Your Comprehensive Guide to Creating Directories in Python
- Gunashree RS
- Jul 8, 2024
- 6 min read
Updated: Aug 13, 2024
Introduction
Creating directories programmatically in Python is essential for tasks ranging from organizing project files to handling large datasets. Python's os module provides robust methods to create directories efficiently and handle potential errors gracefully. This guide aims to equip you with the knowledge to use these methods effectively, ensuring your projects remain organized and maintainable.
Understanding the OS Module
The os module in Python provides a way to interact with the operating system. It includes functions for file and directory management, process management, and more. Here are some key points about the os module:
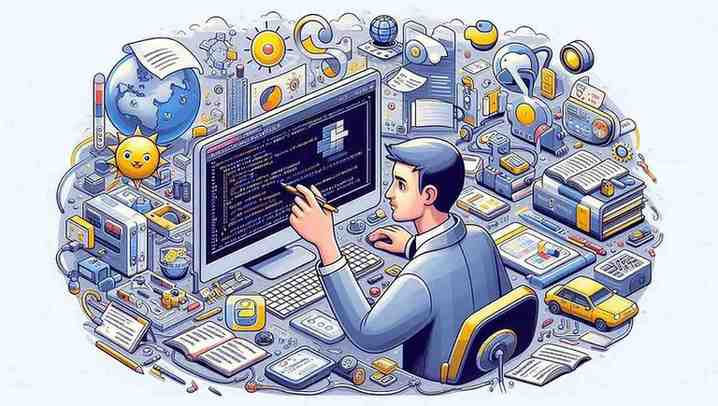
Overview: The os module is part of Python's standard library, making it readily available without the need for additional installations.
Importance: It allows developers to perform operating system-dependent tasks in a portable manner
Common Functions: Functions such as os.mkdir(), os.makedirs(), os.remove(), os.rename(), and os.listdir() are commonly used for file and directory operations.
os.mkdir() Method
The os.mkdir() method creates a single directory at the specified path. Here's a detailed look at its syntax and parameters:
Syntax: os.mkdir(path, mode=0o777, *, dir_fd=None)
Parameters:
path: A path-like object representing the directory path.
mode: (Optional) An integer value representing the directory's mode (permissions). Default is 0o777.
dir_fd: (Optional) A file descriptor referring to a directory. Default is None.
Example 1: Creating a Simple Directory
python
import os
# Directory name directory = "SampleDirectory"
# Parent directory path parent_dir = "C:/Users/YourUsername/Documents"
# Full path path = os.path.join(parent_dir, directory)
# Create the directory os.mkdir(path) print(f"Directory '{directory}' created")
|
Output:
php
Directory 'SampleDirectory' created
|
Example 2: Creating a Directory with Specific Permissions
python
Copy code
import os
# Directory name directory = "TestDirectory"
# Parent directory path parent_dir = "C:/Users/YourUsername/Documents"
# Full path path = os.path.join(parent_dir, directory)
# Directory mode mode = 0o666
# Create the directory with specified mode os.mkdir(path, mode) print(f"Directory '{directory}' created with mode {oct(mode)}")
|
Output:
csharp
Directory 'TestDirectory' created with mode 0o666
|
Handling Errors with os.mkdir()
When using os.mkdir(), several errors can occur, such as trying to create a directory that already exists or specifying an invalid path. Here's how to handle these errors:
Common Errors
FileExistsError: Raised if the directory already exists.
FileNotFoundError: Raised if the specified path is invalid.
Example 3: Handling Errors
Python
import os
# Directory path path = "C:/Users/YourUsername/Documents/ExistingDirectory"
try: os.mkdir(path) except OSError as error: print(f"Error: {error}")
|
Output:
sql
Error: [WinError 183] Cannot create a file when that file already exists: 'C:/Users/YourUsername/Documents/ExistingDirectory'
|
os.makedirs() Method
The os.makedirs() method is used to create directories recursively. This means it can create intermediate-level directories as needed.
Syntax: os.makedirs(path, mode=0o777, exist_ok=False)
Parameters:
path: A path-like object representing the directory path.
mode: (Optional) An integer value representing the directory's mode (permissions). Default is 0o777.
exist_ok: (Optional) If False, an OSError is raised if the directory exists. If True, the error is suppressed.
Example 1: Creating Nested Directories
python
import os
# Directory name directory = "NestedDirectory"
# Parent directories parent_dir = "C:/Users/YourUsername/Documents/Parent/Child"
# Full path path = os.path.join(parent_dir, directory)
# Create the directories os.makedirs(path) print(f"Directory '{directory}' created at path '{path}'")
|
Output:
Lua
Directory 'NestedDirectory' created at path 'C:/Users/YourUsername/Documents/Parent/Child/NestedDirectory' |
Example 2: Creating Directories with Specific Permissions
python
import os
# Directory name directory = "SubDirectory"
# Parent directories parent_dir = "C:/Users/YourUsername/Documents/Main/Branch"
# Full path path = os.path.join(parent_dir, directory)
# Directory mode mode = 0o666
# Create the directories with specified mode os.makedirs(path, mode) print(f"Directory '{directory}' created with mode {oct(mode)}")
|
Output:
csharp
Directory 'SubDirectory' created with mode 0o666
|
Handling Errors with os.makedirs()
Just like os.mkdir(), os.makedirs() can also raise errors. Here's how to handle them:
Example 3: Suppressing Errors
python
import os
# Directory path path = "C:/Users/YourUsername/Documents/ExistingNestedDirectory"
try: os.makedirs(path, exist_ok=True) print(f"Directory '{path}' created successfully") except OSError as error: print(f"Error: {error}")
|
Output:
mathematica
Directory 'C:/Users/YourUsername/Documents/ExistingNestedDirectory' created successfully
|
Comparing os.mkdir() and os.makedirs()
While both methods are used to create directories, they have different use cases:
os.mkdir(): Best for creating a single directory. It raises an error if any part of the path does not exist.
os.makedirs(): Ideal for creating nested directories. It creates intermediate directories as needed.
Best Practices for Directory Management
When creating directories in Python, consider the following best practices:
Naming Conventions: Use meaningful and consistent names for directories.
Error Handling: Always handle potential errors to prevent your program from crashing.
Directory Permissions: Set appropriate permissions to ensure security and accessibility.
Advanced Directory Operations
Beyond simple directory creation, Python allows for advanced operations such as setting directory permissions and creating nested directories.
Directory Permissions
Setting the right permissions is crucial for security and functionality. Use the mode parameter to specify permissions.
Nested Directories
Creating nested directories is straightforward with os.makedirs(), which ensures all necessary intermediate directories are created.
Using Pathlib for Directory Management
The pathlib module offers an object-oriented approach to directory and file management.
Overview
pathlib simplifies path operations and enhances code readability.
Examples
python
from pathlib import Path
# Directory path path = Path("C:/Users/YourUsername/Documents/NewDirectory")
# Create directory path.mkdir(parents=True, exist_ok=True) print(f"Directory '{path}' created")
|
Cross-Platform Compatibility
Ensure your directory creation code works across different operating systems by using absolute paths and handling path separators correctly.
Practical Applications
Directory creation is essential in various scenarios, such as organizing project files, handling large datasets, and managing user uploads in web applications.
Common Mistakes to Avoid
Hardcoding Paths: Use dynamic paths with os.path.join() or pathlib.
Ignoring Errors: Always handle potential errors to ensure robustness.
Inconsistent Naming: Use consistent and meaningful names for directories.
Optimizing Directory Creation
Enhance performance and efficiency by minimizing the number of directory creation operations and using appropriate error handling mechanisms.
Integrating Directory Creation into Larger
Projects
Modularize your directory creation code to make it reusable and maintainable within larger projects.
Modular Code
Organize your directory creation functions into modules for better code management.
Reusability
Design your functions to be reusable across different parts of your project.
Security Considerations
Ensure safe directory creation by setting appropriate permissions and validating paths.
Testing Directory Creation Code
Testing is crucial to ensure your directory creation code works as expected.
Unit Tests
Write unit tests to verify the functionality of your directory creation functions.
Mocking File Systems
Use libraries like pyfakefs to mock file systems and test directory creation in isolation.
Conclusion
Creating directories in Python is a fundamental task that can significantly improve your workflow and project organization. By understanding and utilizing the os module, particularly the os.mkdir() and os.makedirs() methods, you can efficiently manage directories in your applications. Remember to follow best practices, handle errors gracefully, and consider cross-platform compatibility to ensure your code is robust and maintainable.
Key Takeaways:
1. Fundamental Skill: Creating directories in Python is essential for organizing data and managing file systems in projects of any scale.
2. Methods in os Module: Python's os module provides os.mkdir() for single directory creation and os.makedirs() for creating nested directories.
3. Error Handling: Handle common errors like FileExistsError and FileNotFoundError when creating directories using appropriate try-except blocks.
4. Permissions: Use the mode parameter in os.mkdir() and os.makedirs() to set specific permissions for directories.
5. Best Practices: Follow naming conventions, handle errors robustly, and set permissions correctly to ensure secure and efficient directory management.
6. Cross-Platform Compatibility: Ensure directory creation code works across different operating systems by using absolute paths and handling path separators.
7. Advanced Operations: Beyond basic directory creation, explore setting directory permissions, creating nested directories, and using the pathlib module.
8. Integration in Projects: Modularize directory creation code for reusability and maintainability within larger Python projects.
Frequently Asked Questions (FAQs)
What is the difference between os.mkdir() and os.makedirs()?
os.mkdir() creates a single directory, while os.makedirs() can create nested directories, including intermediate ones.
How do I handle errors when creating directories in Python?
Use try-except blocks to catch and handle OSError and its subclasses like FileExistsError and FileNotFoundError.
Can I create directories with specific permissions?
Yes, you can use the mode parameter in both os.mkdir() and os.makedirs() to set specific permissions.
Is it possible to create directories recursively?
Yes, use os.makedirs() to create directories recursively, including any missing intermediate directories.
What are some best practices for directory creation?
Use consistent naming conventions, handle errors gracefully, and set appropriate directory permissions.
How can I ensure my directory creation code is cross-platform compatible?
Use absolute paths, handle different path separators, and test your code on multiple operating systems.
Comments