Introduction
Node Package Manager (npm) is a critical tool for developers working with JavaScript, offering a robust package management system that simplifies the process of sharing and reusing code. However, as you work on various projects and install numerous packages, your npm cache can grow significantly. This cache, while beneficial for improving performance, can sometimes cause issues that lead to bugs, outdated packages, or even failed installations. The solution? Deleting or clearing your npm cache.
In this comprehensive guide, we will delve into the intricacies of the npm cache, why you might need to delete it, and how to effectively manage it. Whether you're a seasoned developer or just starting, understanding how to manage your npm cache is essential for maintaining a smooth and efficient workflow.
1. Understanding npm Cache
What is npm Cache?
The npm cache is a local repository where npm stores downloaded packages and their metadata. This cache acts as a backup, allowing npm to avoid re-downloading the same packages repeatedly, thus speeding up subsequent installations and reducing bandwidth usage. Every time you install a package, npm checks its cache first to see if it already has a copy, significantly improving the efficiency of package management.
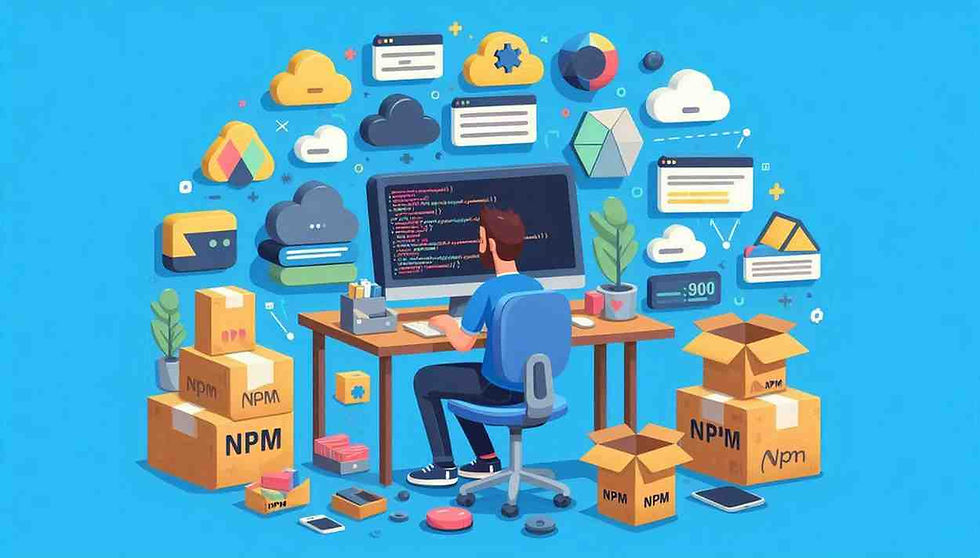
Why Does npm Use Cache?
Caching in npm serves several purposes:
Performance Optimization: By storing packages locally, npm avoids the need to repeatedly fetch the same files from the internet, leading to faster installations.
Offline Installation: If a package is available in the cache, you can install it even without an active internet connection.
Version Management: npm maintains multiple versions of the same package, which helps in maintaining consistency across different projects and versions.
The Benefits and Drawbacks of npm Cache
While npm cache is generally beneficial, it has its pros and cons:
Benefits:
Speed: Faster package installations, especially for large projects.
Reduced Bandwidth Usage: Saves data by reusing cached packages.
Reliability: Provides a fallback if a package is temporarily unavailable online.
Drawbacks:
Disk Space: Over time, the cache can consume significant disk space.
Outdated Packages: If not managed properly, npm might use an outdated package from the cache instead of downloading the latest version.
Cache Corruption: Occasionally, the cache might become corrupted, leading to installation failures and other issues.
2. Why You Might Need to Delete npm Cache
Common Issues Caused by npm Cache
Despite its benefits, the npm cache can sometimes cause problems:
Corrupted Files: Cached files can become corrupted, leading to unexpected errors during package installation.
Outdated Dependencies: If npm uses outdated cached versions of packages, it can cause compatibility issues or fail to incorporate important updates.
Cache Bloat: Over time, the cache can accumulate unnecessary data, consuming disk space and slowing down your system.
When to Consider Deleting npm Cache
You might consider clearing your npm cache under several circumstances:
Installation Failures: If you encounter errors during package installation that are difficult to resolve.
Disk Space Issues: When your system's storage is running low, and you need to free up space.
Outdated Packages: To ensure that npm fetches the latest version of a package rather than relying on an old cached version.
Impact of Cache Deletion on Your Projects
Deleting the npm cache is generally safe and won't break your existing projects. However, it will force npm to re-download all packages the next time you install or update them, which might slightly slow down the process. In some cases, clearing the cache can resolve persistent issues that might otherwise hinder your development workflow.
3. How to Check Your Current npm Cache Status
Inspecting npm Cache Using Commands
Before deleting your npm cache, it's useful to inspect it to understand its current state. npm provides several commands to help you do this:
npm cache verify: This command checks the integrity of your cache and reports any issues it finds. It also removes any corrupted or unnecessary files, ensuring that your cache is in good condition.
npm cache ls: Use this command to list all cached files. It helps you see what is currently stored in your npm cache.
Verifying Cache Integrity
Running npm cache verify is a good first step before deciding to clear your cache. This command checks the validity of the cached files and can resolve minor issues without needing to delete the entire cache. The verification process involves:
Checksum Validation: Ensuring that all cached files match their expected checksums.
Removing Stale Files: Automatically deleting any files that are no longer necessary or are corrupt.
Understanding Cache Contents
The npm cache contains more than just the packages themselves; it also includes metadata, logs, and other auxiliary files. These components work together to make npm installations faster and more reliable. By understanding what's in your cache, you can make informed decisions about what to keep and what to delete.
4. Step-by-Step Guide to Deleting npm Cache
Basic Command: npm cache clean
To delete the npm cache, the basic command you'll use is:
bash
npm cache clean
This command clears out your entire npm cache. However, starting from npm version 5, this command won't work without the --force flag due to safety concerns.
Forceful Cache Clearing with npm cache clean --force
In npm 5 and later, you'll need to add the --force flag to clear the cache:
bash
npm cache clean --force
Using --force ensures that all cache files are deleted, but be cautious. This command is powerful and should be used when you're certain that the cache needs to be cleared. It's especially useful when dealing with stubborn cache issues that don't resolve with other methods.
Deleting Specific Cached Files
If you don't want to delete the entire cache, npm allows you to target specific files or directories:
Deleting a Specific Package Cache: You can remove the cache for a particular package by manually navigating to the cache directory and deleting it.
Using npm Scripts: Customize npm scripts in your package.json to clear specific parts of the cache, making it easier to maintain without wiping everything out.
Clearing Cache for a Specific Project
Sometimes, cache issues might be project-specific. In such cases, it's more efficient to clear the cache for just that project:
Navigate to Project Directory: Go to the root of your project.
Run the Clear Command: Use npm cache clean --force within the project directory to target that specific cache.
5. Advanced npm Cache Management
Automating Cache Deletion
If you frequently encounter cache issues, automating the deletion process can save time. Use task runners like Gulp or Grunt, or configure npm scripts to regularly clean the cache:
json
{
"scripts": {
"clean:cache": "npm cache clean --force"
}
}
Scheduling this script to run periodically can help prevent cache-related problems before they occur.
Managing Cache Across Multiple Projects
For developers working on multiple projects, managing the npm cache across all of them can be challenging. To simplify this:
Global Cache Management: Use the global npm cache for all your projects, ensuring consistency and reducing the risk of conflicts.
Separate Caches for Projects: Alternatively, you can maintain separate caches for each project by configuring .npmrc files with different cache directories.
Using Third-Party Tools for Enhanced Cache Management
There are third-party tools available that offer more control over npm cache management:
npm-cache: A utility that can create, restore, and delete cache archives, making it easier to manage large projects or CI/CD environments.
yarn: Although not an npm tool, Yarn offers robust caching mechanisms and might be worth considering if cache management becomes too cumbersome with npm.
6. Troubleshooting Common npm Cache Issues
Handling Corrupted Cache Files
Corrupted cache files are one of the most common issues developers face. Symptoms include installation errors, missing files, or incorrect versions of packages being installed. Here’s how to deal with it:
Run npm cache verify: This command often fixes minor corruptions automatically.
Manual Deletion: If npm cache verify doesn’t work, you might need to manually locate and delete the corrupted files.
Resolving Cache-Related Installation Failures
If your npm installation is failing, and you suspect the cache is the culprit, follow these steps:
Clear the Cache: Run npm cache clean --force to remove all cached files.
Reinstall Packages: After clearing the cache, try reinstalling your packages. This forces npm to fetch fresh copies, potentially resolving the issue.
Fixing Issues with Outdated Packages
If npm is installing outdated versions of packages, it’s likely due to the cache:
Force Latest Versions: Use npm install package-name@latest to bypass the cache and get the most recent version directly from the npm registry.
Clear Specific Cache Entries: Target the cache of the outdated package and delete it manually, ensuring npm fetches the latest version next time.
7. Best Practices for npm Cache Maintenance
Regular Cache Cleaning: How Often?
While it’s not necessary to clear your npm cache after every install, regular maintenance can prevent issues:
Periodic Cleaning: Consider setting up a schedule to clean the cache every few weeks or months, depending on your development activity.
Project Milestones: Clear the cache after significant project milestones, like major updates or before final deployment.
Strategies for Preventing Cache Issues
Preventing cache issues is better than having to fix them later. Here are some strategies:
Keep npm Updated: Regularly updating npm ensures you have the latest fixes and improvements, including better cache management.
Use CI/CD Pipelines: In automated build environments, configure your CI/CD pipelines to manage caches effectively, reducing the risk of issues during deployment.
Balancing Performance and Stability
Cache management is about finding the right balance between performance and stability:
Performance: Keep the cache intact for faster installations.
Stability: Regularly clean or verify the cache to avoid issues.
A good strategy is to automate cache cleaning but retain essential files that speed up development.
8. npm Cache Configuration Options
Adjusting Cache Settings in npm
npm allows you to configure various cache-related settings to better suit your development environment:
Cache Directory: Use npm config set cache <path> to specify a different cache directory.
Cache Max Age: Control how long npm should keep cached packages using the cache-max setting.
Disable Cache: In rare cases, you might want to disable caching entirely using npm config set cache-min 9999999, but this is generally not recommended.
Using .npmrc for Custom Cache Management
The .npmrc file in your project or user directory can be configured with custom cache settings:
Per-Project Settings: Add project-specific cache settings to .npmrc in your project root.
Global Settings: Adjust global npm cache settings in the user’s .npmrc file.
Optimizing Cache Size and Location
To manage disk space effectively, you might want to:
Limit Cache Size: Use the cache-max and cache-min settings to control how much space npm is allowed to use.
Relocate Cache: Move your npm cache to a drive with more space or faster access speeds for better performance.
9. Understanding npm Cache Behavior in CI/CD Pipelines
The Role of npm Cache in Continuous Integration
In CI/CD pipelines, the npm cache plays a critical role in speeding up builds and deployments:
Cached Dependencies: Reusing cached dependencies can drastically reduce build times.
Consistency: Ensures that builds remain consistent by using the same versions of packages across different environments.
Best Practices for Cache Management in Automated Builds
Managing npm cache effectively in CI/CD pipelines requires careful planning:
Cache Key Management: Use unique cache keys for different builds or branches to avoid conflicts.
Selective Cache Cleaning: Clean the cache only when necessary to preserve build speed while maintaining stability.
Ensuring Cache Consistency Across Environments
To ensure that your builds remain consistent across different environments:
Centralized Cache Management: Use a shared cache location across all build agents.
Automated Cache Validation: Implement scripts that validate the cache before each build to detect issues early.
10. Security Implications of npm Cache
Potential Risks of Cache Mismanagement
If not managed properly, the npm cache can pose security risks:
Stale Packages: Old cached packages might contain vulnerabilities that have since been patched.
Cache Poisoning: Attackers could potentially poison your cache by introducing malicious packages.
Secure Cache Practices for Sensitive Projects
For projects dealing with sensitive data or critical applications:
Regular Cache Audits: Periodically review your cache contents to ensure they’re up to date and secure.
Use npm audit: Run npm audit regularly to detect vulnerabilities in your installed packages.
How to Safeguard Your Cache from Vulnerabilities
To protect your npm cache:
Update Packages: Regularly update your packages to ensure you’re using the latest, most secure versions.
Isolate Caches: Use separate caches for different projects to limit the impact of a potential vulnerability.
11. Clearing npm Cache in Different Environments
Cache Management on Windows
On Windows, clearing the npm cache involves:
Command Prompt or PowerShell: Open a terminal and run npm cache clean --force.
Manual Deletion: Navigate to %AppData%\npm-cache and delete the cache manually if needed.
Deleting Cache on macOS
For macOS users:
Terminal Command: Open Terminal and execute npm cache clean --force.
File Explorer: Navigate to ~/.npm and remove cache files manually.
Linux-Based Cache Clearing
On Linux, you can clear the npm cache by:
Terminal Command: Use npm cache clean --force in your terminal.
Manual Removal: Go to ~/.npm and delete the cache files manually if necessary.
12. The Future of npm Cache
Evolving Cache Management Features
npm continues to evolve, with cache management seeing regular improvements:
Improved Cache Verification: Future versions of npm are likely to include better tools for automatically detecting and fixing cache issues.
Enhanced Performance: Expect more performance enhancements as npm optimizes how it handles and stores cache data.
What npm 7+ and Beyond Bring to the Table
npm 7+ introduced several new features, including:
Workspaces Support: Improved handling of monorepos and workspaces, which can complicate cache management.
Better Dependency Resolution: Enhancements in how npm resolves and caches dependencies, reducing the likelihood of issues.
Community Tools and Innovations
The open-source community often leads the way in developing new tools for npm cache management:
Custom Scripts and Plugins: Developers continue to create custom scripts and plugins that extend npm’s cache management capabilities.
Alternative Package Managers: Tools like Yarn and pnpm offer different approaches to cache management, providing developers with more options.
13. Frequently Asked Questions (FAQs)
How often should I delete my npm cache?
You don’t need to delete your npm cache frequently. Regular checks and periodic cleaning, especially when facing specific issues, should suffice.
Will deleting the cache affect my existing projects?
Deleting the cache won't harm your projects, but it will force npm to re-download all packages, which might slightly slow down the next installation process.
How can I verify if my npm cache is causing issues?
Run npm cache verify to check the integrity of your cache. If this doesn’t resolve your issues, try clearing the cache to see if the problem persists.
Is there a way to recover accidentally deleted cache files?
Once deleted, npm cache files cannot be recovered. However, npm will automatically re-download the necessary files when you next install packages.
What are the risks of not managing the npm cache properly?
Improper cache management can lead to issues like installation failures, outdated packages, and disk space consumption. It can also pose security risks if old, vulnerable packages remain in the cache.
Can I share my npm cache between different machines?
Yes, you can share your npm cache between machines by configuring the cache directory on a shared network drive or using tools designed for cache synchronization.
How does the npm cache differ from other package managers?
npm cache is similar to other package managers in that it stores downloaded packages locally. However, tools like Yarn or pnpm may offer more advanced or different cache management features, such as offline installations and more efficient disk space usage.
Are there any downsides to automating npm cache deletion?
Automating npm cache deletion can lead to slower builds if you clear the cache too frequently. It's important to find a balance that maintains performance while preventing potential issues.
14. Conclusion
Managing your npm cache effectively is crucial for maintaining a smooth development workflow. While the npm cache offers significant performance benefits, it can also cause problems if not managed properly. From understanding what the cache is and why it exists, to learning when and how to delete it, this guide has covered all the essentials you need to know.
By following best practices, leveraging the right tools, and keeping your cache in check, you can avoid many common pitfalls and ensure that your development environment remains stable and efficient. Remember, a well-maintained cache is a key part of a successful development process, enabling you to focus on writing great code without being bogged down by avoidable issues.
15. Key Takeaways
Understand npm Cache: Know what npm cache is and how it benefits your development process.
Regular Maintenance: Periodically clean or verify your cache to prevent issues.
Targeted Deletion: Clear specific cache entries when needed instead of the entire cache.
Use Automation: Automate cache management in CI/CD pipelines for efficiency.
Stay Updated: Keep npm and your packages updated to minimize cache-related problems.
Security First: Regularly audit your cache to ensure it’s free from vulnerabilities.
Custom Configurations: Tailor cache settings in .npmrc to fit your project’s needs.
Comentarios