Guide to 8080 Localhost: Master Local Development
- Gunashree RS
- Jul 6, 2024
- 5 min read
Updated: Aug 13, 2024
Introduction
In web development, having a reliable local development server is crucial. Ports like localhost:3000 and localhost:5000 are popular choices, but they can become busy with multiple applications. This is where localhost:8080 comes into play. By using port 8080, developers can run additional services and ensure smooth testing and debugging processes.
This guide will walk you through everything you need to know about localhost:8080. We'll cover how to set up and start servers using different technologies, troubleshoot common issues, and provide practical examples. Whether you're a novice or an experienced developer, this article will help you effectively utilize localhost:8080 in your development workflow.
Understanding Localhost and Port 8080
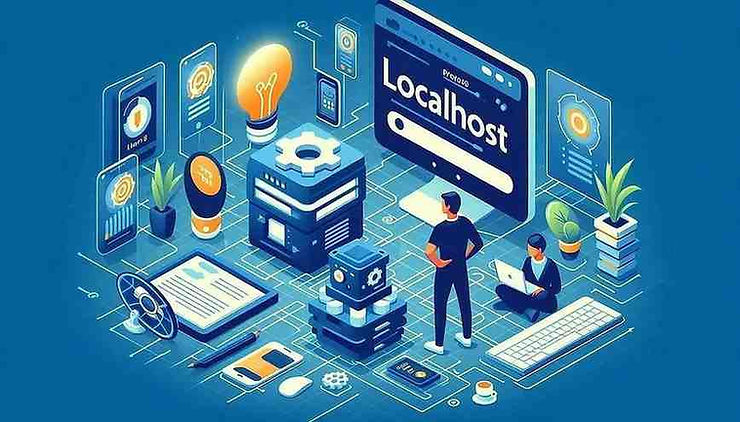
What is Localhost?
Localhost is a hostname that points to the current machine being used. It is commonly used for testing and development purposes, allowing developers to run web applications locally before deploying them to production environments.
What is Port 8080?
Ports are virtual endpoints used for network communications on a device. Port 8080 is a commonly used alternative to the default port 80 (HTTP) and 443 (HTTPS) for web servers. It is especially useful when ports like 3000 or 5000 are occupied.
Benefits of Using 8080 Localhost
Using localhost:8080 offers several advantages:
Avoiding Port Conflicts: It serves as an alternative when other commonly used ports are busy.
Flexibility: Many frameworks and tools allow easy configuration to switch ports.
Standardization: It is widely recognized and used in various development environments.
How to Start the Localhost:8080 Server
To start a server on localhost:8080, you need to use a service that can listen on this port. Below are the instructions for different frameworks and tools.
Node.js
Node.js is a JavaScript runtime commonly used for building server-side applications. Here’s how to start a Node.js server on port 8080.
Install Server Tools:
bash
npm install -g http-server live-server |
Navigate to Your Project Directory:
bash
cd pathToYourProject |
Start the Server:
bash
http-server -p 8080 # or live-server --port=8080 |
React
React is a popular library for building user interfaces. The create-react-app tool includes a built-in development server.
Create a React App:
bash
npx create-react-app my-app |
Navigate to Your Project Directory:
bash
cd my-app |
Start the Development Server on Port 8080:
bash
$env:PORT=8080 ; npm start |
Angular
Angular is a platform for building mobile and desktop web applications. It uses the Angular CLI for development.
Create an Angular App:
bash
ng new my-app |
Navigate to Your Project Directory:
bash
cd my-app |
Start the Development Server on Port 8080:
bash
ng serve --port 8080 |
Vue.js
Vue.js is a progressive JavaScript framework for building user interfaces.
Create a Vue App:
bash
vue create my-app |
Navigate to Your Project Directory:
bash
cd my-app |
Start the Development Server on Port 8080:
bash
npm run serve -- --port 8080 |
Creating a Development Server in React
To create and start a development server in React on port 8080, follow these steps:
Step 1: Create a React App
Use create-react-app to set up a new React project.
bash
npx create-react-app my-app |
Step 2: Navigate to Your Project Directory
Change into your project directory.
bash
cd my-app |
Step 3: Start the Development Server on Port 8080
Run the following command to start the server on port 8080.
bash
$env:PORT=8080 ; npm start |
Example React Application
Below is a simple example of a React component.
javascript
// App.js import React, { useState } from 'react'; const App = () => { const [isHidden, setIsHidden] = useState(false); const toggleContent = () => { setIsHidden(!isHidden); }; return ( <div style={{ textAlign: 'center' }}> <h1 style={{ color: 'green' }}>GeeksforGeeks</h1> <h2>Welcome to GeeksforGeeks</h2> {isHidden && <h3>A Computer Science Portal</h3>} <button onClick={toggleContent} style={{ backgroundColor: 'green', color: '#fff', padding: '15px', cursor: 'pointer', border: 'none', borderRadius: '10px', }} > Toggle More Content </button> {isHidden && <p>This content is toggled dynamically using a button click event.</p>} </div> ); }; export default App; |
Troubleshooting Localhost 8080
Port Already in Use
If localhost:8080 is already in use, you can either stop the process of occupying the port or change the port for your server.
Find and Kill the Process:
bash
lsof -i :8080 kill -9 <PID> |
Change the Port: Modify your server start command to use a different port, such as 8081.
Access Issues
If you can't access localhost:8080, ensure your firewall or antivirus software is not blocking the port. Additionally, check your network settings to ensure there are no restrictions on localhost connections.
Server Not Starting
Ensure all dependencies are installed correctly. Run npm install to install missing packages and check for errors in your terminal.
Key Takeaway:
Localhost:8080 serves as a crucial alternative port for web developers when common ports like 3000 or 5000 are occupied, offering flexibility and reliability.
Setting up a development server on localhost:8080 involves specific commands tailored to frameworks like Node.js, React, Angular, and Vue.js.
Troubleshooting tips include handling port conflicts, resolving access issues, and ensuring server dependencies are correctly installed.
Understanding localhost and port 8080 facilitates efficient local testing and debugging before deploying applications to production environments.
Security considerations ensure that while localhost itself is secure, developers must configure their servers to protect sensitive data appropriately.
Conclusion
Localhost:8080 is an essential tool for web developers, providing a flexible and reliable environment for testing and previewing applications locally. Whether you're working with React, Node.js, Angular, or another framework, understanding how to start and manage a local server on localhost:8080 is crucial.
This guide has covered the basics of what localhost:8080 is, how to start a server using various frameworks, troubleshooting tips, and common FAQs. By mastering these concepts, you can streamline your development workflow and ensure your applications run smoothly in a local environment.
FAQs
What is localhost:8080?
Localhost:8080 is the address used to access a local development server running on port 8080. It is commonly used when other ports like 3000 or 5000 are occupied.
How do I start a server on localhost:8080?
You can start a server on localhost:8080 using various commands depending on the framework you are using. For example, http-server -p 8080 for Node.js, $env:PORT=8080 ; npm start for React applications, and ng serve --port 8080 for Angular.
What if port 8080 is already in use?
If port 8080 is already in use, you can either stop the process using the port or start your server on a different port. Use commands like lsof -i :8080 to find the process ID and kill -9 <PID> to stop it.
Why can't I access localhost:8080?
Access issues can be due to firewall or antivirus settings blocking the port. Ensure that your local network settings allow connections to localhost and that no other applications are conflicting with port 8080.
Can I change the default port from 8080 to another port?
Yes, you can change the default port by modifying the server start command. For example, in React, you can set a different port by running $env:PORT=8081 ; npm start
.
Is localhost:8080 secure?
Localhost itself is secure since it refers to the local machine. However, ensure that the development server running on this port does not expose sensitive data and is configured correctly.
Comments