Binary to Gray Code Conversion: Guide to Error-Minimizing Code | 2025
- Gunashree RS
- 21 hours ago
- 7 min read
Introduction to Binary and Gray Code Systems
In the world of digital electronics and computing, different numbering systems serve various purposes. Among these, binary code is the fundamental language of computers, while Gray code offers unique advantages in specific applications. Understanding binary to gray code conversion is essential for engineers, programmers, and students working in fields like digital signal processing, rotary encoders, and error correction systems.
Binary code uses a base-2 number system with only two digits (0 and 1) to represent all values. It's the foundation of modern computing, directly mapping to the on/off states of electronic circuits. However, binary code has a significant drawback: when transitioning between consecutive numbers, multiple bits often change simultaneously. For example, when moving from binary 7 (0111) to 8 (1000), all four bits change state.
Gray code (also known as reflected binary code) addresses this limitation by ensuring that only one bit changes between adjacent numbers. This property, called unit distance, makes the Gray code particularly valuable in applications where errors during transitions must be minimized.
Let's explore the fascinating world of binary to gray code conversion, its algorithms, implementations, and real-world applications.
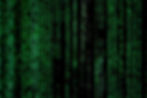
Understanding the Binary to Gray Code Conversion Algorithm
The process of converting binary to Gray code follows a surprisingly simple algorithm. Given a binary number, we can derive its Gray code equivalent through these steps:
The most significant bit (MSB) of the Gray code is identical to the MSB of the binary number
Each subsequent bit in the Gray code is determined by XORing adjacent bits in the binary number
This can be expressed mathematically as:
G₁ = B₁ G₂ = B₁ ⊕ B₂ G₃ = B₂ ⊕ B₃ ...and so on
Where:
G₁, G₂, G₃... are bits of the Gray code (G₁ being the MSB)
B₁, B₂, B₃... are bits of the binary number (B₁ being the MSB)
⊕ represents the XOR (exclusive OR) operation
Let's walk through an example to illustrate this conversion process:
Example: Converting Binary 1011 to Gray Code
Step | Description | Calculation | Result |
1 | MSB of Gray code = MSB of binary | G₁ = B₁ = 1 | G₁ = 1 |
2 | Second bit of Gray code | G₂ = B₁ ⊕ B₂ = 1 ⊕ 0 = 1 | G₂ = 1 |
3 | The third bit of Gray code | G₃ = B₂ ⊕ B₃ = 0 ⊕ 1 = 1 | G₃ = 1 |
4 | LSB of Gray code | G₄ = B₃ ⊕ B₄ = 1 ⊕ 1 = 0 | G₄ = 0 |
Therefore, the Gray code representation of binary 1011 is 1110.
Alternatively, a more concise way to express this conversion is:
Gray code = Binary number ⊕ (Binary number shifted right by 1 position)
For our example:
Binary number: 1011
Binary shifted right: 0101
XOR result: 1110 (Gray code)
This XOR-based method is both elegant and computationally efficient, making it the preferred approach for binary to Gray code conversion in most applications.
Implementing Binary to Gray Code Conversion in Programming Languages
Converting binary to Gray code can be implemented efficiently in various programming languages. Here are implementations in some popular languages:
Python Implementation
def binary_to_gray(binary):
"Convert a binary number to its Gray code equivalent."
if not all(bit in '01' for bit in binary):
raise ValueError("Input must be a binary number (containing only 0s and 1s)")
# Convert binary string to integer
binary_int = int(binary, 2)
# Apply the formula: gray = binary XOR (binary >> 1)
gray_int = binary_int ^ (binary_int >> 1)
# Convert back to binary string with the same length as input
gray_code = bin(gray_int)[2:].zfill(len(binary))
return gray_code
# Example usage
binary_num = "1011"
gray_code = binary_to_gray(binary_num)
print(f"Binary: {binary_num} → Gray Code: {gray_code}")
# Output: Binary: 1011 → Gray Code: 1110
Java Implementation
public class BinaryToGrayConverter {
public static String binaryToGray(String binary) {
// Validate input
if (!binary.matches("[01]+")) {
throw new IllegalArgumentException("Input must be a binary number");
}
// Convert binary string to integer
int binaryInt = Integer.parseInt(binary, 2);
// Apply the formula: gray = binary XOR (binary >> 1)
int grayInt = binaryInt ^ (binaryInt >> 1);
// Convert back to binary string
String grayCode = Integer.toBinaryString(grayInt);
// Pad with leading zeros if necessary
while (grayCode.length() < binary.length()) {
grayCode = "0" + grayCode;
}
return grayCode;
}
public static void main(String[] args) {
String binaryNum = "1011";
String grayCode = binaryToGray(binaryNum);
System.out.println("Binary: " + binaryNum + " → Gray Code: " + grayCode);
// Output: Binary: 1011 → Gray Code: 1110
}
}
C++ Implementation
# include <iostream>
# include <string>
# include <bitset>
std::string binaryToGray(const std::string& binary) {
// Validate input
for (char bit : binary) {
if (bit != '0' && bit != '1') {
throw std::invalid_argument("Input must be a binary number");
}
}
// Convert binary string to integer
unsigned long binaryInt = std::stoul(binary, nullptr, 2);
// Apply the formula: gray = binary XOR (binary >> 1)
unsigned long grayInt = binaryInt ^ (binaryInt >> 1);
// Convert back to binary string with the same length as input
std::string grayCode = std::bitset<32>(grayInt).to_string();
// Remove leading zeros and ensure the result has the same length as the input
grayCode = grayCode.substr(32 - binary.length());
return grayCode;
}
int main() {
std::string binaryNum = "1011";
std::string grayCode = binaryToGray(binaryNum);
std::cout << "Binary: " << binaryNum << " → Gray Code: " << grayCode << std::endl;
// Output: Binary: 1011 → Gray Code: 1110
return 0;
}
These implementations demonstrate the straightforward nature of the binary to Gray code conversion algorithm across different programming languages. The XOR operation (^) is universally available, making this conversion highly portable.
Applications of Binary to Gray Code Conversion
The unique property of the Gray code—changing only one bit between consecutive numbers—makes it invaluable in various applications:
1. Rotary Encoders and Position Sensors
Rotary encoders are electromechanical devices that convert angular position to digital code. Using Gray code in these devices minimizes errors during position reading because if a transition occurs during reading, at most one bit will be incorrect.
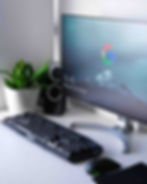
2. Error Correction in Digital Communications
When transmitting digital signals, Gray code helps reduce the impact of noise. If noise causes a value to shift to an adjacent value, only one bit will be affected, making error detection and correction more manageable.
3. Analog-to-Digital Converters (ADCs)
ADCs often use Gray code internally to minimize conversion errors. If a signal is at the boundary between two quantization levels, using Gray code ensures that the output will have at most one bit of error.
4. Genetic Algorithms and Optimization
In genetic algorithms, Gray coding is used to represent chromosomes because neighboring values have minimal differences. This property helps in more effective mutation and crossover operations.
5. Digital Circuit Design
Gray code is used in state machines and sequential circuits to reduce glitches and hazards by ensuring that only one bit changes between consecutive states.
6. Puzzle Solving
The famous Tower of Hanoi puzzle and some variants of the Chinese ring puzzle can be elegantly solved using Gray code sequences, demonstrating their application beyond pure engineering.
Binary to Gray Code Conversion Tools and Resources
Several online tools and resources are available to assist with binary to Gray code conversion:
Online Converters:
LambdaTest's Binary to Gray Code Converter offers a simple interface for quick conversions.
Digital Electronics calculators that include this conversion, among other utilities
Mobile Apps:
Engineering calculators and digital electronics toolkits often include binary-to-Gray code conversion features.
Software Libraries:
Most programming languages have libraries or packages that implement various coding scheme conversions, including binary to Gray.
Hardware-based Converters:
IC chips designed specifically for encoding and decoding Gray code in electronic applications
These tools make binary-to-Gray code conversion accessible for both educational and professional use cases.
Conclusion
Binary to Gray code conversion represents an elegant solution to a significant problem in digital systems: minimizing errors during transitions between consecutive values. The simple XOR-based algorithm enables efficient implementation across various programming languages and hardware platforms.
From rotary encoders to genetic algorithms, Gray code's unique property of changing only one bit between adjacent values continues to find relevant applications in modern technology. Understanding this conversion process is valuable for students, engineers, and anyone working with digital systems.
As digital technologies continue to evolve, the principles behind Gray code remain relevant, demonstrating how fundamental mathematical concepts can solve practical engineering challenges.
Key Takeaways
Binary to Gray code conversion uses a simple XOR operation: Gray = Binary XOR (Binary >> 1)
Gray code ensures only one bit changes between consecutive numbers, reducing transition errors.
The most significant bit of Gray code is always identical to the most significant bit of binary code.
The conversion algorithm is computationally efficient and easily implemented in any programming language.
Key applications include rotary encoders, error correction systems, and analog-to-digital converters.
Understanding Gray code helps in designing more robust digital systems and communication protocols.
Online tools like LambdaTest's Binary to Gray converter provide convenient conversion services.
Gray code demonstrates how mathematical principles can elegantly solve practical engineering problems.
FAQ: Common Questions About Binary to Gray Code Conversion
Q: What is the main advantage of Gray code over binary code?
A: The main advantage of Gray code is that only one bit changes between consecutive code values, which significantly reduces the risk of errors during transitions in digital systems.
Q: Is gray code more efficient than binary code for computer operations?
A: No, Gray code is not more efficient for general computer operations. Binary is still the standard for computation because it directly maps to the logical operations in computer hardware. Gray code is specifically useful in applications where transitional errors need to be minimized.
Q: How do I convert from Gray code back to binary?
A: To convert Gray code back to binary, you use a different algorithm: the first bit of binary is the same as the first bit of Gray code, and each subsequent binary bit is the XOR of the corresponding Gray bit and the previous binary bit.
Q: Can Gray code be used for arithmetic operations?
A: Gray code is not directly suitable for arithmetic operations. It's designed for minimizing transition errors, not for computation. For arithmetic, you would typically convert Gray code to binary, perform the calculations, and then convert back if needed.
Q: Does Gray code work with any number of bits?
A: Yes, Gray code can be generated for any bit length. The conversion algorithm works regardless of the number of bits in the input binary number.
Q: Are there variations of the Gray code for specific applications?
A: Yes, there are several variations of Gray code optimized for specific applications, including balanced Gray codes, monotonic Gray codes, and n-ary Gray codes for non-binary applications.
Sources and Further Reading
Binary to Gray Code Converter by LambdaTest: https://www.lambdatest.com/free-online-tools/binary-to-gray
IEEE Transactions on Computers - Applications of Gray Codes: https://ieeexplore.ieee.org/xpl/RecentIssue.jsp?punumber=12
Computer Organization and Design by David A. Patterson and John L. Hennessy: https://www.elsevier.com/books/computer-organization-and-design-mips-edition/patterson/978-0-12-407726-3
Journal of Digital Signal Processing - Error Correction Using Gray Codes: https://www.journals.elsevier.com/digital-signal-processing
The Art of Computer Programming, Volume 4A: Combinatorial Algorithms, Part 1 by Donald E. Knuth: https://www-cs-faculty.stanford.edu/~knuth/taocp.html
Applied Cryptography and Network Security - Error Minimization Techniques: https://link.springer.com/conference/acns