Mastering Explicit Wait in Selenium: A Comprehensive Tutorial
- 18 hours ago
- 8 min read
Introduction to Explicit Wait in Selenium
When writing automated tests with Selenium WebDriver, one of the most common challenges is synchronizing your test execution with the application's state. Web applications often load elements dynamically, and without proper waiting mechanisms, your tests might fail simply because they're trying to interact with elements that aren't yet ready.
Selenium provides several wait mechanisms to address this challenge, and among them, Explicit Wait stands out as one of the most powerful and flexible options. Unlike Implicit Wait, which applies globally to all elements, Explicit Wait allows you to wait for specific conditions to be met for particular elements, making your tests more precise and efficient.
In this comprehensive guide, we'll dive deep into Explicit Wait in Selenium WebDriver, exploring its syntax, usage patterns, best practices, and real-world examples that will help you create more robust and reliable automated tests.
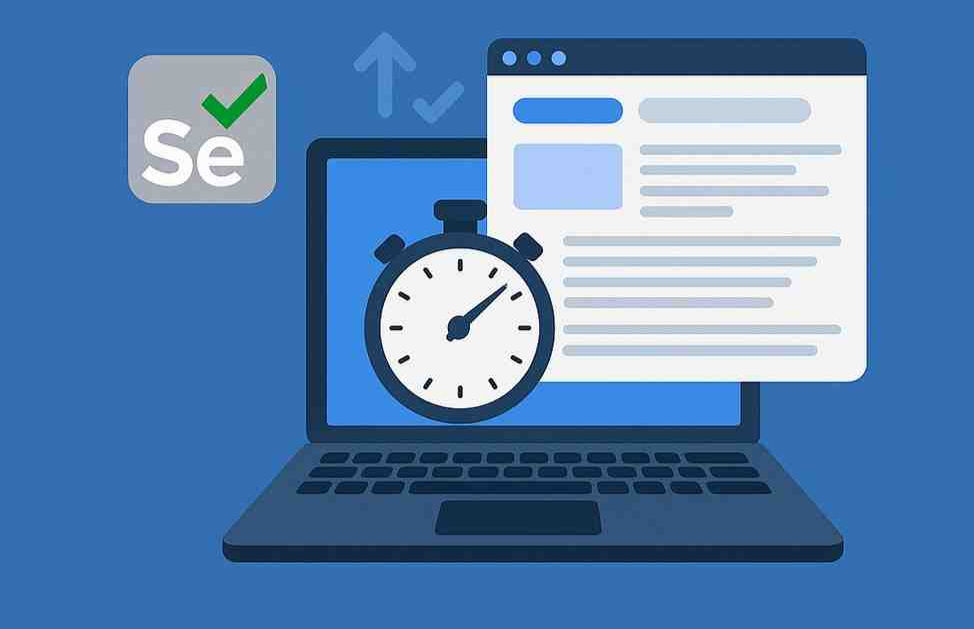
Understanding Selenium Wait Commands
Before we focus specifically on Explicit Wait, let's briefly understand the context of wait commands in Selenium:
Why We Need Wait Commands
Web applications are dynamic, with elements loading at different speeds based on network conditions, server response times, and client-side rendering. Without proper
wait mechanisms, your automated tests will face issues like:
ElementNotVisibleException: When trying to interact with elements that haven't loaded yet
NoSuchElementException: When trying to locate elements that aren't yet in the DOM
Flaky tests: Inconsistent test results due to timing issues
False negatives: Tests failing not because of actual bugs but because of synchronization issues
Selenium provides three main types of wait mechanisms:
Implicit Wait: A global timeout setting for the entire WebDriver session
Explicit Wait: A conditional wait for specific elements or conditions
Fluent Wait: A more configurable version of Explicit Wait with polling frequency control
Let's now focus specifically on Explicit Wait and understand why it's often the preferred choice for test automation professionals.
What is Explicit Wait in Selenium?
Explicit Wait in Selenium is a synchronization mechanism that directs WebDriver to wait for certain conditions to occur before proceeding with executing the next steps in your test script. Unlike Implicit Wait, which applies globally to all elements, Explicit Wait is applied only to specific elements or conditions.
Explicit Wait works by:
Defining a maximum time period to wait
Specifying a condition to wait for
Checking periodically if the condition is met
Proceeding as soon as the condition is met, without waiting for the full timeout period
Throwing an exception if the condition isn't met within the timeout period
This targeted approach makes Explicit Wait more intelligent and efficient, especially when dealing with dynamic web applications where different elements may have different loading times.
When to Use Explicit Wait
Explicit Wait is particularly useful in scenarios such as:
When specific elements take longer to load than others
For elements loaded via AJAX calls
When waiting for animations to complete
When verifying that certain conditions are met before proceeding
In single-page applications, where DOM updates happen without page reloads
When dealing with complex UI interactions that require proper timing
Implementing Explicit Wait in Selenium
To implement Explicit Wait in Selenium WebDriver, you'll need to:
Import the necessary packages
Create a WebDriverWait instance
Define the expected condition
Apply the wait to specific elements or conditions
Required Imports
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
Basic Syntax
// Create a wait with 10 10-second timeout
WebDriverWait wait = new WebDriverWait(driver, 10);
// Wait until the element is visible, then interact with it
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("elementId")));
element.click();
Expected Conditions
Selenium provides a rich set of pre-defined conditions in the ExpectedConditions class. Here are some of the most commonly used ones:
Element visibility and presence:
visibilityOfElementLocated(By locator)
presenceOfElementLocated(By locator)
visibilityOf(WebElement element)
Element state:
elementToBeClickable(By locator)
elementToBeSelected(WebElement element)
elementSelectionStateToBe(WebElement element, boolean selected)
Text-related conditions:
textToBePresentInElement(WebElement element, String text)
textToBePresentInElementLocated(By locator, String text)
textToBePresentInElementValue(By locator, String text)
Frame handling:
frameToBeAvailableAndSwitchToIt(By locator)
Alerts:
alertIsPresent()
Title checks:
titleIs(String title)
titleContains(String title)
Visibility conditions:
invisibilityOfElementLocated(By locator)
invisibilityOfElementWithText(By locator, String text)
This extensive list allows you to wait for virtually any condition that you might need to verify before proceeding with your test execution.
Detailed Example of Explicit Wait in Selenium
Let's look at a complete example that demonstrates how to use Explicit Wait effectively in a real-world scenario:
package waitExample;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.testng.annotations.AfterMethod;
import org.testng.annotations.BeforeMethod;
import org.testng.annotations.Test;
public class ExplicitWaitDemo {
WebDriver driver;
@BeforeMethod
public void setup() {
// Initialize the WebDriver
driver = new FirefoxDriver();
// Navigate to the test application
driver.get("https://the-internet.herokuapp.com/dynamic_loading/1");
// Maximize browser window
driver.manage().window().maximize();
}
@Test
public void testExplicitWait() {
// Click the start button
driver.findElement(By.cssSelector("#start button")).click();
// Initialize WebDriverWait with 30 seconds timeout
WebDriverWait wait = new WebDriverWait(driver, 30);
// Wait until the loading indicator disappears
wait.until(ExpectedConditions.invisibilityOfElementLocated(By.id("loading")));
// Wait until the Hello World text is visible
WebElement helloWorldElement = wait.until(
ExpectedConditions.visibilityOfElementLocated(By.id("finish"))
);
// Verify the text
String actualText = helloWorldElement.getText();
System.out.println("The text is: " + actualText);
// Additional interactions can continue now that the element is confirmed to be visible
}
@AfterMethod
public void tearDown() {
// Close the browser
if (driver != null) {
driver.quit();
}
}
}
In this example:
We navigate to a test page with dynamically loaded content
Click a button that triggers the content loading process
Wait for the loading indicator to disappear
Wait for the target element to become visible
Interact with the element once it's available
This pattern ensures that our test interacts with elements only when they're ready, avoiding flaky tests due to timing issues.
Explicit Wait vs. Implicit Wait in Selenium
Understanding the differences between Explicit Wait and Implicit Wait is crucial for choosing the right waiting strategy for your tests:
Feature | Explicit Wait | Implicit Wait |
Scope | Applied to specific elements | Applied globally to all elements |
Conditions | Can wait for various conditions (visibility, clickability, etc.) | Only waits for the element's presence in the DOM |
Usage | Requires specific code for each wait | Set once and applies throughout the WebDriver instance |
Flexibility | Highly flexible with different conditions | Limited to presence checks only |
Control | Precise control over what to wait for | Global timeout setting only |
Performance | More efficient as it waits only when needed | May slow down tests by always waiting |
Code Complexity | Requires more code | Simpler to implement |
In general, Explicit Wait is recommended for most scenarios because:
It provides more precise control
It can handle complex conditions
It's more efficient as it doesn't add unnecessary waits
It makes tests more readable and maintainable
Best Practices for Using Explicit Wait in Selenium
To get the most out of Explicit Wait in your Selenium tests, follow these best practices:
Choose appropriate timeout values:
Too short: May cause flaky tests.
Too long: May hide performance issues and slow down test execution
Recommended: 10-30 seconds, depending on your application's performance
Use the right ExpectedCondition:
visibilityOfElementLocated instead of presenceOfElementLocated when you need to interact with the element
elementToBeClickable when you need to click on elements
invisibilityOfElementLocated when waiting for elements to disappear
Handle timeout exceptions gracefully:
Implement proper error handling for TimeoutException.
Log meaningful error messages to help with debugging
Avoid mixing Implicit and Explicit Waits:
Using both can lead to unpredictable wait times
Prefer Explicit Wait for most scenarios
Create custom utility methods:
Encapsulate common wait operations in utility methods to improve code reusability.
Consider creating custom ExpectedConditions for application-specific scenarios.
Use Explicit Wait for verifications:
Instead of assertions followed by sleeps, use Explicit Wait to verify conditions.
Common Issues and Troubleshooting
Even with Explicit Wait, you might encounter some challenges. Here are common issues and their solutions:
TimeoutException:
Increase the timeout value.
Verify that the locator is correct.
Check if the condition you're waiting for is realistic
StaleElementReferenceException:
Use fresh element references after page updates
Relocate elements that might have been detached from the DOM
NoSuchElementException despite using waits:
Verify that the element appears in the application
Check if your locator is correct
Consider using presenceOfElementLocated before checking visibility
Slow test execution:
Review your timeout values.
Ensure you're not waiting longer than necessary
Use more specific locators for faster element identification
Conclusion
Explicit Wait is a powerful feature in Selenium WebDriver that helps create more stable and reliable automated tests. By allowing fine-grained control over waiting conditions, it addresses one of the most common challenges in test automation: synchronization.
By mastering Explicit Wait, you can create tests that are not only more reliable but also more efficient and maintainable. The ability to wait for specific conditions makes your tests more robust when dealing with dynamic web applications and varying network conditions.
Remember that effective use of Explicit Wait is about finding the right balance – waiting long enough for elements to be ready, but not so long that your tests become unnecessarily slow. With the knowledge gained from this guide, you should be well-equipped to implement Explicit Wait effectively in your Selenium automation projects.
Key Takeaways
Explicit Wait in Selenium allows you to wait for specific conditions before proceeding with test execution.
Unlike Implicit Wait, Explicit Wait applies only to specific elements, making it more precise and efficient.
WebDriverWait, combined with ExpectedConditions, provides a powerful way to handle dynamic web elements.
Choose the right ExpectedCondition based on what you need to do with the element (visibility, clickability, etc.)
Avoid mixing Implicit and Explicit Waits to prevent unpredictable behavior
Create utility methods for common wait operations to improve code maintainability
Set reasonable timeout values based on your application's performance characteristics
Proper wait strategies are essential for creating reliable, non-flaky automated tests
FAQ Section
What is the difference between Explicit Wait and Fluent Wait in Selenium?
Explicit Wait is a type of wait that pauses the execution until a certain condition is met or the maximum time has elapsed. Fluent Wait is an extension of Explicit Wait that additionally allows you to define the polling frequency (how often Selenium checks for the condition) and which exceptions to ignore during polling. Fluent Wait provides more configuration options for complex scenarios.
How do I handle dynamic elements with Explicit Wait?
For dynamic elements, use appropriate ExpectedConditions like visibilityOfElementLocated or elementToBeClickable with a suitable locator strategy. If elements change their attributes or location, consider using more stable locators like XPath with contains() functions or CSS selectors that target stable attributes.
Can I create custom wait conditions with Explicit Wait?
Yes, you can create custom wait conditions by implementing the ExpectedCondition interface. This is useful for application-specific wait conditions that aren't covered by the built-in ExpectedConditions class.
What is the recommended timeout value for Explicit Wait?
The recommended timeout depends on your application's performance characteristics. Typically, values between 10-30 seconds work well for most web applications. Set it long enough to accommodate reasonable loading times, but not so long that tests take too much time when issues occur.
Can Explicit Wait help with AJAX-based applications?
Absolutely! Explicit Wait is especially useful for AJAX applications where content loads dynamically without full page refreshes. By waiting for specific conditions rather than fixed time periods, your tests can adapt to varying loading times of AJAX components.
Is it possible to use Explicit Wait without ExpectedConditions?
Yes, you can create your own conditions by implementing the ExpectedCondition interface. However, the built-in ExpectedConditions class covers most common scenarios and is recommended for readability and maintainability.
How does Explicit Wait impact test execution time?
Explicit Wait can actually improve test execution time compared to fixed Thread.sleep() calls because it proceeds as soon as the condition is met, without waiting for the full timeout period. This makes tests run faster when the application responds quickly.
Can I use Explicit Wait with any browser supported by Selenium?
Yes, Explicit Wait works with all browsers supported by Selenium WebDriver. The implementation is consistent across different browser drivers, making your wait strategy portable across browsers.
Comments