Your Ultimate Guide to Understanding Static Meaning in Java
- Gunashree RS
- Jul 20, 2024
- 4 min read
Introduction
In the realm of Java programming, understanding the 'static' keyword is essential for both beginners and experienced developers. The 'static' keyword plays a significant role in defining class-level variables and methods, allowing for memory efficiency and improved performance. This guide will demystify the 'static' keyword, exploring its meaning, usage, and implications in Java programming. Whether you're developing simple applications or complex systems, grasping the concept of 'static' will enhance your coding skills and optimize your software's functionality.
What Does 'Static' Mean in Java?
In Java, the 'static' keyword is used to indicate that a particular member (variable or method) belongs to the class itself rather than to instances of the class. This means that static members are shared among all instances of the class, making them accessible without creating an object of the class.
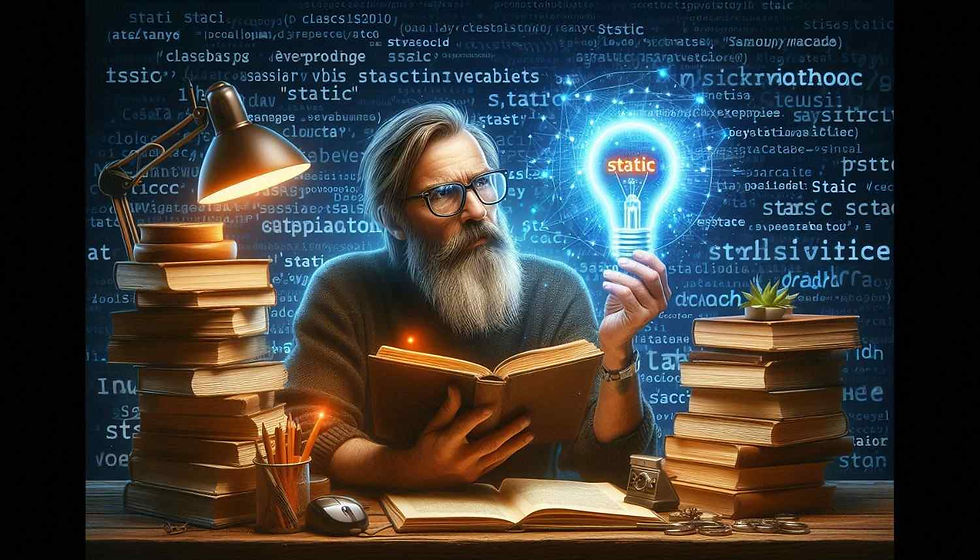
The Role of Static Variables
Static variables are class-level variables that are initialized once when the class is loaded. They retain their value between different instances of the class, providing a way to share data among all objects of the class.
Example:
java
public class MyClass { static int staticVariable = 0; int instanceVariable = 0; public void increment() { staticVariable++; instanceVariable++; } } |
The Role of Static Methods
Static methods, like static variables, belong to the class rather than any particular instance. They can be called without creating an instance of the class. Static methods can only access static variables and other static methods directly.
Example:
java
public class MyClass { static int staticVariable = 0; public static void staticMethod() { System.out.println("Static method called."); System.out.println("Static variable: " + staticVariable); } } |
Benefits of Using Static Members
1. Memory Efficiency:
Static variables are shared among all instances, reducing memory overhead.
2. Utility Methods:
Static methods are ideal for utility or helper methods that do not require instance-specific data.
3. Global Constants:
Static variables can be used to define global constants, ensuring they remain unchanged across instances.
Understanding Static Blocks
Static blocks are used for the static initialization of a class. They are executed when the class is loaded before any static variables or methods are accessed.
Example:
java
public class MyClass { static int staticVariable; static { staticVariable = 10; System.out.println("Static block executed."); } } |
Common Uses of Static in Java
1. Singleton Pattern:
The singleton pattern ensures that a class has only one instance, often implemented using a static variable and a static method.
2. Utility Classes:
Classes like Math in Java use static methods to provide mathematical operations without needing an instance.
3. Main Method:
The main method in Java is static, allowing the Java runtime to invoke it without creating an instance of the class.
Static vs. Instance Members
Static Members:
Belong to the class.
Shared among all instances.
Initialized once when the class is loaded.
Instance Members:
Belong to specific instances.
Each instance has its own copy.
Initialized when the instance is created.
Best Practices for Using Static Members
1. Limit Scope:
Use static members judiciously to avoid potential side effects and maintain encapsulation.
2. Thread Safety:
Ensure thread safety when using static variables in multithreaded environments.
3. Use Final with Static:
Combine static with final for constants to prevent modification and enhance readability.
Common Pitfalls and How to Avoid Them
1. Overuse of Static Variables:
Overusing static variables can lead to tightly coupled code and difficulty in testing. Limit their use to scenarios where a shared state is necessary.
2. Thread Safety Issues:
Static variables can cause concurrency issues in multithreaded applications. Use synchronization techniques to ensure thread safety.
3. Misunderstanding Static Context:
Remember that static methods cannot access instance variables or methods directly. Always pass instance references if needed.
Conclusion
Understanding the 'static' keyword in Java is crucial for efficient memory management and optimizing code performance. By leveraging static variables, methods, and blocks appropriately, developers can create more streamlined and effective applications. Whether defining constants, creating utility methods, or managing shared data, the static keyword offers powerful capabilities that enhance Java programming.
Key Takeaways
The 'static' keyword in Java denotes class-level variables and methods.
Static variables and methods are shared among all instances of the class.
Static blocks are used for class-level initialization.
Best practices for using static members include limiting their scope and ensuring thread safety.
Understanding the differences between static and instance members is essential for effective Java programming.
FAQs
What is a static variable in Java?
A static variable is a class-level variable that is shared among all instances of the class. It is initialized once when the class is loaded and retains its value across instances.
Can static methods access instance variables?
No, static methods cannot directly access instance variables. They can only access static variables and methods.
Why is the main method static in Java?
The main method is static so that it can be invoked by the Java runtime without creating an instance of the class, serving as the entry point for the application.
Can we override static methods in Java?
No, static methods cannot be overridden as they belong to the class, not instances. However, they can be hidden in subclasses.
How do static blocks work in Java?
Static blocks are used for static initialization and are executed when the class is loaded, before any static variables or methods are accessed.
What is the difference between static and final in Java?
Static denotes that a member belongs to the class itself, while final indicates that the member's value cannot be changed once assigned.
Can we have static constructors in Java?
No, Java does not support static constructors. However, static blocks can be used for static initialization.
Are static variables thread-safe in Java?
Static variables are not inherently thread-safe. Synchronization techniques must be used to ensure thread safety in multithreaded environments.
Comentários