: Add detailed logging to understand element lookup behavior
Use Browser Developer Tools: Inspect the DOM to understand element loading patterns
Test in Different Environments: Verify that timing issues aren't environment-specific
Monitor Network Conditions: Slow networks can affect element loading times
Advanced Implicit Wait Techniques
Dynamic Timeout Adjustment
For applications with varying performance characteristics, consider implementing dynamic timeout adjustment:
public class DynamicImplicitWait {
private WebDriver driver;
private int baseTimeout = 10;
public void adjustTimeoutForEnvironment() {
String environment = System.getProperty("test.environment");
switch(environment) {
case "local":
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(baseTimeout));
break;
case "staging":
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(baseTimeout + 5));
break;
case "production":
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(baseTimeout + 10));
break;
}
}
}
Framework Integration
When building automation frameworks, consider creating wrapper methods that handle implicit wait configuration:
public class WebDriverManager {
private WebDriver driver;
public WebDriver initializeDriver(String browser, int implicitWaitSeconds) {
driver = createDriver(browser);
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(implicitWaitSeconds));
return driver;
}
private WebDriver createDriver(String browser) {
// Driver creation logic
return new ChromeDriver();
}
}
Frequently Asked Questions
What happens if I set both implicit and explicit waits?
When both implicit and explicit waits are set, Selenium will wait for the longer of the two timeouts. This can lead to unexpectedly long wait times, so it's generally recommended to use one approach consistently or understand the interaction between them.
Can I change the implicit wait timeout during test execution?
Yes, you can modify the implicit wait timeout at any time during test execution by calling the implicitlyWait method again with a new timeout value. The new setting will apply to all subsequent element lookups.
Does implicit wait affect page load times?
No, implicit wait only affects element lookup operations. It doesn't influence how long Selenium waits for pages to load completely. For page load timeouts, you need to use the pageLoadTimeout configuration.
Why does my test still fail even with an implicit wait set?
Implicit wait only helps with element lookup operations. If elements exist but aren't in the expected state (like being clickable or visible), you may need explicit waits with specific conditions.
What's the maximum implicit wait timeout I should use?
While there's no hard limit, timeouts longer than 30 seconds are generally not recommended as they can significantly slow down test execution, especially when elements genuinely don't exist.
Does implicit wait work with all locator strategies?
Yes, implicit wait works with all Selenium locator strategies, including ID, class name, XPath, CSS selectors, and others. The wait mechanism is applied at the WebDriver level, regardless of the locator method used.
Can I disable implicit wait once it's set?
Yes, you can effectively disable implicit wait by setting it to zero: driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(0)). This will make element lookups fail immediately if elements aren't found.
How does implicit wait behave with dynamic content?
Implicit wait is particularly effective with dynamic content that loads after page initialization. It will continuously poll the DOM until the element appears or the timeout is reached, making it ideal for AJAX-loaded content.
Conclusion
Implicit wait in Selenium is an essential tool for building robust, reliable automation tests. By providing a global safety net for element lookup operations, it helps address one of the most common causes of test flakiness: timing issues with dynamic web content.
The key to successful implementation lies in understanding your application's behavior, choosing appropriate timeout values, and integrating implicit wait thoughtfully into your overall automation strategy. While it's not a silver bullet for all timing issues, when used correctly, implicit wait can significantly improve the stability and maintainability of your test suite.
Remember that implicit wait works best as part of a comprehensive wait strategy that may also include explicit waits for specific scenarios. By combining these approaches thoughtfully, you can create automation frameworks that are both reliable and efficient.
As web applications continue to become more dynamic and complex, mastering wait strategies like implicit wait becomes increasingly important for successful test automation. The investment in understanding and properly implementing these techniques will pay dividends in terms of test reliability, maintenance overhead, and overall automation success.
Key Takeaways
• Implicit wait provides a global timeout setting that applies to all element lookup operations throughout your test session
• Proper timeout configuration is crucial - typically 10-30 seconds, depending on your application and environment characteristics
• Implicit wait works by continuously polling the DOM until elements appear or the timeout period expires
• It's most effective for handling dynamic content that loads after initial page rendering, including AJAX-generated elements
• Combining implicit and explicit waits requires careful consideration to avoid unexpectedly long wait times
• Framework integration should include dynamic timeout adjustment based on environment and application characteristics
• Monitoring and optimization are essential to balance test reliability with execution speed
• Troubleshooting timing issues requires systematic debugging, including logging, DOM inspection, and environment testing
• Modern Selenium versions offer more granular timeout control with separate settings for different types of waits
Comments