What is Jenkins Continuous Integration? Your Ultimate Guide
- Gunashree RS
- Aug 6, 2024
- 6 min read
Introduction
In today's fast-paced software development environment, maintaining high code quality while meeting tight deadlines is a challenging task. This is where Continuous Integration (CI) comes in, and Jenkins is one of the most popular tools to implement it. But what exactly is Jenkins Continuous Integration, and how can it benefit your development process? This comprehensive guide will walk you through everything you need to know about Jenkins CI, from its basics to advanced concepts.
What is Jenkins Continuous Integration?
Continuous Integration (CI) is a software development practice where developers frequently merge their code changes into a central repository, followed by automated builds and tests. The primary goal is to identify and address integration issues early in the development cycle. Jenkins, an open-source automation server written in Java, is a widely used tool for implementing CI. It supports building, deploying, and automating software projects.
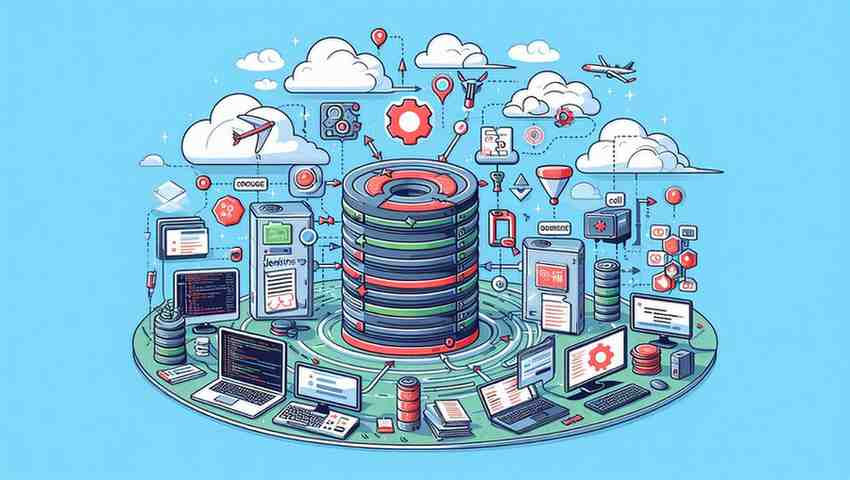
Jenkins can handle various tasks such as:
Building Projects: Compiling source code and creating build artifacts.
Running Tests: Executing automated tests to ensure code quality.
Deploying Applications: Moving the build to different environments.
Integrating with Other Tools: Connecting with version control systems, build tools, and other third-party applications.
How to Create a CI Pipeline in Jenkins
Creating a CI pipeline in Jenkins involves defining a series of automated steps to build, test, and deploy your application. Jenkins offers two primary methods for defining pipelines: Declarative Pipeline and Jenkins Job Builder.
Declarative Pipeline
The Declarative Pipeline syntax simplifies the process of creating a CI pipeline by providing a predefined structure. It is written using Groovy DSL (Domain Specific Language) and allows for easy readability and maintenance.
Sample Declarative Pipeline Script:
groovy
pipeline {
agent any
stages {
stage('Git Checkout') {
steps {
echo 'Cloning repository'
sh 'git clone https://github.com/sample.git'
}
}
stage('Build') {
steps {
echo 'Building project'
sh 'gradle clean build'
}
}
stage('Test') {
steps {
echo 'Running tests'
sh 'gradle test'
}
}
stage('Deploy') {
steps {
echo 'Deploying application'
sh 'gradle deploy'
}
}
}
}
Jenkins Job Builder
Jenkins Job Builder (JJB) uses YAML files to define jobs and pipelines. It is particularly useful for managing a large number of Jenkins jobs through code.
Sample JJB Configuration:
yaml
- project:
name: example-project
jobs:
- '{name}-build'
- '{name}-test'
- job-template:
name: '{name}-build'
builders:
- shell: |
echo 'Building project'
gradle clean build
- job-template:
name: '{name}-test'
builders:
- shell: |
echo 'Running tests'
gradle test
Benefits of Using Jenkins CI
Jenkins CI offers numerous advantages that can significantly enhance your development workflow:
Reduced Development Cycle
Automating the build and test process with Jenkins CI ensures that new features and bug fixes are delivered faster. Developers receive immediate feedback on their code changes, allowing them to make quick adjustments.
Shorter Time to Integrate Code
Manual code integration is time-consuming and error-prone. Jenkins CI automates this process, making it easier to identify and fix integration issues early.
Faster Feedback Loops
Automated testing with Jenkins CI provides instant feedback to developers. If a test fails, they can quickly identify the problematic code and fix it, reducing the time spent on debugging.
Automated Workflow
Jenkins CI automates repetitive tasks, freeing up developers to focus on more critical aspects of the project. It handles the entire build process, from code compilation to deployment.
Concerns While Using Jenkins CI
While Jenkins CI offers many benefits, there are also some concerns to be aware of:
Cost
Setting up and maintaining a Jenkins CI server requires infrastructure and resources. Deploying Jenkins CI on the cloud can be expensive, especially for large projects with extensive testing requirements.
Maintenance
Jenkins CI requires regular maintenance, including updates, plugin management, and pipeline adjustments. This can be time-consuming and requires dedicated resources.
Jenkins Continuous Integration Pipeline
Implementing a Jenkins CI pipeline involves several steps, from setting up Jenkins to configuring the pipeline and running tests.
Setting Up Jenkins
Install Jenkins: Download and install Jenkins on your server. It supports multiple platforms, including Windows, Linux, and macOS.
Configure Jenkins: Set up Jenkins by installing necessary plugins and configuring global settings.
Create a New Job: Define a new Jenkins job and configure its settings.
Configuring the Pipeline
Define the Pipeline Script: Write the pipeline script using either Declarative Pipeline or Jenkins Job Builder.
Add Stages: Break down the pipeline into stages, such as build, test, and deploy.
Configure Triggers: Set up triggers to run the pipeline automatically on code changes.
Running Tests
Unit Tests: Run unit tests to verify the functionality of individual components.
Integration Tests: Execute integration tests to ensure different parts of the application work together.
Acceptance Tests: Perform acceptance tests to validate the application's behavior against user requirements.
Automate Your Jenkins Pipeline
Automating your Jenkins pipeline involves integrating it with other tools and services to streamline the CI process.
Integrating with Version Control Systems
Jenkins can integrate with various version control systems, such as Git, SVN, and Mercurial. This allows Jenkins to automatically trigger builds when code changes are committed.
Using Build Tools
Jenkins supports several build tools, including Maven, Gradle, and Ant. These tools automate the process of compiling and packaging the application.
Integrating with Testing Frameworks
Jenkins can integrate with testing frameworks like JUnit, TestNG, and Selenium. This enables automated testing of the application as part of the CI process.
Using Notification Plugins
Jenkins offers plugins for integrating with communication tools like Slack, email, and Microsoft Teams. These plugins send notifications about build status, test results, and other important events.
Jenkins Continuous Integration Tutorial
This tutorial will guide you through setting up and using Jenkins CI for your project.
Step 1: Installing Jenkins
Download Jenkins from the official website.
Follow the installation instructions for your platform.
Start Jenkins and complete the initial setup.
Step 2: Configuring Jenkins
Install necessary plugins from the Jenkins plugin repository.
Configure global settings, such as system configuration, security settings, and environment variables.
Step 3: Creating a New Job
Go to the Jenkins dashboard and click on "New Item."
Enter a name for your job and select "Pipeline" as the job type.
Configure the job settings, such as source code repository, build triggers, and pipeline script.
Step 4: Writing the Pipeline Script
Define the stages of your pipeline, such as checkout, build, test, and deploy.
Write the pipeline script using either Declarative Pipeline or Jenkins Job Builder.
Save and run the job to start the CI process.
Step 5: Running Tests
Configure the job to run unit, integration, and acceptance tests.
Use testing frameworks like JUnit, TestNG, and Selenium.
Analyze the test results and make necessary code adjustments.
Conclusion
Jenkins Continuous Integration is a powerful tool that can significantly improve the efficiency and quality of your software development process. By automating the build, test, and deployment stages, Jenkins CI helps identify and address issues early, reducing the time and effort required to deliver high-quality software. Whether you're working on a small project or managing a large codebase, Jenkins CI provides the flexibility and scalability you need to streamline your workflow.
Key Takeaways
Continuous Integration ensures code quality by frequently merging and testing code changes.
Jenkins is a popular CI tool that automates the build, test, and deployment process.
Declarative Pipeline and Jenkins Job Builder are two methods for defining Jenkins pipelines.
Jenkins CI offers benefits such as reduced development cycles, faster feedback loops, and automated workflows.
Concerns include the cost and maintenance of Jenkins CI servers.
Automating Jenkins Pipelines involves integrating with version control systems, build tools, and testing frameworks.
Setting Up Jenkins requires installation, configuration, and creating jobs.
Running Tests includes unit, integration, and acceptance tests.
FAQs
How does Jenkins support Continuous Integration?
Jenkins supports Continuous Integration by automating the process of building, testing, and deploying code changes. It integrates with version control systems to trigger builds on code changes and runs automated tests to ensure code quality.
What is the difference between Declarative Pipeline and Jenkins Job Builder?
Declarative Pipeline uses a predefined structure and Groovy DSL to define pipelines, while Jenkins Job Builder uses YAML files for configuration. Declarative Pipeline is easier to read and maintain, while JJB is better for managing large numbers of jobs.
Can Jenkins integrate with other tools and services?
Yes, Jenkins can integrate with various tools and services, including version control systems (e.g., Git, SVN), build tools (e.g., Maven, Gradle), testing frameworks (e.g., JUnit, Selenium), and communication tools (e.g., Slack, email).
How can Jenkins CI improve development workflow?
Jenkins CI improves development workflow by automating repetitive tasks, providing faster feedback on code changes, and ensuring code quality through automated testing. This allows developers to focus on more critical aspects of the project.
What are the costs associated with using Jenkins CI?
The costs associated with using Jenkins CI include infrastructure setup, server maintenance, and potentially cloud deployment fees. These costs can vary depending on the size and complexity of the project.
How does Jenkins handle build and test automation?
Jenkins handles build and test automation by defining pipelines that automate the process of compiling code, running tests, and deploying applications. Pipelines can be configured to trigger automatically on code changes.
Is it necessary to maintain a Jenkins CI server?
Yes, maintaining a Jenkins CI server is necessary to ensure it runs smoothly and can handle new features and updates. Regular maintenance includes updating Jenkins, managing plugins, and adjusting pipelines.
Can Jenkins CI be used for both small and large projects?
Yes, Jenkins CI is scalable and can be used for both small and large projects. It offers flexibility in defining pipelines and integrating with various tools, making it suitable for projects of all sizes.
Comments