Your Comprehensive Guide to JavaScript Check if Key Exist
- Gunashree RS
- Jul 10, 2024
- 6 min read
Updated: Aug 13, 2024
Introduction
In JavaScript, ensuring that a key exists in an object is a crucial step in data validation and manipulation. By confirming the existence of a property, you can avoid runtime errors, ensure data integrity, and make your code more robust and maintainable. This comprehensive guide will delve into various methods to check if a key exists in a JavaScript object, providing syntax, examples, and best practices.
Understanding JavaScript Objects
JavaScript objects are collections of key-value pairs. They serve as the foundation for storing structured data in web applications. Each key in an object must be unique, and it can hold any value, including other objects, functions, or primitive data types.
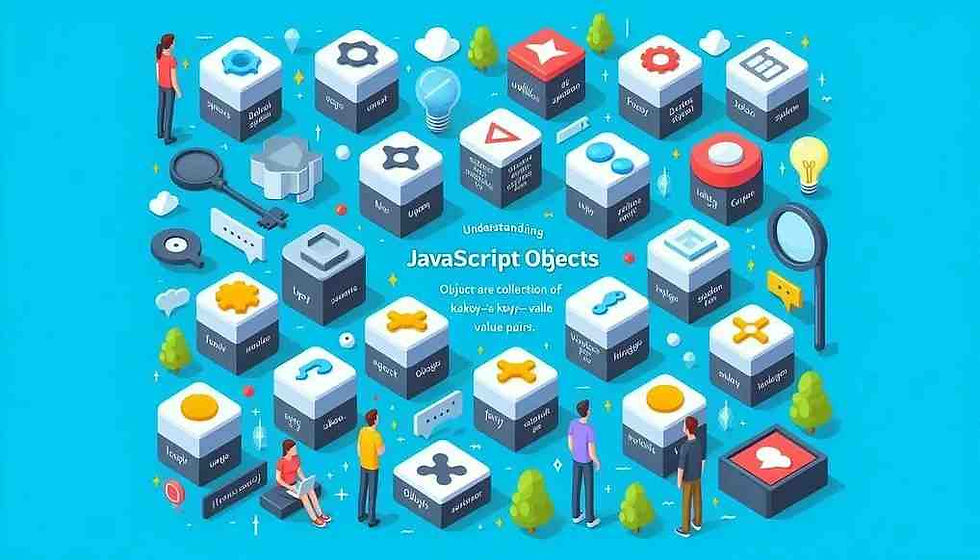
Example of a JavaScript Object
javascript
let exampleObj = { id: 1, remarks: 'Good' }; |
In this guide, we will explore several approaches to check if a key, such as id or remarks, exists in the exampleObj object.
Using the in Operator
The in operator is a simple and effective way to check for the existence of a key in an object. It returns a boolean value indicating whether the specified key is present in the object.
Syntax
javascript
'key' in object |
Example
javascript
let exampleObj = { id: 1, remarks: 'Good' }; // Check for the keys let output1 = 'name' in exampleObj; let output2 = 'remarks' in exampleObj; console.log(output1); // false console.log(output2); // true |
Advantages of Using the in Operator
Simplicity: The in operator is straightforward and easy to use.
Coverage: It checks both own properties and inherited properties.
Using hasOwnProperty() Method
The hasOwnProperty() method is another popular approach for checking the existence of a key. This method is called on the object and checks if it has the specified property as its own (not inherited).
Syntax
javascript
object.hasOwnProperty('key') |
Example
javascript
let exampleObj = { id: 1, remarks: 'Good' }; // Check for the keys let output1 = exampleObj.hasOwnProperty('name'); let output2 = exampleObj.hasOwnProperty('remarks'); console.log(output1); // false console.log(output2); // true |
Advantages of Using hasOwnProperty()
Accuracy: Ensures that the property is an own property, not inherited.
Reliability: Reduces the risk of false positives from inherited properties.
Using Object.keys() Method
The Object.keys() method retrieves an array of an object's own enumerable property names. You can then check if the desired key is included in this array.
Syntax
javascript
Object.keys(object); |
Example
javascript
const obj = { name: 'Sandeep', age: '32' }; if (Object.keys(obj).includes('age')) { console.log('true'); } else { console.log('false'); } |
Advantages of Using Object.keys()
Utility: Useful for getting a list of all keys in an object.
Clarity: Makes it clear what keys exist in the object.
Using typeof Operator
The typeof operator can be used to check if a property is defined. However, it’s not always reliable for checking key existence because it only checks if the value associated with the key is not undefined.
Syntax
javascript
typeof operand // OR typeof (operand) |
Example
javascript
let exampleObj = { id: 1, remarks: 'Good' }; // Check for the keys let output1 = typeof exampleObj['name'] !== 'undefined'; let output2 = typeof exampleObj['remarks'] !== 'undefined'; console.log(output1); // false console.log(output2); // true |
Limitations of typeof Operator
Ambiguity: Cannot distinguish between properties that are explicitly set to undefined and properties that do not exist.
Practical Examples and Use Cases
Let's explore some practical scenarios where checking key existence is essential:
Validating API Responses
When handling API responses, it’s crucial to check for the existence of certain keys to ensure the response contains the expected data.
Example
javascript
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { if ('id' in data && 'remarks' in data) { // Process the data } else { // Handle missing keys } }); |
Form Data Validation
In web forms, validating that the submitted data includes necessary keys helps prevent errors and ensure completeness.
Example
javascript
function validateFormData(formData) { if (formData.hasOwnProperty('name') && formData.hasOwnProperty('email')) { // Process the form data } else { // Handle missing fields } } |
Common Pitfalls and How to Avoid Them
When checking for key existence, be mindful of these common pitfalls:
Confusing Own Properties with Inherited Properties: Use hasOwnProperty() to avoid false positives from inherited properties.
Assuming Non-Existent Keys Are undefined: Use in or Object.keys() to distinguish between undefined values and non-existent keys.
Performance Considerations
The performance of key existence checks can vary based on the method used and the object size. Generally, the in operator and hasOwnProperty() are faster than Object.keys() and typeof.
Benchmarking Example
javascript
console.time('in'); for (let i = 0; i < 1000000; i++) { 'id' in exampleObj; } console.timeEnd('in'); console.time('hasOwnProperty'); for (let i = 0; i < 1000000; i++) { exampleObj.hasOwnProperty('id'); } console.timeEnd('hasOwnProperty'); |
Best Practices for Checking Key Existence
Choose the Right Method: Use the in operator for simplicity, hasOwnProperty() for accuracy, and Object.keys() for utility.
Consider Object Structure: For complex objects, ensure you are checking the correct level of nesting.
Handle Missing Keys Gracefully: Provide fallback values or error handling for missing keys.
Comparative Analysis of Methods
in Operator
Pros: Simple, checks both own and inherited properties.
Cons: Can return true for inherited properties.
hasOwnProperty()
Pros: Accurate, only checks own properties.
Cons: Slightly more verbose.
Object.keys()
Pros: Provides a list of keys, useful for iteration.
Cons: Less efficient for simple existence checks.
typeof
Pros: Can check for undefined values.
Cons: Not reliable for distinguishing between non-existent and undefined keys.
Advanced Techniques
For complex objects with nested keys, you can use a recursive function to check key existence at any level.
Example
javascript
function checkNestedKey(obj, key) { for (let k in obj) { if (k === key) return true; if (typeof obj[k] === 'object' && obj[k] !== null) { if (checkNestedKey(obj[k], key)) return true; } } return false; } |
How to Choose the Right Method
Consider the following criteria when selecting a method:
Complexity of Object: For simple objects, in or hasOwnProperty() is sufficient. For nested objects, consider recursive checks.
Performance Requirements: For performance-critical applications, benchmark the methods to choose the fastest.
Accuracy Needs: Use hasOwnProperty() for the most accurate results.
Case Studies
E-Commerce Application
In an e-commerce application, checking if a product object has keys like price and stock ensures that the necessary information is available for processing orders.
Example
javascript
let product = { name: 'Laptop', price: 1000, stock: 50 }; if ('price' in product && 'stock' in product) { // Proceed with order processing } else { // Handle missing product information } |
Conclusion
Checking if a key exists in a JavaScript object is a fundamental task that ensures data integrity and prevents errors in your code. By understanding and utilizing methods like the in operator, hasOwnProperty(), Object.keys(), and typeof, you can effectively manage and validate your data structures. Choose the method that best fits your needs, considering the complexity of your objects and performance requirements. Implement best practices and handle missing keys gracefully to create robust and maintainable code.
Key Takeaways
Importance of Checking Key Existence:
Ensures data validation and integrity.
Prevents runtime errors.
Enhances code robustness and maintainability.
Understanding JavaScript Objects:
Objects store key-value pairs.
Each key must be unique and can hold various data types.
Methods to Check Key Existence:
in Operator:
Simple and checks both own and inherited properties.
Syntax: 'key' in object
hasOwnProperty() Method:
Checks only own properties.
Syntax: object.hasOwnProperty('key')
Object.keys() Method:
Retrieves an array of own enumerable property names.
Syntax: Object.keys(object)
typeof Operator:
Checks if the value associated with the key is not undefined.
Syntax: typeof operand
Advantages and Limitations:
in Operator:
Pros: Simple, covers inherited properties.
Cons: May return true for inherited properties.
hasOwnProperty():
Pros: Accurate, checks only own properties.
Cons: Slightly more verbose.
Object.keys():
Pros: Useful for getting a list of all keys.
Cons: Less efficient for simple checks.
typeof:
Pros: Can check for undefined values.
Cons: Not reliable for distinguishing between non-existent and undefined keys.
Practical Examples and Use Cases:
Validating API responses.
Form data validation.
Common Pitfalls:
Confusing own properties with inherited properties.
Assuming non-existent keys are undefined.
Performance Considerations:
in Operator and hasOwnProperty() are generally faster than Object.keys() and typeof.
Best Practices:
Choose the right method based on simplicity, accuracy, and utility.
Consider object structure and handle missing keys gracefully.
FAQ
How do you check if a key exists in a JavaScript object?
You can use the in operator, hasOwnProperty() method, Object.keys() method, or typeof operator to check if a key exists in a JavaScript object.
What is the difference between in operator and hasOwnProperty() method?
The in operator checks both own and inherited properties, while hasOwnProperty() only checks own properties.
Is typeof a reliable method for checking key existence?
No, typeof checks if a property is defined and not undefined, but it cannot distinguish between non-existent keys and keys with undefined values.
Which method is the most efficient for checking key existence?
The in operator and hasOwnProperty() method are generally more efficient than Object.keys() for checking key existence.
Can Object.keys() be used to check key existence?
Yes, Object.keys() retrieves an array of keys, and you can use the includes() method to check if a specific key exists.
What are the common pitfalls when checking key existence in JavaScript objects?
Common pitfalls include confusing own properties with inherited properties and assuming non-existent keys are undefined.
Additional Resources
JavaScript: The Good Parts by Douglas Crockford
JavaScript.info: Working with Objects
W3Schools: JavaScript Objects
Comments