Your Guide to Understanding def input in Programming
- Gunashree RS
- Jul 11, 2024
- 5 min read
Updated: Aug 13, 2024
Introduction
In the world of programming, mastering different functions and commands is crucial to becoming an efficient coder. One such command that often comes up in various programming languages is "def input." Understanding how to use this function effectively can significantly streamline your coding process and enhance your problem-solving skills. This article aims to provide an in-depth look at "def input," its applications, and its benefits.
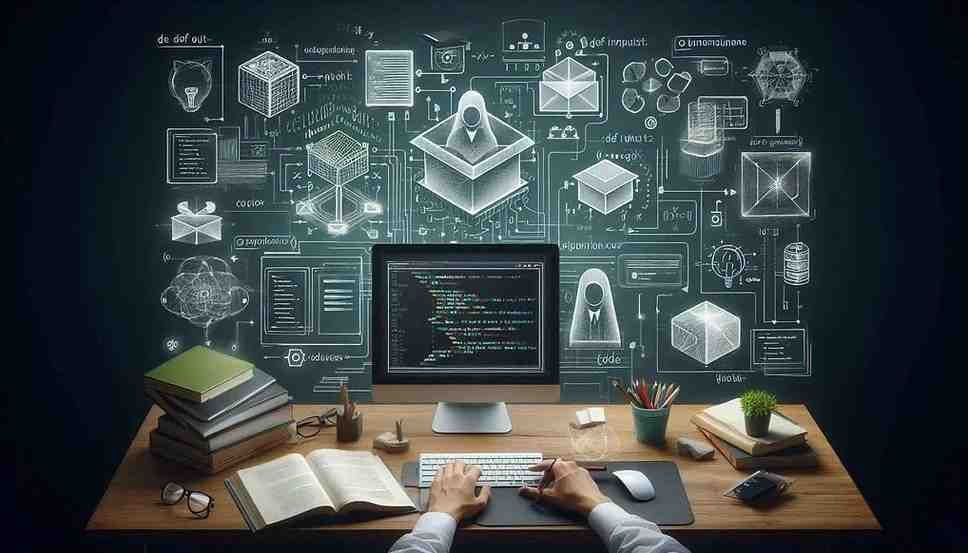
What is "def input"?
The term "def input" typically refers to defining an input function in programming. In languages like Python, "def" is used to define a function, while "input" is a built-in function that allows users to receive input from the console. Combining these two concepts enables the creation of custom input functions that can simplify and organize code.
Defining Functions in Python
In Python, functions are defined using the "def" keyword, followed by the function name and parentheses. Here's a basic example:
python
def my_function(): print("Hello, World!") |
Using the Input Function
The input() function in Python allows you to prompt the user for input. It reads a line from the input, converts it into a string, and returns it. For example:
python
user_input = input("Enter something: ") print("You entered:", user_input) |
Combining "def" and "input"
When you combine "def" and "input," you can create a function that takes user input and processes it in some way. Here's an example:
Python
def get_user_name(): name = input("Enter your name: ") print("Hello,", name) |
Benefits of Using "def input" Functions
Creating custom input functions using "def input" has several advantages:
Reusability: Once defined, you can call the function multiple times without rewriting the code.
Organization: Functions help break down complex code into manageable sections.
Error Handling: Custom input functions can include error checking to ensure valid input.
Practical Applications
Form Validation
In web development, validating user input is essential to ensure data integrity. Custom input functions can be used to validate forms effectively.
python
def get_valid_age(): while True: age = input("Enter your age: ") if age.isdigit() and 0 < int(age) < 120: return int(age) else: print("Please enter a valid age.") |
Data Processing
Custom input functions can streamline data processing tasks. For example, gathering and processing user data in a survey:
python
def collect_survey_data(): responses = [] while True: response = input("Enter your response (type 'done' to finish): ") if response.lower() == 'done': break responses.append(response) return responses |
Best Practices
Meaningful Function Names
Use descriptive names for your functions to make your code more readable. Instead of generic names, choose names that convey the function's purpose.
Docstrings
Include docstrings in your functions to provide a brief explanation of what the function does. This is helpful for anyone reading your code.
python
def get_user_input(): """Prompts the user to enter some input and returns it.""" return input("Enter something: ") |
Error Handling
Incorporate error handling in your custom input functions to manage unexpected input gracefully.
python
def get_positive_number(): while True: try: number = int(input("Enter a positive number: ")) if number > 0: return number except ValueError: print("Invalid input. Please enter a number.") |
Common Mistakes to Avoid
Not Validating Input
Failing to validate user input can lead to unexpected errors or security vulnerabilities. Always check for valid input.
Hardcoding Values
Avoid hardcoding values in your functions. Use variables and parameters to make your functions more flexible and reusable.
Ignoring Edge Cases
Consider edge cases when defining your input functions. For example, what happens if the user enters a very large number or an empty string?
Advanced Techniques
Using Default Parameters
You can provide default values for parameters in your functions. This can make your functions more versatile.
python
def greet_user(name="Guest"): print("Hello,", name) |
Type Annotations
Type annotations can improve code readability and help with debugging by specifying the expected data types of function parameters and return values.
python
def add_numbers(a: int, b: int) -> int: return a + b |
Conclusion
Understanding and effectively using "def input" in programming can significantly enhance your coding skills. By defining custom input functions, you can create more organized, reusable, and error-resistant code. Whether you're validating user input, processing data, or implementing advanced techniques, mastering "def input" functions is a valuable skill for any programmer.
Key Takeaways:
Definition of "def input": In programming, "def input" refers to defining custom input functions, leveraging the "def" keyword to create functions and the "input" function to receive user input.
Creating Functions in Python: Use the "def" keyword to define functions, organizing code into reusable blocks for enhanced efficiency.
Using the Input Function: Incorporate Python's built-in "input()" function to interactively gather user input from the console.
Benefits of Custom Input Functions: Enhance reusability, organization, and error handling in your code by encapsulating input logic into custom functions.
Practical Applications: Apply custom input functions for tasks like form validation and data processing to ensure data integrity and streamline workflows.
Best Practices: Adopt meaningful function names, include docstrings for clarity, and implement robust error handling to improve code quality.
Advanced Techniques: Explore techniques like default parameters and type annotations to further refine the versatility and readability of your functions.
Conclusion: Mastering "def input" functions empowers programmers to write cleaner, more efficient code with improved user interaction and error management.
FAQs
What does "def input" mean in programming?
"Def input" refers to defining an input function in programming, particularly in languages like Python where "def" is used to define a function and "input" is a built-in function for receiving user input.
How do you define a function in Python?
In Python, you define a function using the "def" keyword followed by the function name and parentheses. The function body is indented under the definition.
Why is input validation important?
Input validation ensures that the data entered by users is correct and safe to use, preventing errors and security vulnerabilities in your code.
Can you provide an example of a custom input function?
Sure, here's a simple example:
python
def get_user_name(): name = input("Enter your name: ") print("Hello,", name) |
What are the default parameters in Python functions?
Default parameters allow you to specify default values for function parameters. If the function is called without those parameters, the default values are used.
How can type annotations improve my code?
Type annotations specify the expected data types of function parameters and return values, improving code readability and helping with debugging.
Comments