Unlocking the Potential of Cores in Java
- Gunashree RS
- Jul 20, 2024
- 4 min read
Updated: Sep 16, 2024
Introduction
Java, one of the most popular programming languages, is known for its versatility and efficiency. With the rise of multi-core processors, understanding how to leverage multiple cores in Java has become crucial for optimizing performance and efficiency. This guide delves into the concept of cores in Java, how to determine the number of cores and best practices for writing multi-threaded Java applications.
Understanding Multi-Core Processors
Multi-core processors consist of multiple processing units (cores) on a single chip, allowing parallel execution of tasks. Utilizing these cores effectively can significantly enhance the performance of Java applications by enabling concurrent processing and reducing execution time.
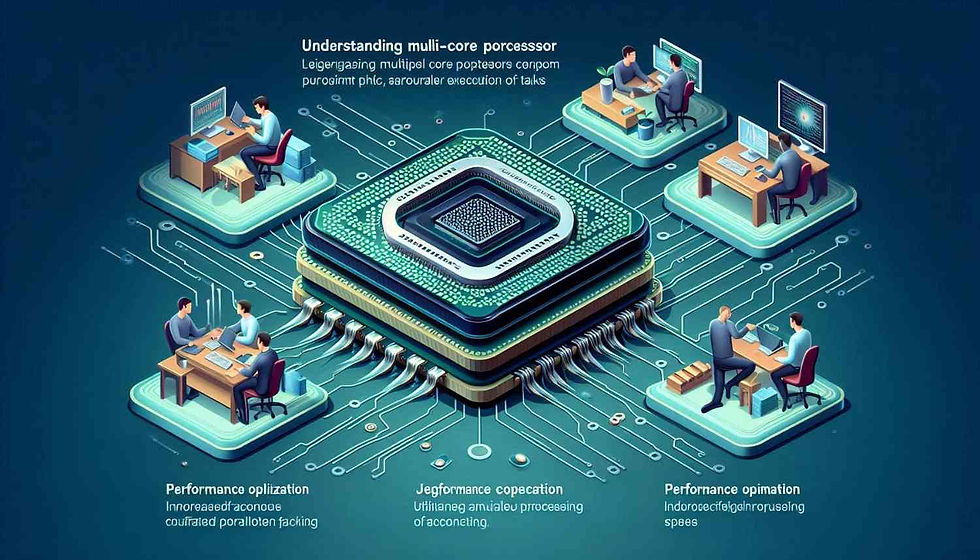
The Importance of Cores in Java
Leveraging multiple cores in Java applications is essential for:
Performance Optimization: Improved processing speed and efficiency.
Scalability: Enhanced ability to handle large-scale applications and high workloads.
Responsiveness: Better user experience through quicker processing of tasks.
Finding the Number of Cores in Java
To utilize multi-core processors effectively, it's important to know how many cores are available. In Java, this can be easily determined using the Runtime class:
java
public class CoreCount { public static void main(String[] args) { int cores = Runtime.getRuntime().availableProcessors(); System.out.println("Number of cores: " + cores); } } |
This simple code snippet will output the number of available cores on the machine running the application.
Multi-Threading in Java
Multi-threading is the concurrent execution of two or more threads to maximize CPU utilization. Java provides robust support for multi-threading through the java.lang.Thread class and the java.util.concurrent package.
Creating Threads in Java
Threads can be created in Java by extending the Thread class or implementing the Runnable interface.
Using the Thread Class:
java
public class MyThread extends Thread { public void run() { System.out.println("Thread is running"); } public static void main(String[] args) { MyThread thread = new MyThread(); thread.start(); } } |
Using the Runnable Interface:
java
public class MyRunnable implements Runnable { public void run() { System.out.println("Runnable is running"); } public static void main(String[] args) { Thread thread = new Thread(new MyRunnable()); thread.start(); } } |
Thread Pools and Executors
To manage multiple threads efficiently, Java provides the Executor framework, which includes thread pools. Thread pools help in reusing threads, reducing the overhead of creating new threads, and managing concurrent tasks effectively.
Creating a Thread Pool:
java
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; public class ThreadPoolExample { public static void main(String[] args) { ExecutorService executor = Executors.newFixedThreadPool(4); for (int i = 0; i < 10; i++) { Runnable worker = new WorkerThread("" + i); executor.execute(worker); } executor.shutdown(); while (!executor.isTerminated()) { } System.out.println("Finished all threads"); } } class WorkerThread implements Runnable { private String command; public WorkerThread(String s) { this.command = s; } @Override public void run() { System.out.println(Thread.currentThread().getName() + " Start. Command = " + command); processCommand(); System.out.println(Thread.currentThread().getName() + " End."); } private void processCommand() { try { Thread.sleep(5000); } catch (InterruptedException e) { e.printStackTrace(); } } } |
Concurrency Utilities in Java
Java provides various concurrency utilities in the java.util.concurrent package to handle synchronization and inter-thread communication more effectively.
CountDownLatch
A synchronization aid that allows one or more threads to wait until a set of operations being performed in other threads completes.
java
import java.util.concurrent.CountDownLatch; public class CountDownLatchExample { public static void main(String[] args) { final int COUNT = 3; CountDownLatch latch = new CountDownLatch(COUNT); for (int i = 0; i < COUNT; i++) { new Thread(new Worker(latch)).start(); } try { latch.await(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("All threads have finished"); } } class Worker implements Runnable { private CountDownLatch latch; public Worker(CountDownLatch latch) { this.latch = latch; } @Override public void run() { System.out.println(Thread.currentThread().getName() + " is working"); try { Thread.sleep(2000); } catch (InterruptedException e) { e.printStackTrace(); } latch.countDown(); } } |
Best Practices for Multi-Threading in Java
Minimize Synchronization: Use synchronization sparingly to avoid contention and deadlocks.
Thread Safety: Ensure that shared resources are thread-safe.
Use Thread Pools: Manage threads efficiently with thread pools instead of creating new threads manually.
Avoid Blocking Operations: Prefer non-blocking algorithms and data structures.
Monitor and Tune Performance: Regularly monitor the performance and tune your application as needed.
Conclusion
Understanding and utilizing cores in Java is essential for optimizing application performance in the modern multi-core processor environment. By leveraging multi-threading, thread pools, and concurrency utilities, you can enhance the scalability, efficiency, and responsiveness of your Java applications. Adopting best practices in multi-threading ensures that your applications remain robust and performant under varying loads.
Key Takeaways
Multi-core processors enhance Java application performance through parallel execution.
Use Runtime.getRuntime().availableProcessors() to find the number of cores.
Create threads by extending Thread or implementing Runnable.
Manage threads efficiently with thread pools from the Executor framework.
Utilize concurrency utilities like CountDownLatch for synchronization.
Follow best practices to ensure robust and performant multi-threaded applications.
FAQs
What is the primary use of multi-core processors in Java?
Multi-core processors allow Java applications to execute multiple threads concurrently, enhancing performance and efficiency.
How can I find the number of cores available in my system using Java?
You can find the number of cores using Runtime.getRuntime().availableProcessors().
What are the methods to create threads in Java?
Threads can be created by extending the Thread class or implementing the Runnable interface.
Why should I use thread pools in Java?
Thread pools manage multiple threads efficiently, reducing the overhead of thread creation and improving performance.
What is CountDownLatch in Java?
CountDownLatch is a synchronization aid that allows threads to wait until a set of operations completes in other threads.
How can I ensure thread safety in my Java application?
Ensure thread safety by synchronizing shared resources, using concurrent data structures, and following best practices for multithreading.
What are the benefits of multi-threading in Java?
Multi-threading improves performance, scalability, and responsiveness by enabling concurrent execution of tasks.
What are some best practices for multi-threading in Java?
Best practices include minimizing synchronization, using thread pools, ensuring thread safety, avoiding blocking operations, and monitoring performance.
Comments