With the rise of automated testing, developers and testers are constantly seeking tools that simplify and streamline testing processes. While tools like Selenium IDE, Testim, and Katalon have long been used for recording and replaying tests, Google recently entered the field with its Chrome DevTools Recorder. Introduced in November 2021, Chrome DevTools Recorder is a built-in tool that allows users to record, replay, and export tests directly from the browser. This tool provides a convenient, no-frills solution for quickly creating automated tests without needing external applications or switching between tools.
In this article, we’ll explore how the Google Chrome DevTools Recorder works, how to use it for recording tests, and how to export and extend the test scripts. Additionally, we will introduce advanced use cases, including integrating visual testing and exporting tests as Puppeteer Replay scripts, allowing for further customization and flexibility.
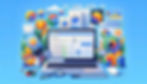
What Is Google Chrome DevTools Recorder?
The Google Chrome DevTools Recorder is a simple yet powerful tool that allows you to record user interactions on a webpage, replay the recorded tests, measure performance, and export the tests for further customization. Embedded directly into Chrome, it saves you the hassle of switching between applications or installing third-party tools.
With DevTools Recorder, you can:
Record user interactions with a webpage, such as clicking buttons, navigating between pages, and more.
Replay the recorded interactions to verify consistency and behavior.
Export the recording as a Puppeteer Replay script or JSON file for further use.
Measure performance by analyzing the time taken for specific steps in the recorded interactions.
The DevTools Recorder is a game-changer for developers and testers alike, offering a built-in, easy-to-use solution for capturing tests.
How to Access Google Chrome DevTools Recorder
Accessing the DevTools Recorder is straightforward. Since it’s built directly into Chrome, you won’t need any additional installations or configurations. Here’s how to get started:
Open Chrome DevTools:
Right-click anywhere on a webpage.
Select "Inspect" from the context menu.
Alternatively, use the shortcut: Ctrl + Shift + I on Windows or Cmd + Option + I on macOS.
Open the Recorder Tool:
In DevTools, click the three dots in the upper-right corner.
Go to More Tools and select Recorder.
You’ll now see the Recorder panel, where you can start recording your interactions.
Recording a Test with Google Chrome DevTools Recorder
Let’s walk through a simple test recording process using the Chrome DevTools Recorder. For this demo, we’ll use a Coffee Cart website where we will record actions such as selecting a product, adding it to the cart, and placing an order.
Steps to Record a Test:
Open the Recorder Tool:
Follow the steps mentioned above to open the Recorder panel.
Start Recording:
Click Start new recording.
Name your recording (e.g., “Order Coffee”).
Click Start recording.
Perform Actions on the Webpage:
Navigate through the webpage and perform actions such as clicking buttons, adding products to the cart, and placing an order. Every action will be recorded in real-time.
Stop Recording:
Once you’ve finished performing the necessary actions, click Stop Recording in the Recorder panel.
Congratulations! You’ve just recorded your first automated test with Chrome DevTools Recorder.
Options After Recording: Replaying, Editing, and Exporting
Once your test is recorded, the Chrome DevTools Recorder provides several useful options:
1. Replay the Test:
You can replay the test directly within the browser to verify that the actions have been correctly captured. This feature is helpful for ensuring that the flow behaves as expected.
How to Replay: Click the Replay button in the Recorder panel. The browser will execute the recorded steps, and you can observe the actions being performed in real time.
2. Measure Performance:
The performance of your test can be analyzed by re-executing the recording and generating a performance report. This helps to identify potential performance bottlenecks, such as slow loading times.
How to Measure Performance: After replaying the test, a Performance panel will open. This panel shows detailed information about the time it took to load resources, rendering times and other performance metrics.
3. Edit and Add Steps:
If you need to modify the recorded test or add additional steps (like waiting for certain elements to load), you can do this directly from the Recorder panel.
How to Edit Steps: Simply click on a recorded step, edit its details, or manually insert new steps. This is especially useful for customizing your test without the need for code.
4. Export the Test:
Once you’re satisfied with the recording, you can export it for use in other testing environments. The Chrome DevTools Recorder supports exporting tests as JSON files or Puppeteer Replay scripts.
How to Export: Click the Export button and choose either:
Export as JSON: A versatile format that can be shared with other tools.
Export as Puppeteer Replay: This generates a script compatible with Puppeteer, which you can extend further.
Exporting your tests as Puppeteer Replay scripts opens up more possibilities for advanced users, allowing them to customize and integrate their tests into larger test suites.
Understanding the Puppeteer Replay Script
When you export a recording as a Puppeteer Replay script, you’ll receive a file containing the recorded test as JavaScript code that can be executed using the Puppeteer framework.
What is Puppeteer?
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium via the DevTools Protocol. Puppeteer can be used for various automated tasks, including generating screenshots, crawling websites, and running automated tests.
Sample Puppeteer Replay Script:
Here’s an example of what a basic Puppeteer Replay script looks like after exporting from DevTools:
javascript
import { createRunner } from '@puppeteer/replay';
export const flow = {
"title": "order-a-coffee",
"steps": [
{
"type": "navigate",
"url": "https://coffeecart.com"
},
{
"type": "click",
"selector": "button.add-to-cart"
},
...
]
};
export async function run() {
const runner = await createRunner(flow);
await runner.run();
}
This script contains the flow object, which represents the sequence of actions recorded in the test. The createRunner function from the Puppeteer Replay library allows you to execute the recorded steps programmatically.
By running this script with Node.js, you can replay the recorded test as often as needed.
Customizing the Puppeteer Replay Script:
The beauty of exporting a Puppeteer Replay script is the ability to customize it further. You can:
Add conditions: Include logic to handle different scenarios or dynamic content.
Integrate with other tools: For example, use Applitools Eyes for visual testing or integrate it into a CI/CD pipeline.
Run headless or headful: Puppeteer runs tests in headless mode by default, but you can turn this off to see the browser in action.
Here’s an example of how you can customize the script to run in headful mode (with the browser visible):
javascript
import puppeteer from 'puppeteer';
import { createRunner } from '@puppeteer/replay';
export async function run() {
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
const runner = await createRunner(flow, { page });
await runner.run();
await browser.close();
}
Adding Visual Testing with Applitools Eyes
Visual testing goes beyond just verifying functionality—it ensures that the user interface appears correctly across different browsers and screen sizes. By integrating Applitools Eyes with your Puppeteer Replay script, you can perform automated visual testing effortlessly.
Steps to Integrate Applitools Eyes:
Install Applitools SDK:
Run the following command to install the Applitools Eyes SDK:
bash
npm install -D @applitools/eyes-puppeteer
Initialize Applitools Eyes:
After launching Puppeteer, initialize
Applitools Eyes:
javascript
import { Eyes, VisualGridRunner } from '@applitools/eyes-puppeteer';
const runner = new VisualGridRunner({ testConcurrency: 5 });
const eyes = new Eyes(runner);
eyes.setApiKey('YOUR_APPLITOOLS_API_KEY');
Add Visual Checkpoints:
In your Puppeteer script, add visual checkpoints to validate the appearance of the page:
javascript
await eyes.open(page, 'Coffee Cart', 'Test Order');
await eyes.check('Coffee Cart Page', Target.window().fully());
Close Applitools Eyes:
Don’t forget to close your Eyes and gather the results:
javascript
await eyes.closeAsync();
Exporting Tests to Cypress from Chrome DevTools Recorder
For teams that prefer using Cypress for end-to-end testing, Chrome DevTools Recorder provides a straightforward method for converting recordings into Cypress tests.
Steps to Export to Cypress:
Install the Cypress Recorder CLI:
bash
npm install -g @cypress/chrome-recorder
Convert the Recording:
bash
npx @cypress/chrome-recorder your-recording-file.json
Run the Cypress Test:
The exported test will be saved in the cypress/integration folder. You can then run it using standard Cypress commands.
Conclusion
The Google Chrome DevTools Recorder simplifies the process of creating, replaying, and exporting tests, making it an essential tool for developers and testers looking for a quick and easy way to automate testing tasks. From basic user interactions to complex visual validations with tools like Applitools, the Recorder provides immense value across the testing lifecycle. Its Puppeteer Replay integration further extends its functionality, offering customization options for more advanced test suites.
Whether you’re a seasoned developer or just getting started with automated testing, the Chrome DevTools Recorder is a powerful addition to your toolset.
Key Takeaways
Chrome DevTools Recorder allows users to record and replay tests directly from the browser.
The tool supports performance measurement, providing insights into load times and resource usage.
Tests can be exported as JSON or Puppeteer Replay scripts for further customization.
Visual testing can be integrated with Applitools Eyes, enabling thorough UI validation.
The tool simplifies test creation without the need for external applications.
Advanced users can customize Puppeteer Replay scripts, turning headless mode on or off and adding complex logic.
The Recorder supports exporting tests to Cypress, making it easy to integrate with different testing frameworks.
Performance analysis capabilities help identify potential performance bottlenecks.
FAQs
1. What is Google Chrome DevTools Recorder?
Chrome DevTools Recorder is a built-in tool in Chrome that allows users to record and replay user interactions on a webpage, as well as measure performance and export tests.
2. Can I export my recordings from Chrome DevTools Recorder?
Yes, you can export recordings as JSON files or Puppeteer Replay scripts for further customization.
3. How do I access Chrome DevTools Recorder?
Open Chrome DevTools, click the three-dot menu in the upper-right corner, go to More Tools, and select Recorder.
4. Can I integrate Applitools Eyes with Chrome DevTools Recorder?
Yes, you can integrate Applitools Eyes with Puppeteer Replay scripts exported from DevTools Recorder to perform automated visual testing.
5. Does Chrome DevTools Recorder support performance testing?
Yes, after recording a test, you can measure its performance and analyze load times, rendering times, and resource usage.
6. Is it possible to export recordings to Cypress?
Yes, recordings can be exported to Cypress using the Cypress Chrome Recorder library.
7. What makes Chrome DevTools Recorder different from other record and playback tools?
Chrome DevTools Recorder is built directly into Chrome, making it highly convenient, and it supports performance testing, Puppeteer Replay, and Cypress integration.
8. Can I edit recorded tests in Chrome DevTools Recorder?
Yes, you can manually edit and add steps to recorded tests directly from the Recorder panel.