Comprehensive Guide to Implementing Sortable Tables
- Gunashree RS
- Sep 26, 2024
- 7 min read
Sortable tables are an essential feature in many web applications, providing users with the ability to organize data in ways that suit their needs. Whether it's sorting a list of products, user data, or even scientific information, properly implemented sortable tables enhance user experience by making large datasets easier to navigate and understand.
In this guide, we will explore everything you need to know about sortable tables, including their implementation, testing, and why they are critical in today’s web applications. We will also introduce advanced testing methods such as visual testing with tools like Applitools Eyes and Playwright to ensure that your sortable tables work seamlessly across all conditions.
What Are Sortable Tables?
Sortable tables allow users to reorder data displayed in a tabular format by clicking on column headers. This functionality is particularly useful in applications with large datasets where users may need to sort data alphabetically, numerically, or by other metrics.
For example, imagine an e-commerce platform where users are browsing through products. A sortable table allows them to:
Sort by price (ascending or descending).
Organize items by availability.
View product ratings from highest to lowest.
The ability to dynamically reorder data gives users more control over how they view information and enhances the usability of your application.
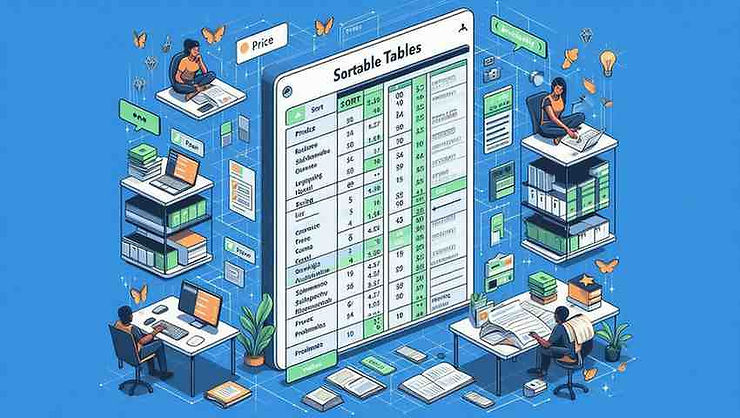
Why Are Sortable Tables Important?
Sortable tables are crucial for several reasons:
Enhanced User Experience: Users appreciate the ability to organize data in a way that is meaningful to them, increasing the usability of your application.
Efficient Data Management: Sortable tables make it easy to browse through large datasets, making it easier to find relevant information quickly.
Dynamic Content: Whether in finance, healthcare, e-commerce, or general analytics, sortable tables provide a dynamic way to interact with data.
Customization: Allowing users to sort data by their preference gives them more control over the displayed information, leading to increased engagement.
How to Implement Sortable Tables in HTML and JavaScript
Implementing sortable tables in a web application can be achieved using a combination of HTML and JavaScript. Below is a simple example that demonstrates how to make a table sortable.
Basic Sortable Table Example
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Sortable Tables Example</title>
<style>
table {
width: 50%;
border-collapse: collapse;
}
th {
cursor: pointer;
background-color: # f2f2f2;
}
th, td {
padding: 12px;
border: 1px solid # ddd;
text-align: left;
}
</style>
</head>
<body>
<h2>Sortable Table</h2>
<table id="myTable">
<thead>
<tr>
<th onclick="sortTable(0)">Name</th>
<th onclick="sortTable(1)">Category</th>
<th onclick="sortTable(2)">Price</th>
</tr>
</thead>
<tbody>
<tr><td>Apple</td><td>Fruit</td><td>2.50</td></tr>
<tr><td>Banana</td><td>Fruit</td><td>1.25</td></tr>
<tr><td>Carrot</td><td>Vegetable</td><td>0.75</td></tr>
<tr><td>Peach</td><td>Fruit</td><td>3.00</td></tr>
<tr><td>Broccoli</td><td>Vegetable</td><td>1.50</td></tr>
</tbody>
</table>
<script>
function sortTable(columnIndex) {
var table = document.getElementById("myTable");
var rows = table.rows;
var switching = true;
var shouldSwitch, i;
var direction = "ascending";
var switchcount = 0;
while (switching) {
switching = false;
var rowsArray = Array.from(rows).slice(1);
for (i = 0; i < rowsArray.length - 1; i++) {
shouldSwitch = false;
var x = rowsArray[i].getElementsByTagName("TD")[columnIndex];
var y = rowsArray[i + 1].getElementsByTagName("TD")[columnIndex];
if (direction == "ascending") {
if (x.innerHTML.toLowerCase() > y.innerHTML.toLowerCase())
{
shouldSwitch = true;
break;
}
} else if (direction == "descending") {
if (x.innerHTML.toLowerCase() < y.innerHTML.toLowerCase())
{
shouldSwitch = true;
break;
}
}
}
if (shouldSwitch) {
rowsArray[i].parentNode.insertBefore(rowsArray[i + 1], rowsArray[i]);
switching = true;
switchcount++;
} else {
if (switchcount == 0 && direction == "ascending") {
direction = "descending";
switching = true;
}
}
}
}
</script>
</body>
</html>
Explanation:
HTML Structure: The table has column headers (th) that are clickable to trigger sorting. The onclick attribute is used to call the sortTable() function.
JavaScript Sorting Logic: The sortTable() function takes the index of the column to be sorted and rearranges the rows based on the clicked column. The sorting direction toggles between ascending and descending after each click.
This example demonstrates how to implement a basic sortable table with JavaScript and HTML. You can extend this logic to support more complex features, such as sorting numerical values, dates, or even multi-column sorting.
Challenges of Implementing Sortable Tables
While implementing sortable tables can seem straightforward, there are several challenges you may encounter, especially as the complexity of the data grows.
1. Handling Different Data Types
Sorting strings is relatively simple, but sorting numbers, dates, or currency values requires additional logic. For example, a column displaying prices in different currencies must be normalized before sorting.
2. Performance Issues
For very large datasets, sorting can become slow if not handled efficiently. Techniques such as lazy loading, pagination, or client-side sorting libraries can help alleviate performance issues.
3. Maintaining Data Integrity
When sorting data, it's crucial to ensure that related data remains aligned. For instance, if you're sorting a table of products by price, the product names, categories, and other attributes must stay correctly associated with the new order.
4. Accessibility Concerns
Ensuring that sortable tables are accessible to all users, including those using screen readers or keyboard navigation, is essential. Using ARIA attributes like aria-sort helps communicate sorting information to assistive technologies.
Advanced Testing of Sortable Tables with Playwright
Once you’ve implemented a sortable table, it’s important to test that the sorting functionality works as expected. Traditional code-based tests using tools like Playwright can help verify that the table behaves correctly, but manual testing can become tedious, especially when you need to ensure that data integrity is maintained after sorting.
1. Manual Testing with Playwright
Playwright is a powerful end-to-end testing framework that can be used to write tests for sortable tables. Here’s how you can manually test the sorting of a table:
javascript
const { test, expect } = require('@playwright/test');
test.describe('Table Sorting', () => {
test.beforeEach(async ({ page }) => {
await page.goto('https://example.com/sortable-table');
});
test('should verify table data is sorted ascending by name', async ({ page }) => {
// Click on the column header to sort by name
await page.click('#column-button-name');
// Extract the table data after sorting
const tableData = await page.$$eval('#table-id tbody tr', (rows) => {
return rows.map(row => {
const cells = row.querySelectorAll('td');
return Array.from(cells).map(cell => cell.textContent);
});
});
// Verify that the data is sorted correctly
const sortedData = [...tableData].sort((a, b) =>
a[0].localeCompare(b[0]));
expect(tableData).toEqual(sortedData);
});
});
In this example, we use Playwright to:
Navigate to the webpage.
Click on the column header to trigger sorting.
Extract and verify that the data is sorted correctly.
2. Visual Testing with Applitools Eyes
To avoid the tedious process of manually verifying each cell in the table, you can use visual testing tools like Applitools Eyes. Visual testing compares screenshots of the table before and after sorting, ensuring that the layout and content remain correct.
Here’s how you can integrate Applitools Eyes with Playwright:
javascript
const { Eyes, Target } = require('@applitools/eyes-playwright');
test('Visual Testing for Table Sorting', async ({ page }) => {
const eyes = new Eyes();
await eyes.open(page, 'Table Sorting', 'Test Table Ascending Sort');
// Capture the table before sorting
await eyes.check('Before Sort', Target.window().fully());
// Perform sorting by clicking on the column header
await page.click('#column-button-name');
// Capture the table after sorting
await eyes.check('After Sort', Target.window().fully());
// Close the eyes session
await eyes.close();
});
In this test, Applitools Eyes captures screenshots before and after sorting, and automatically compares the images to detect any discrepancies. This method is ideal for ensuring that sorting does not disrupt the layout or integrity of the table data.
Best Practices for Implementing Sortable Tables
To ensure that your sortable tables are user-friendly and performant, follow these best practices:
1. Optimize for Large Datasets
For large datasets, consider using server-side sorting where only the current page of data is sorted on the client side, while the rest is handled by the server. This approach helps with performance issues.
2. Use Accessible Table Elements
Ensure that your tables are accessible by using appropriate ARIA attributes, such as aria-sort, to indicate the sorting state of each column.
3. Implement Multi-Column Sorting
Allow users to sort by multiple columns (e.g., first by name, then by price). This feature adds flexibility and improves the usability of your tables for advanced users.
4. Provide Visual Feedback
When users sort a table, provide visual feedback (such as a sorting arrow) to indicate the current sort order. This helps users understand how the data is currently organized.
5. Test Regularly
Always test your sortable tables to ensure they are functioning as expected. Utilize both functional testing and visual testing to catch issues with sorting logic, performance, or UI rendering.
Conclusion
Sortable tables are a powerful and flexible way to present dynamic data in web applications. By allowing users to reorganize data with ease, you enhance the functionality and usability of your application. Implementing and testing sortable tables can range from basic HTML and JavaScript to more complex solutions involving frameworks like Playwright and Applitools Eyes for visual testing.
By following best practices and utilizing modern testing tools, you can ensure that your sortable tables work efficiently and deliver a smooth user experience.
Key Takeaways
Sortable tables enhance user experience by allowing dynamic data reordering.
They can be implemented using basic HTML and JavaScript, or through more advanced libraries for handling large datasets.
Manual testing with Playwright can verify sorting functionality but requires more effort for large datasets.
Visual testing with Applitools Eyes simplifies the process by comparing the visual state of the table before and after sorting.
Accessibility, performance optimization, and multi-column sorting are essential considerations when building sortable tables.
Regular testing, including both functional and visual checks, ensures sortable tables work as intended and provide a consistent user experience.
FAQs
1. What is a sortable table?
A sortable table allows users to reorder rows of data based on the column headers, providing flexibility in viewing and analyzing data.
2. How do I make an HTML table sortable?
You can make an HTML table sortable by using JavaScript to capture click events on column headers and then reordering the rows accordingly.
3. Can I use Playwright to test sortable tables?
Yes, Playwright can be used to test the functionality of sortable tables by verifying that the data is sorted correctly after user interaction.
4. What is visual testing?
Visual testing involves comparing screenshots of a webpage before and after a change to detect differences, ensuring that the UI and data remain consistent.
5. How does Applitools Eyes work with Playwright?
Applitools Eyes integrates with Playwright to perform automated visual testing, capturing screenshots and comparing them to identify any issues with the display or data.
6. What are some common challenges in implementing sortable tables?
Challenges include handling different data types (e.g., numbers, dates), ensuring performance with large datasets, and maintaining accessibility.
7. Is it possible to sort by multiple columns in a table?
Yes, many implementations allow multi-column sorting, where users can sort by more than one column to further organize data.
8. How do I optimize sortable tables for large datasets?
For large datasets, consider using server-side sorting, pagination, or client-side libraries optimized for performance to handle the sorting efficiently.