Action Class in Selenium: Guide to Mouse & Keyboard Automation 2025
- Gunashree RS
- 20 hours ago
- 8 min read
Modern web applications have evolved far beyond simple click-and-type interactions. Today's users expect rich, interactive experiences with drag-and-drop functionality, hover effects, context menus, keyboard shortcuts, and complex multi-step interactions. For test automation professionals, this evolution presents both opportunities and challenges—how do you effectively simulate these sophisticated user behaviors in your automated tests?
Enter the Action Class in Selenium, a powerful toolkit that bridges the gap between basic element interactions and real-world user behavior. If you've ever struggled with testing complex UI interactions or found yourself limited by simple click() and sendKeys() methods, the Action Class is your gateway to comprehensive interaction testing.
This comprehensive guide will transform your understanding of user interaction automation, taking you from basic concepts to advanced implementation strategies. Whether you're testing modern single-page applications, complex web interfaces, or traditional websites with rich interactions, mastering the Action Class will elevate your automation capabilities to professional levels.
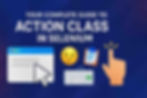
Understanding the Foundation: What is Action Class in Selenium?
The Action Class in Selenium represents a sophisticated approach to user interaction simulation. Unlike basic WebDriver methods that perform simple operations, the Action Class provides a comprehensive framework for executing complex user behaviors that mirror real-world usage patterns.
The Architecture Behind Actions
At its core, the Action Class operates on a builder pattern, allowing you to chain multiple actions together before executing them as a single, coordinated sequence. This approach provides several key advantages:
Precision Control: Execute actions with exact timing and coordination
Complex Sequences: Combine multiple interactions into seamless workflows
Real User Simulation: Replicate authentic user behavior patterns
Cross-Platform Consistency: Maintain behavior across different operating systems and browsers
Basic Action Class Initialization
The foundation of all Action Class operations begins with proper initialization:
import org.openqa.selenium.interactions.Actions;
WebDriver driver = new ChromeDriver();
Actions actions = new Actions(driver);
// Chain actions and execute
actions.moveToElement(element)
.click()
.perform();
This initialization creates an Actions object tied to your WebDriver instance, enabling all subsequent interaction methods.
Comprehensive Mouse Actions: Beyond Basic Clicks
Mouse interactions form the backbone of modern web application testing. The Action Class provides extensive mouse operation capabilities that go far beyond simple clicking.
Essential Mouse Operations
Single Click Actions: While basic click functionality exists in standard WebDriver methods, Action Class clicking provides enhanced control and timing precision.
Double Click Operations: Critical for applications that differentiate between single and double-click behaviors, particularly in data tables, file managers, and complex UI components.
Right-Click (Context Click): Essential for testing context menus, copy-paste operations, and alternative interaction paths that users commonly employ.
Click and Hold: Fundamental for drag operations, selection behaviors, and interactions that require sustained mouse pressure.
Advanced Mouse Movement Techniques
Precision Hover Operations: Move mouse cursor to specific elements with pixel-perfect accuracy, triggering hover states and revealing hidden interface elements.
Offset-Based Movements: Navigate to specific coordinates relative to elements, enabling precise interaction with complex UI components like sliders, charts, and custom controls.
Mouse Action | Use Case | Implementation Complexity |
Basic Click | Button interactions | Low |
Double Click | File/item selection | Medium |
Right Click | Context menus | Medium |
Hover | Dropdown menus | Medium |
Drag and Drop | UI rearrangement | High |
Practical Mouse Action Implementation
public class AdvancedMouseActions {
private Actions actions;
private WebDriver driver;
public void performHoverSequence(WebElement menuItem, WebElement subMenuItem) {
actions.moveToElement(menuItem)
.pause(Duration.ofMillis(500)) // Wait for menu to appear
.moveToElement(subMenuItem)
.click()
.perform();
}
public void performDragAndDrop(WebElement source, WebElement target) {
actions.dragAndDrop(source, target)
.perform();
}
public void performPrecisionClick(WebElement element, int xOffset, int yOffset) {
actions.moveToElement(element, xOffset, yOffset)
.click()
.perform();
}
}
Mastering Keyboard Actions: Advanced Text Input and Shortcuts
Keyboard interactions extend far beyond simple text input. Modern applications rely heavily on keyboard shortcuts, modifier keys, and complex key combinations for power user functionality.
Fundamental Keyboard Operations
Enhanced Send Keys: The Action Class sendKeys() method provides more control than the standard WebElement method, allowing for precise timing and key combination handling.
Key Press and Release: Separate key press and release operations enable complex key combination sequences and sustained key presses.
Modifier Key Handling: Control, Shift, Alt, and Command key operations for shortcuts and complex input scenarios.
Advanced Keyboard Techniques
Multi-Key Combinations: Execute complex shortcuts like Ctrl+Shift+T, Alt+Tab sequences, and application-specific key combinations.
Text Selection Operations: Combine mouse and keyboard actions to select text, copy content, and perform editing operations.
Navigation Key Usage: Arrow keys, Page Up/Down, Home, End, and Tab navigation for comprehensive UI traversal testing.
Keyboard Action Implementation Examples
public class KeyboardActionFramework {
private Actions actions;
public void performSelectAll(WebElement textArea) {
actions.click(textArea)
.keyDown(Keys.CONTROL)
.sendKeys("a")
.keyUp(Keys.CONTROL)
.perform();
}
public void performCopyPaste(WebElement source, WebElement destination) {
// Select all text in source
actions.click(source)
.keyDown(Keys.CONTROL)
.sendKeys("a")
.sendKeys("c")
.keyUp(Keys.CONTROL)
.perform();
// Paste in destination
actions.click(destination)
.keyDown(Keys.CONTROL)
.sendKeys("v")
.keyUp(Keys.CONTROL)
.perform();
}
public void performTabNavigation(int tabCount) {
for (int i = 0; i < tabCount; i++) {
actions.sendKeys(Keys.TAB).perform();
try {
Thread.sleep(100); // Brief pause between tabs
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
}
Complex Interaction Patterns: Combining Actions
Real-world user interactions often involve complex sequences that combine mouse and keyboard actions. The Action Class excels at orchestrating these sophisticated interaction patterns.
Multi-Step Interaction Workflows
File Upload with Drag and Drop: Combine mouse hover, drag operations, and drop actions to simulate modern file upload interfaces.
Form Navigation with Validation: Chain tab navigation, input validation, error handling, and submission processes.
Dynamic Content Interaction: Handle hover-triggered content, delayed loading, and responsive interface elements.
Building Robust Action Sequences
public class ComplexInteractionPatterns {
private Actions actions;
private WebDriverWait wait;
public void performComplexFormFilling(WebElement form) {
// Navigate through form with the tab key, fill fields, and handle validation
actions.click(form)
.sendKeys("John Doe")
.sendKeys(Keys.TAB)
.sendKeys("john.doe@email.com")
.sendKeys(Keys.TAB)
.sendKeys("555-1234")
.sendKeys(Keys.ENTER)
.perform();
// Wait for validation and handle any errors
wait.until(ExpectedConditions.or(
ExpectedConditions.presenceOfElementLocated(By.className("success-message")),
ExpectedConditions.presenceOfElementLocated(By.className("error-message"))
));
}
public void performDragAndDropWithValidation(WebElement source, WebElement target) {
// Get initial states
String sourceText = source.getText();
// Perform drag and drop
actions.dragAndDrop(source, target).perform();
// Validate the operation
wait.until(ExpectedConditions.textToBePresentInElement(target, sourceText));
}
}
Best Practices for Action Class Implementation
Performance Optimization Strategies
Minimize Unnecessary Actions: Avoid redundant movements and clicks that don't add value to your test scenarios.
Use Appropriate Wait Strategies: Combine Action Class operations with explicit waits to handle dynamic content properly.
Chain Actions Efficiently: Group related actions into a single perform() call to reduce execution overhead.
Error Handling and Reliability
Implement Robust Exception Handling: Action Class operations can fail due to timing issues, element state changes, or browser limitations.
Add Verification Steps: Always verify that actions achieved their intended results before proceeding with subsequent operations.
Handle Browser Differences: Different browsers may interpret actions slightly differently, especially regarding timing and coordinate calculations.
Code Organization Best Practices
public class ActionClassBestPractices {
private Actions actions;
private WebDriverWait wait;
public boolean performActionWithVerification(Runnable action, Supplier<Boolean> verification) {
try {
action.run();
return wait.until((driver) -> verification.get());
} catch (Exception e) {
System.out.println("Action failed: " + e.getMessage());
return false;
}
}
public void performRobustHover(WebElement element) {
performActionWithVerification(
() -> actions.moveToElement(element).perform(),
() -> element.getAttribute("class").contains("hover-state")
);
}
}
Advanced Action Class Techniques
Custom Action Builders
Create reusable action components for common interaction patterns:
public class CustomActionBuilder {
private Actions actions;
public CustomActionBuilder hover(WebElement element) {
actions.moveToElement(element);
return this;
}
public CustomActionBuilder pause(int milliseconds) {
actions.pause(Duration.ofMillis(milliseconds));
return this;
}
public CustomActionBuilder clickWithOffset(WebElement element, int x, int y) {
actions.moveToElement(element, x, y).click();
return this;
}
public void execute() {
actions.perform();
}
}
Integration with Page Object Model
public class InteractivePage {
private WebDriver driver;
private Actions actions;
@FindBy(id = "draggable-element")
private WebElement draggableItem;
@FindBy(id = "drop-zone")
private WebElement dropZone;
public InteractivePage(WebDriver driver) {
this.driver = driver;
this.actions = new Actions(driver);
PageFactory.initElements(driver, this);
}
public void performDragAndDrop() {
actions.dragAndDrop(draggableItem, dropZone).perform();
}
public void performHoverAndClick(WebElement hoverElement, WebElement clickElement) {
actions.moveToElement(hoverElement)
.moveToElement(clickElement)
.click()
.perform();
}
}
Frequently Asked Questions
What's the difference between Action Class click() and WebElement click()?
Action Class click() provides more control and can handle complex scenarios like clicking at specific coordinates, while WebElement click() is simpler but more limited. Action Class is better for complex interactions, while WebElement click() is sufficient for basic button clicks.
Can I use Action Class for mobile testing with Appium?
Yes, Action Class works with Appium for mobile testing, but some desktop-specific actions (like right-click) may not be available. Mobile-specific actions like touch, swipe, and pinch are available through Appium's TouchActions class.
How do I handle timing issues with the Action Class?
Use explicit waits before actions, add pause() methods between actions, and implement verification steps after actions. Always wait for elements to be in the expected state before interacting with them.
Why do my drag-and-drop actions sometimes fail?
Drag and drop failures often occur due to timing issues, element not being interactable, or browser-specific behaviors. Try adding pauses, ensuring elements are fully loaded, and using moveToElement() before dragAndDrop().
Can I record and replay Action Class sequences?
While there's no built-in recording feature, you can create custom logging mechanisms to record action sequences and replay them later. Some third-party tools also provide recording capabilities for Selenium actions.
How do I test keyboard shortcuts with Action Class?
Use keyDown() and keyUp() methods for modifier keys, combine them with sendKeys() for letter/number keys, and use Keys enum for special keys like ENTER, TAB, and ESCAPE. Always release modifier keys properly.
What's the best way to debug Action Class failures?
Add logging before each action, take screenshots at key points, use browser developer tools to inspect element states, and implement step-by-step verification. Consider adding explicit waits and element state checks.
Can Action Class handle HTML5 drag and drop?
Yes, but HTML5 drag and drop sometimes requires JavaScript execution for full compatibility. You may need to combine Action Class with JavascriptExecutor for complex HTML5 drag and drop scenarios.
Conclusion
The Action Class in Selenium represents a fundamental shift from basic automation to sophisticated user interaction simulation. By mastering mouse actions, keyboard operations, and complex interaction patterns, you can create test automation that truly reflects real-world user behavior.
The key to success with Action Class lies in understanding that it's not just about executing actions—it's about orchestrating realistic user experiences. The techniques covered in this guide provide the foundation for building robust, maintainable automation frameworks that can handle even the most complex web applications.
Remember that effective Action Class usage requires balancing sophistication with reliability. While the class offers powerful capabilities, the most successful implementations focus on clear, purposeful actions that directly support your testing objectives. By following the best practices and patterns outlined in this guide, you'll be well-equipped to tackle any user interaction challenge in your automation journey.
As web applications continue to evolve toward richer, more interactive experiences, mastering the Action Class becomes increasingly valuable. The investment you make in understanding these concepts will pay dividends in terms of test coverage, reliability, and the ability to catch issues that simpler automation approaches might miss.
Key Takeaways
• Action Class enables sophisticated user interaction simulation beyond basic click and type operations, supporting real-world testing scenarios
• Mouse actions include hover, drag-and-drop, double-click, and right-click operations with precise coordinate control and timing management
• Keyboard actions support complex key combinations, including modifier keys, shortcuts, and navigation sequences for comprehensive input testing
• Builder pattern implementation allows action chaining for creating complex, multi-step interaction sequences that execute as coordinated workflows
• Integration with Page Object Model enhances maintainability by encapsulating complex interactions within reusable page components
• Proper timing and wait strategies are crucial for reliable Action Class implementation, especially with dynamic content and responsive interfaces
• Error handling and verification steps ensure robust automation by confirming actions achieve intended results before proceeding
• Performance optimization through efficient action chaining reduces execution overhead while maintaining comprehensive test coverage
• Cross-browser compatibility requires careful consideration, as different browsers may interpret actions with slight variations in timing and behavior