The Ultimate Guide to Keyword Parameters in Python
- Gunashree RS
- Aug 24, 2024
- 7 min read
Updated: Aug 29, 2024
Python, known for its simplicity and flexibility, has various ways to pass arguments to functions. Among these, keyword parameters stand out for their ability to enhance code readability, maintainability, and functionality. Understanding keyword parameters is essential for writing clean, efficient, and Pythonic code. This guide will delve into what keyword parameters are, their significance, and best practices, with real-world examples to illustrate their use.
Introduction to Keyword Parameters in Python
Python functions can take arguments in multiple ways: positional arguments, keyword arguments, and a combination of both. Keyword parameters, or keyword arguments, allow you to specify which arguments a function should use by name rather than by position. This feature provides greater flexibility and clarity, especially in complex functions with many parameters.
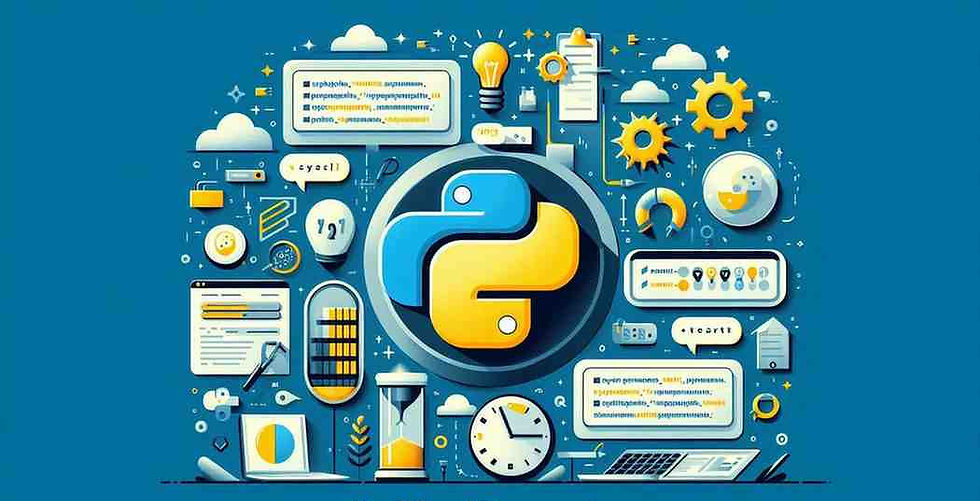
What Are Keyword Parameters?
Keyword parameters are arguments passed to a function by explicitly specifying the parameter name in the function call. This differs from positional arguments, where the order of the arguments matters. By using keyword arguments, you can pass values to specific parameters without worrying about their position in the function signature.
Example:
python
def greet(name, message="Hello"):
return f"{message}, {name}!"
# Using keyword parameters
print(greet(name="Alice", message="Good morning"))
In the above example, the greet function takes two parameters, name and message. By using keyword arguments, we can easily specify the message without worrying about the order of arguments.
Advantages of Keyword Parameters
Keyword parameters offer several benefits that make them a preferred choice in many scenarios:
1. Improved Readability
Keyword parameters make the function call more explicit, making the code easier to understand. When you see a function call with keyword arguments, it's clear what each argument represents, enhancing the code's self-documentation.
2. Flexibility in Argument Order
Unlike positional arguments, the order of keyword arguments doesn't matter. This flexibility allows you to structure function calls in a way that makes sense for your specific use case.
3. Handling Default Values
Keyword parameters work seamlessly with default values, allowing you to override only the necessary parameters without providing values for all arguments.
4. Backward Compatibility
Using keyword arguments can make your code more robust and maintainable. Since the function's signature is less dependent on the argument order, you can modify the function's internals without breaking existing code that uses keyword arguments.
5. Optional Parameters
With keyword parameters, you can create functions that accept optional parameters, which can be skipped during function calls.
Example:
python
def user_profile(username, age=None, location=None):
return f"User: {username}, Age: {age or 'N/A'}, Location: {location or 'N/A'}"
# Call with optional keyword arguments
print(user_profile(username="JohnDoe", location="USA"))
Differences Between Positional and Keyword Parameters
Understanding the distinction between positional and keyword parameters is key to writing effective Python functions.
Positional Parameters
Positional parameters require arguments to be passed in the exact order as defined in the function signature. While simple and effective for functions with a few arguments, positional parameters can become cumbersome in functions with multiple parameters.
Example:
python
def add(x, y):
return x + y
# Positional arguments
print(add(2, 3))
Keyword Parameters
Keyword parameters allow you to specify the values for certain parameters without adhering to the order. This makes the code more readable and reduces the chance of errors.
Example:
python
def add(x, y):
return x + y
# Keyword arguments
print(add(y=3, x=2))
Mixing Positional and Keyword Parameters
Python allows you to use both positional and keyword arguments in the same function call, but there are rules. Positional arguments must be listed before keyword arguments.
Example:
python
def multiply(x, y, z=1):
return x y z
# Mixing positional and keyword arguments
print(multiply(2, 3, z=4))
Attempting to use a keyword argument before a positional one will raise a SyntaxError.
Best Practices for Using Keyword Parameters
To leverage keyword parameters effectively, consider these best practices:
1. Prioritize Readability
Always aim to write clear and readable code. Use keyword arguments to make your function calls self-explanatory. This is especially useful in functions with multiple parameters or where argument names provide significant meaning.
2. Use Default Values Wisely
Assign default values to parameters that are commonly used with the same value. This minimizes the number of arguments passed and simplifies function calls.
Example:
python
def connect(host="localhost", port=5432):
print(f"Connecting to {host} on port {port}")
# Default parameters
connect()
connect(port=3306)
3. Combine with Positional Parameters for Simplicity
For parameters that always need to be provided, use positional arguments. Reserve keyword arguments for optional parameters.
4. Avoid Excessive Use
While keyword parameters are powerful, overusing them can clutter your code. Use them judiciously to maintain a balance between readability and simplicity.
5. Adopt Consistent Naming Conventions
Ensure your keyword parameters have descriptive names that make their purpose clear. Consistency in naming helps maintain readability across the codebase.
Keyword-Only Parameters in Python
Keyword-only parameters are another feature in Python that enforces the use of keyword arguments for certain parameters. Introduced in Python 3, this feature is marked by the * operator in the function definition.
Understanding Keyword-Only Parameters
By placing the * marker before certain parameters in the function definition, you force those parameters to be passed only as keyword arguments. This is useful when you want to ensure that the argument names are explicitly stated, avoiding ambiguity in function calls.
Example:
python
def order(item, quantity, *, delivery=False):
return f"Order: {quantity} x {item}, Delivery: {'Yes' if delivery else 'No'}"
# Keyword-only parameter
print(order("Laptop", 1, delivery=True))
In this example, delivery is a keyword-only parameter. You cannot pass it as a positional argument, making the function call more explicit and preventing potential errors.
Use Cases for Keyword-Only Parameters
Keyword-only parameters are useful in various scenarios:
Clarifying Function Calls: When a function has many optional parameters, keyword-only parameters clarify which arguments are being modified.
Preventing Misuse: By enforcing keyword arguments, you ensure that the function is used correctly, avoiding issues related to positional argument confusion.
Positional-Only Parameters: The Other Side of the Coin
Python 3.8 introduced positional-only parameters, a feature that limits how arguments can be passed to a function. This feature is the counterpart to keyword-only parameters and uses the / symbol in the function signature.
Understanding Positional-Only Parameters
Positional-only parameters are defined before the / symbol in the function definition. These parameters must be passed positionally, and you cannot use their names when calling the function.
Example:
python
def pow(x, y, /, mod=None):
r = x ** y
if the mod is not None:
r %= mod
return r
# Positional-only parameters
print(pow(2, 10))
Here, x and y are positional-only parameters. They must be passed in the correct order without using keywords.
When to Use Positional-Only Parameters
Positional-only parameters are ideal when:
The parameter names are not meaningful to the caller.
You want to enforce a specific calling convention for performance or design reasons.
Avoiding API breakage by preventing the use of parameter names that might change in the future.
Practical Examples of Keyword Parameters in Python
Let’s explore some real-world scenarios where keyword parameters shine.
Configuring a Database Connection
python
def connect_db(host="localhost", port=5432, user="admin", password="admin"):
print(f"Connecting to {host}:{port} as {user}")
# Use default parameters for a quick connection
connect_db()
# Override only the necessary parameters
connect_db(host="192.168.1.1", user="root")
Building a URL with Query Parameters
python
def build_url(base, **params):
query = "&".join(f"{k}={v}" for k, v in params.items())
return f"{base}?{query}"
# Dynamic URL construction with keyword arguments
url = build_url("https://api.example.com/data", page=2, limit=50, sort="asc")
print(url)
Formatting a Message with Optional Details
python
def format_message(name, *, age=None, occupation=None):
message = f"Name: {name}"
if age:
message += f", Age: {age}"
if occupation:
message += f", Occupation: {occupation}"
return message
# Customize the output with optional keyword arguments
print(format_message("Alice", age=30, occupation="Engineer"))
When to Prefer Keyword Parameters Over Positional Parameters
While both positional and keyword parameters have their place, keyword parameters are often preferable in these scenarios:
Complex Functions with Many Parameters
When a function has numerous parameters, keyword arguments enhance clarity by making it clear what each argument represents. This avoids the confusion that can arise from having to remember the order of many positional arguments.
APIs and Libraries
In public APIs or libraries, keyword arguments offer flexibility to the user without risking API breakage due to changes in argument order.
Parameters with Default Values
When dealing with optional parameters that have default values, keyword arguments are ideal because they allow you to override only the necessary values.
Conclusion
Keyword parameters in Python are a powerful feature that enhances the readability, maintainability, and flexibility of your code. By understanding when and how to use keyword parameters, you can write more Pythonic functions that are clear and easy to use. Whether you're designing APIs, writing complex functions, or simply trying to make your code more robust, keyword parameters offer the versatility you need.
Key Takeaways
Keyword parameters allow arguments to be passed by name, improving readability and flexibility.
Positional-only parameters enforce argument order, useful for performance and API design.
Keyword-only parameters require explicit argument names, avoiding ambiguity.
Use cases: Keyword parameters are ideal for complex functions, APIs, and functions with optional arguments.
Best practices: Maintain readability, use default values wisely, and avoid excessive use.
Frequently Asked Questions (FAQs)
1. What is the difference between positional and keyword arguments in Python?
Positional arguments must be passed in the order they appear in the function definition, while keyword arguments are passed by specifying the parameter name, allowing for more flexibility.
2. When should I use keyword parameters?
Use keyword parameters when the function has many parameters, when clarity is essential, or when certain parameters are optional and have default values.
3. Can I mix positional and keyword arguments?
Yes, but positional arguments must be listed before any keyword arguments in the function call.
4. What are keyword-only parameters?
Keyword-only parameters are arguments that must be passed using their parameter names, as indicated by the * marker in the function definition.
5. How do positional-only parameters work in Python?
Positional-only parameters, introduced in Python 3.8, are defined using the / marker and must be passed by position, not by name.
6. Are there performance benefits to using positional-only parameters?
Yes, positional-only parameters can lead to performance improvements by simplifying the argument parsing process.
7. Can I use both positional-only and keyword-only parameters in the same function?
Yes, Python allows a combination of positional-only, positional-or-keyword, and keyword-only parameters in the same function.
8. What is the significance of the * and / symbols in function definitions?
The * enforces keyword-only parameters, while the / enforces positional-only parameters.
Comments