C# Web API Interview Questions: Guide for Developers 2025
- Gunashree RS
- 21 hours ago
- 8 min read
Preparing for a C# Web API interview can feel overwhelming, especially when you're unsure what questions to expect. Whether you're a junior developer starting your career or an experienced professional aiming for a senior position, having the right answers ready can make all the difference in landing your dream job.
This comprehensive guide covers the most commonly asked C# Web API interview questions, from basic concepts to advanced architectural patterns. Each question includes detailed explanations and practical examples to help you understand not just the "what" but also the "why" behind each concept.
The demand for skilled C# Web API developers continues to grow as businesses increasingly rely on robust, scalable web services. Understanding these interview questions and their answers will give you the confidence and knowledge needed to excel in your next technical interview.
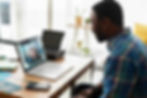
Basic C# Web API Interview Questions
Q1: What is a Web API, and how does it differ from a web application?
A Web API (Application Programming Interface) is a set of protocols and tools that allow different software applications to communicate with each other over HTTP. Unlike traditional web applications that return HTML pages for human consumption, Web APIs return data (typically in JSON or XML format) that can be consumed by other applications, mobile apps, or frontend frameworks.
The key differences include:
Web APIs return data, not HTML views
They're designed for machine-to-machine communication
They follow REST principles for resource manipulation
They use HTTP methods (GET, POST, PUT, DELETE) to perform operations
They're stateless and don't maintain session information
Q2: What are the main HTTP methods used in RESTful APIs, and when do you use each?
The primary HTTP methods used in RESTful APIs are:
GET: Retrieves data from the server. Used for reading operations and should be idempotent (multiple calls produce the same result)
POST: Creates new resources on the server. Not idempotent, as each call creates a new resource
PUT: Updates entire resources or creates them if they don't exist. Idempotent operation
PATCH: Partially updates existing resources. Used when you only need to modify specific fields
DELETE: Removes resources from the server. Idempotent operation
HEAD: Similar to GET but returns only headers, useful for checking resource existence
OPTIONS: Returns available HTTP methods for a resource, commonly used in CORS preflight requests
Q3: What is the difference between Web API and WCF?
Web API and WCF (Windows Communication Foundation) serve different purposes:
Web API:
Lightweight and designed for HTTP-based services
Supports only the HTTP protocol
Uses standard HTTP verbs and status codes
Returns data in JSON, XML, or other formats
Better for RESTful services and mobile applications
Easier to test and debug
WCF:
More comprehensive communication framework
Supports multiple protocols (HTTP, TCP, Named Pipes, MSMQ)
More complex configuration and setup
Better for enterprise applications requiring advanced features
Supports duplex communication and reliable messaging
Intermediate C# Web API Interview Questions
Q4: How do you implement authentication and authorization in a Web API?
Authentication and authorization in Web API can be implemented through several approaches:
JWT (JSON Web Token) Authentication:
// Configure JWT in Startup.cs
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = "your-issuer",
ValidAudience = "your-audience",
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your-secret-key"))
};
});
Authorization Attributes:
Use [Authorize] attribute on controllers or actions
Implement role-based authorization with [Authorize(Roles = "Admin")]
Create custom authorization policies for complex scenarios
Q5: What are Action Filters, and how do you create custom ones?
Action Filters are attributes that add extra processing logic before or after action method execution. They implement the IActionFilter interface and are useful for cross-cutting concerns like logging, caching, and validation.
Creating a Custom Action Filter:
public class LogActionFilter : ActionFilterAttribute
{
public override void OnActionExecuting(ActionExecutingContext context)
{
// Logic before action execution
var actionName = context.ActionDescriptor.DisplayName;
Console.WriteLine($"Executing action: {actionName}");
}
public override void OnActionExecuted(ActionExecutedContext context)
{
// Logic after action execution
Console.WriteLine("Action completed");
}
}
Usage:
[LogActionFilter]
public class ProductsController : ControllerBase
{
[HttpGet]
public IActionResult GetProducts()
{
return Ok(products);
}
}
Q6: How do you handle exceptions globally in Web API?
Global exception handling ensures consistent error responses and proper logging throughout your application:
Using Exception Middleware:
public class ExceptionHandlingMiddleware
{
private readonly RequestDelegate _next;
private readonly ILogger<ExceptionHandlingMiddleware> _logger;
public ExceptionHandlingMiddleware(RequestDelegate next, ILogger<ExceptionHandlingMiddleware> logger)
{
_next = next;
_logger = logger;
}
public async Task InvokeAsync(HttpContext context)
{
try
{
await _next(context);
}
catch (Exception ex)
{
_logger.LogError(ex, "An unhandled exception occurred");
await HandleExceptionAsync(context, ex);
}
}
private static async Task HandleExceptionAsync(HttpContext context, Exception exception)
{
context.Response.ContentType = "application/json";
var response = new
{
error = new
{
message = "An error occurred while processing your request",
details = exception.Message
}
};
context.Response.StatusCode = exception switch
{
NotFoundException => 404,
ValidationException => 400,
UnauthorizedException => 401,
_ => 500
};
await context.Response.WriteAsync(JsonSerializer.Serialize(response));
}
}
Advanced C# Web API Interview Questions
Q7: How do you implement API versioning, and what are the different strategies?
API versioning is crucial for maintaining backward compatibility while evolving your API. Here are the main strategies:
URL Path Versioning:
[Route("api/v1/[controller]")]
public class ProductsV1Controller : ControllerBase { }
[Route("api/v2/[controller]")]
public class ProductsV2Controller : ControllerBase { }
Query String Versioning:
[HttpGet]
public IActionResult GetProducts([FromQuery] string version = "1.0")
{
if (version == "2.0")
return Ok(GetV2Products());
return Ok(GetV1Products());
}
Header Versioning:
services.AddApiVersioning(options =>
{
options.ApiVersionReader = new HeaderApiVersionReader("X-Version");
options.DefaultApiVersion = new ApiVersion(1, 0);
options.AssumeDefaultVersionWhenUnspecified = true;
});
Q8: What is dependency injection, and how is it implemented in Web API?
Dependency Injection (DI) is a design pattern that provides dependencies to a class rather than having the class create them directly. This promotes loose coupling, testability, and maintainability.
Built-in DI Container Configuration:
// In Startup.cs ConfigureServices method
services.AddScoped<IProductService, ProductService>();
services.AddTransient<IEmailService, EmailService>();
services.AddSingleton<IConfiguration>(Configuration);
Service Lifetimes:
Transient: New instance created each time it's requested
Scoped: One instance per HTTP request
Singleton: Single instance for the application lifetime
Controller Injection:
public class ProductsController : ControllerBase
{
private readonly IProductService _productService;
public ProductsController(IProductService productService)
{
_productService = productService;
}
[HttpGet]
public async Task<IActionResult> GetProducts()
{
var products = await _productService.GetAllProductsAsync();
return Ok(products);
}
}
Q9: How do you implement caching in Web API?
Caching improves performance by storing frequently accessed data in memory:
Response Caching:
[ResponseCache(Duration = 300)] // Cache for 5 minutes
[HttpGet]
public IActionResult GetProducts()
{
return Ok(products);
}
In-Memory Caching:
public class ProductService : IProductService
{
private readonly IMemoryCache _cache;
public ProductService(IMemoryCache cache)
{
_cache = cache;
}
public async Task<List<Product>> GetProductsAsync()
{
if (!_cache.TryGetValue("products", out List<Product> products))
{
products = await GetProductsFromDatabase();
_cache.Set("products", products, TimeSpan.FromMinutes(30));
}
return products;
}
}
Distributed Caching with Redis:
services.AddStackExchangeRedisCache(options =>
{
options.Configuration = "localhost:6379";
});
Performance and Scalability Questions
Q10: How do you optimize Web API performance?
Several strategies can significantly improve Web API performance:
Asynchronous Programming:
[HttpGet]
public async Task<IActionResult> GetProductsAsync()
{
var products = await _productService.GetAllProductsAsync();
return Ok(products);
}
Pagination:
[HttpGet]
public async Task<IActionResult> GetProducts([FromQuery] int page = 1, [FromQuery] int pageSize = 10)
{
var products = await _productService.GetProductsAsync(page, pageSize);
return Ok(new { Data = products, Page = page, PageSize = pageSize });
}
Compression:
services.AddResponseCompression(options =>
{
options.EnableForHttps = true;
options.Providers.Add<GzipCompressionProvider>();
});
Database Optimization:
Use appropriate indexes
Implement connection pooling
Use stored procedures for complex queries
Implement read replicas for read-heavy workloads
Q11: How do you handle file uploads in Web API?
File uploads require proper validation and storage handling:
[HttpPost("upload")]
public async Task<IActionResult> UploadFile(IFormFile file)
{
if (file == null || file.Length == 0)
return BadRequest("No file uploaded");
// Validate file type and size
var allowedTypes = new[] { ".jpg", ".png", ".pdf" };
var extension = Path.GetExtension(file.FileName).ToLowerInvariant();
if (!allowedTypes.Contains(extension))
return BadRequest("Invalid file type");
if (file.Length > 10 1024 1024) // 10MB limit
return BadRequest("File too large");
// Save file
var fileName = Guid.NewGuid().ToString() + extension;
var filePath = Path.Combine("uploads", fileName);
using (var stream = new FileStream(filePath, FileMode.Create))
{
await file.CopyToAsync(stream);
}
return Ok(new { FileName = fileName, Size = file.Length });
}
Security-Related Interview Questions
Q12: What are the common security vulnerabilities in Web APIs, and how do you prevent them?
Common vulnerabilities and prevention strategies:
SQL Injection:
Use parameterized queries or Entity Framework
Validate and sanitize input data
Implement proper error handling
Cross-Site Scripting (XSS):
Encode output data
Use Content Security Policy headers
Validate input on both client and server sides
Cross-Site Request Forgery (CSRF):
Implement anti-forgery tokens
Use SameSite cookie attributes
Validate referrer headers
API Rate Limiting:
services.AddMemoryCache();
services.Configure<IpRateLimitOptions>(Configuration.GetSection("IpRateLimiting"));
services.AddSingleton<IIpPolicyStore, MemoryCacheIpPolicyStore>();
services.AddSingleton<IRateLimitCounterStore, MemoryCacheRateLimitCounterStore>();
services.AddSingleton<IRateLimitConfiguration, RateLimitConfiguration>();
Frequently Asked Questions
Q: What's the difference between PUT and PATCH methods?
A: PUT replaces the entire resource with the provided data, while PATCH applies partial updates to specific fields. PUT is idempotent (multiple identical requests have the same effect), whereas PATCH may or may not be idempotent depending on the implementation.
Q: How do you test Web APIs?
A: Web APIs can be tested using unit tests (testing individual components), integration tests (testing API endpoints), and tools like Postman, Swagger UI, or automated testing frameworks like NUnit or xUnit for comprehensive test coverage.
Q: What is CORS, and why is it important?
A: CORS (Cross-Origin Resource Sharing) is a security feature that allows or restricts web applications running on one domain to access resources from another domain. It's important for security as it prevents unauthorized cross-origin requests while enabling legitimate cross-domain communication.
Q: How do you document Web APIs?
A: Use tools like Swagger/OpenAPI for automatic documentation generation, include XML comments in your code, provide clear examples of request/response formats, and maintain comprehensive API documentation that includes authentication requirements and error codes.
Q: What are DTOs, and why are they important?
A: Data Transfer Objects (DTOs) are simple objects used to transfer data between different layers of an application. They're important because they provide a contract for data exchange, help with versioning, reduce over-posting vulnerabilities, and separate internal models from external APIs.
Q: How do you handle database connections in Web API?
A: Use dependency injection to inject database contexts, implement the repository pattern for data access abstraction, use connection pooling for efficiency, and ensure proper disposal of database connections through using statements or dependency injection scopes.
Q: What is middleware in ASP.NET Core?
A: Middleware is software that sits between different parts of an application and handles requests and responses. It forms a pipeline where each component can process requests before passing them to the next component and can also process responses on their way back to the client.
Q: How do you implement logging in Web API?
A: Use the built-in ILogger interface with dependency injection, configure different log levels (Debug, Information, Warning, Error, Critical), implement structured logging with tools like Serilog, and consider centralized logging solutions for production environments.
Conclusion
Mastering these C# Web API interview questions requires both theoretical knowledge and practical experience. The key to success lies in understanding not just the answers but also the reasoning behind each concept and when to apply different approaches in real-world scenarios.
Remember that interviewers are looking for candidates who can think critically about problems, communicate technical concepts clearly, and demonstrate practical experience with the technologies they've used. Practice implementing these concepts in actual projects, and be prepared to discuss challenges you've faced and how you've solved them.
The landscape of web development continues to evolve, so staying current with new features, best practices, and industry trends will help you stand out as a candidate. Focus on building a solid foundation in these core concepts while also exploring advanced topics like microservices, cloud deployment, and DevOps practices.
Key Takeaways
• Master fundamental HTTP methods, RESTful principles, and proper API design patterns
• Understand authentication and authorization mechanisms, including JWT and OAuth implementations
• Practice implementing common features like file uploads, caching, and error handling
• Learn advanced topics including dependency injection, middleware, and performance optimization
• Understand security best practices and common vulnerability prevention techniques
• Develop strong problem-solving skills and be prepared to explain your reasoning
• Stay current with modern development practices and cloud deployment strategies
• Practice coding exercises and be ready to implement solutions during technical interviews
• Focus on understanding the "why" behind each concept, not just memorizing answers
• Build practical experience through projects that demonstrate your Web API development skills