CSS Scrolling: Master Smooth and Responsive Scrolling Effects
- Gunashree RS
- Aug 27, 2024
- 6 min read
In the ever-evolving world of web development, user experience (UX) has become a paramount focus. One of the most significant aspects of UX is how users interact with a webpage, particularly how they scroll through content. Scrolling is a fundamental interaction on the web, and the way it is handled can make or break the user’s experience.
While traditional scrolling is the default for most websites, smooth scrolling has gained popularity for its ability to create a more fluid and visually appealing experience. This guide delves deep into the various methods of implementing CSS scrolling, including the use of CSS, JavaScript, and jQuery to achieve smooth scrolling effects that work across different browsers.
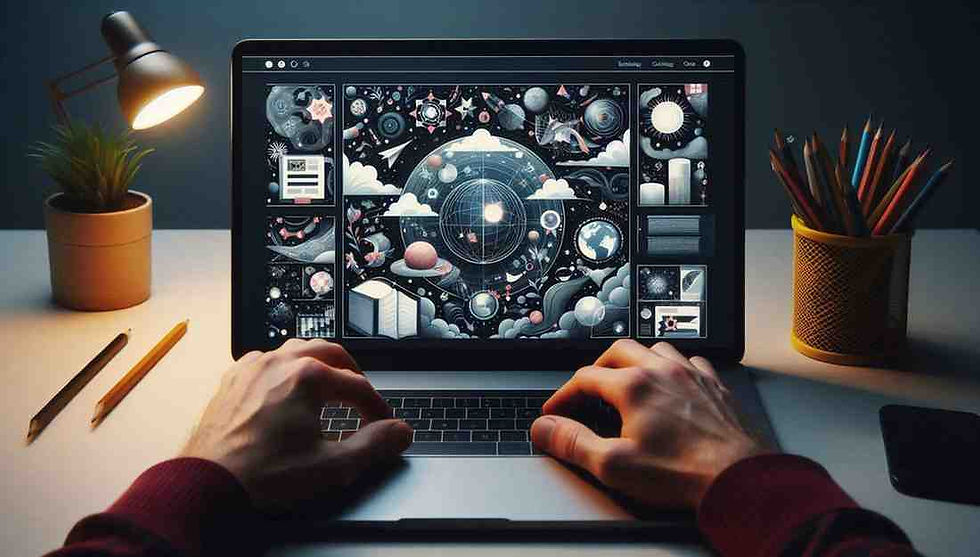
1. Introduction to CSS Scrolling
1.1 What is Scrolling in Web Design?
Scrolling refers to the action of moving vertically or horizontally through content on a webpage. It is a fundamental interaction that allows users to access different parts of a website without navigating away from the current page. Scrolling can be triggered by various means, such as mouse wheels, keyboard keys, touch gestures, or on-screen buttons.
1.2 The Importance of Smooth Scrolling
Smooth scrolling enhances the user experience by providing a more fluid transition between sections of a webpage. Unlike traditional scrolling, which can feel abrupt and jarring, smooth scrolling eases the visual transition, making the movement more natural and less disorienting. This is particularly important for content-heavy sites, where users are likely to scroll frequently.
1.3 Traditional vs. Smooth Scrolling
Traditional scrolling typically results in an instant jump from one section of the page to another. This can be disorienting, especially on long pages. Smooth scrolling, on the other hand, creates a gradual movement, allowing users to better follow the content as they move through it. This guide will explore how to implement smooth scrolling using CSS, JavaScript, and jQuery.
2. Setting Up a Web Page for CSS Scrolling
2.1 Creating the HTML Structure
To demonstrate smooth scrolling, we first need to set up a simple HTML page structure. This will include multiple sections, each linked to navigation items.
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Scrolling Demo</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<nav>
<a href="#home">Home</a>
<a href="#section1">Section 1</a>
<a href="#section2">Section 2</a>
</nav>
</header>
<section id="home">HOME</section>
<section id="section1">SECTION 1</section>
<section id="section2">SECTION 2</section>
<script src="script.js"></script>
</body>
</html>
2.2 Adding CSS for Basic Layout
With the HTML structure in place, we’ll add CSS to style the sections and ensure they are full-screen height for a clear scrolling effect.
css
section {
min-height: 100vh;
display: flex;
align-items: center;
justify-content: center;
font-size: 3em;
color: 333;
font-family: 'Arial', sans-serif;
}
2.3 Implementing Basic Scrolling
Without any additional properties, clicking on the navigation links would result in a jump to the respective sections. We’ll explore how to transform this into smooth scrolling in the next sections.
3. Achieving Smooth Scrolling with CSS
3.1 The scroll-behavior Property
The CSS scroll-behavior property allows developers to implement smooth scrolling with minimal code. By applying this property to the HTML or body elements, all internal links will scroll smoothly.
css
html {
scroll-behavior: smooth;
}
3.2 Applying Smooth Scrolling to the Entire Document
By adding scroll-behavior: smooth to the html element, you ensure that all anchor links within the document will trigger a smooth scroll effect. This method is simple and effective for basic needs.
css
html {
scroll-behavior: smooth;
}
3.3 Browser Compatibility for CSS Scrolling
While the scroll-behavior property is supported by most modern browsers, it is not universally compatible, particularly with older versions of Internet Explorer and some mobile browsers like Opera Mini. Developers must ensure fallback mechanisms or alternative methods for smooth scrolling on unsupported browsers.
Browser Compatibility Overview:
Supported: Chrome, Firefox, Safari, Edge
Not Supported: Internet Explorer, Opera Mini, KaiOS
4. JavaScript for Advanced Scrolling Effects
4.1 Why Use JavaScript for Scrolling?
JavaScript offers greater control over scrolling behavior than CSS alone. With JavaScript, developers can customize the scroll speed, direction, delay, and even trigger additional animations during the scroll. This flexibility makes JavaScript a preferred choice for more complex scrolling needs.
4.2 Implementing Smooth Scrolling with Vanilla JavaScript
Vanilla JavaScript provides a straightforward way to implement smooth scrolling. By adding an event listener to navigation links and using the scrollIntoView method, you can create a custom smooth scrolling effect.
javascript
document.querySelectorAll('nav a').forEach(anchor => {
anchor.addEventListener('click', function(e) {
e.preventDefault();
document.querySelector(this.getAttribute('href')).scrollIntoView({
behavior: 'smooth'
});
});
});
4.3 Controlling Scroll Speed and Behavior
JavaScript also allows you to fine-tune the scroll behavior by manipulating the scrollTo or scrollBy methods. This control can be particularly useful for creating a unique scrolling experience tailored to your website's design.
javascript
window.scrollTo({
top: 500,
behavior: 'smooth'
});
5. Using jQuery for Enhanced Scrolling Control
5.1 Advantages of jQuery Over Vanilla JavaScript
While Vanilla JavaScript is powerful, jQuery simplifies the process of implementing smooth scrolling with fewer lines of code. jQuery is widely supported and includes methods like animate that offer additional customization options.
5.2 Implementing Smooth Scrolling with jQuery
With jQuery, implementing smooth scrolling is as simple as attaching an event to the navigation links and using the animate method to control the scroll behavior.
javascript
$("nav a").on("click", function(e) {
e.preventDefault();
$("html, body").animate({
scrollTop: $($(this).attr("href")).offset().top
}, 1000);
});
5.3 Customizing Scrolling Duration and Delay
One of the key advantages of using jQuery is the ability to easily customize the scroll duration and delay. This can enhance the user experience by making the scroll speed more appropriate for your specific content.
javascript
$("nav a").on("click", function(e) {
e.preventDefault();
const target = $(this).attr("href");
$("html, body").animate({
scrollTop: $(target).offset().top
}, 800); // Duration in milliseconds
});
6. Cross-Browser Compatibility Testing
6.1 Why Cross-Browser Testing is Crucial
Even the most well-designed scrolling effects can fail if they are not compatible across all browsers. Cross-browser testing ensures that your scrolling effects are consistent and reliable, regardless of the user's browser or device.
6.2 Tools for Cross-Browser Testing
Several tools can help you test your scrolling effects across multiple browsers and devices. BrowserStack, for instance, offers real device testing, enabling you to verify how your site performs under real-world conditions.
6.3 Ensuring Consistent Scrolling Across Devices
To deliver a seamless user experience, it is crucial to ensure that smooth scrolling behaves consistently across various devices, including desktops, tablets, and smartphones. Test on multiple screen sizes and resolutions to catch any discrepancies in scrolling behavior.
7. Best Practices for Implementing CSS Scrolling
7.1 Keeping User Experience in Mind
Smooth scrolling should enhance the user experience, not detract from it. Consider your audience and the content of your site when deciding on scroll speed and behavior. Avoid making the scroll too slow or too fast, as this can frustrate users.
7.2 Optimizing for Performance
Smooth scrolling can have an impact on performance, especially on resource-constrained devices. Optimize your implementation by minimizing unnecessary scripts and ensuring that your JavaScript is efficiently written.
7.3 Avoiding Common Pitfalls
Common pitfalls include overusing smooth scrolling or implementing it on pages where it’s not necessary. Not every section needs smooth scrolling, and in some cases, it can feel unnatural. Use smooth scrolling judiciously to enhance user experience without overwhelming it.
8. Conclusion
Smooth scrolling is a powerful tool in web design that can significantly enhance user experience by creating more fluid transitions between sections of a webpage. Whether you opt for a simple CSS implementation or a more complex JavaScript approach, the key is to ensure that your scrolling effects are consistent, perform well, and are compatible across all browsers and devices. By following the guidelines and best practices outlined in this article, you can implement smooth scrolling on your website effectively and with confidence.
9. FAQs
Q1: What is smooth scrolling in CSS?
A1: Smooth scrolling in CSS is achieved using the scroll-behavior property, which allows for a gradual, smooth transition between elements on a webpage.
Q2: How do I implement smooth scrolling using CSS?
A2: Add scroll-behavior: smooth; to the html or body element in your CSS file. This will enable smooth scrolling for anchor links within your document.
Q3: Why should I use JavaScript for smooth scrolling?
A3: JavaScript offers greater control over scrolling behavior, including customization of scroll speed, duration, and additional animations.
Q4: Is smooth scrolling compatible with all browsers?
A4: While most modern browsers support CSS smooth scrolling, older browsers like Internet Explorer and mobile browsers like Opera Mini may not. JavaScript provides a more universally compatible solution.
Q5: What is the advantage of using jQuery for smooth scrolling?
A5: jQuery simplifies the implementation of smooth scrolling with fewer lines of code and provides more control over the scrolling effect, such as customizing scroll speed and delay.
Q6: How can I ensure my smooth scrolling works across all devices?
A6: Perform cross-browser testing using tools like BrowserStack to verify that smooth scrolling behaves consistently across different browsers and devices.
10. Key Takeaways
Smooth scrolling enhances user experience by providing fluid transitions between webpage sections.
CSS scroll behavior is a simple, one-line solution but lacks broad browser support.
JavaScript offers more control and compatibility for smooth scrolling effects.
jQuery is a powerful option for implementing smooth scrolling with additional customization.
Cross-browser testing is essential to ensure consistent behavior across all devices.
Comments