Guide to Global Variables in Java: Unravel Mysteries
- Gunashree RS
- Jul 26, 2024
- 8 min read
Updated: Sep 21, 2024
Introduction
In the world of Java programming, understanding variables is fundamental to mastering the language. Among the various types of variables, global variables often spark interest and curiosity. This comprehensive guide will delve deep into the concept of global variables in Java, exploring their definition, usage, advantages, disadvantages, and best practices. Whether you are a novice programmer or an experienced developer, this article will provide valuable insights into using global variables effectively and efficiently in your Java projects.
What is a Global Variable in Java?
Definition and Characteristics
A global variable in Java refers to a variable that is accessible from any part of the program. Unlike local variables, which are confined to the scope of a method or block, global variables can be accessed and modified from any method, class, or object within the program. This characteristic makes them particularly useful for sharing data across multiple methods and classes.
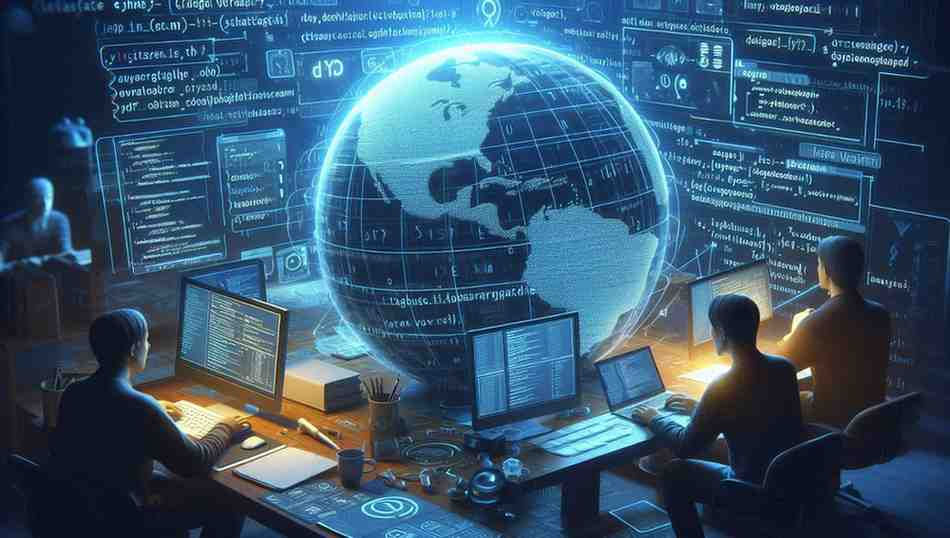
Declaration and Initialization
In Java, global variables are typically declared as static fields within a class. The static keyword indicates that the variable belongs to the class itself, rather than to instances of the class. Here’s an example of how to declare and initialize a global variable in Java:
java
public class GlobalVariablesExample {
public static int globalVar = 10;
}
In this example, globalVar is a global variable that can be accessed from any part of the program using the class name GlobalVariablesExample.globalVar.
Scope and Lifetime of Global Variables
Understanding Scope
The scope of a variable defines the regions of the program where the variable can be accessed. For global variables in Java, the scope extends throughout the entire program. This means that any method or class within the program can access the global variable, provided it has the necessary access permissions.
Lifetime of Global Variables
The lifetime of a global variable is the duration for which the variable exists in memory. Global variables in Java are created when the class is loaded into memory and destroyed when the class is unloaded. This typically means that global variables persist for the entire duration of the program’s execution.
Advantages of Using Global Variables in Java
Easy Data Sharing
One of the primary advantages of global variables is their ability to facilitate easy data sharing across different parts of the program. Since global variables can be accessed from any method or class, they provide a convenient way to share data without the need for complex parameter passing.
Reduced Code Complexity
Global variables can help reduce code complexity by eliminating the need for passing data between methods and classes. This can make the code easier to read and maintain, especially in large and complex programs.
Consistency
Global variables ensure consistency in data access and modification. Since there is only one instance of the global variable, any changes made to the variable are reflected throughout the program, ensuring that all parts of the program have a consistent view of the data.
Disadvantages of Using Global Variables in Java
Increased Risk of Errors
One of the major disadvantages of global variables is the increased risk of errors. Since global variables can be accessed and modified from any part of the program, it becomes challenging to track and control the changes made to the variable. This can lead to unintended side effects and bugs that are difficult to diagnose and fix.
Difficulty in Debugging
Global variables can make debugging more difficult. When a bug arises, it can be challenging to determine which part of the program modified the global variable, especially in large and complex programs. This can significantly increase the time and effort required to debug and fix the issue.
Reduced Modularity
Using global variables reduces the modularity of the code. Since global variables are accessible from any part of the program, they create tight coupling between different parts of the program. This makes it difficult to isolate and test individual components, reducing the overall modularity and maintainability of the code.
Best Practices for Using Global Variables in Java
Minimize Usage
One of the best practices for using global variables is to minimize their usage. Global variables should only be used when absolutely necessary, and alternative approaches such as parameter passing and local variables should be preferred wherever possible.
Encapsulation
Encapsulation is a key principle of object-oriented programming that helps manage the access and modification of global variables. By encapsulating global variables within classes and providing controlled access through getter and setter methods, you can reduce the risk of unintended side effects and improve the maintainability of the code.
Documentation
Proper documentation is essential when using global variables. Clearly document the purpose, usage, and access points of global variables to help other developers understand and maintain the code. This can significantly reduce the risk of errors and improve the overall quality of the code.
Examples of Global Variables in Java
Example 1: Simple Global Variable
Here’s a simple example of a global variable in Java:
java
public class GlobalVariablesExample {
public static int globalVar = 10;
public static void main(String[] args) {
System.out.println("Global Variable: " + globalVar);
modifyGlobalVar();
System.out.println("Global Variable after modification: " + globalVar);
}
public static void modifyGlobalVar() {
globalVar = 20;
}
}
In this example, globalVar is a global variable that is accessed and modified from different methods within the program.
Example 2: Global Variable in a Multi-Class Program
Here’s an example of a global variable in a multi-class program:
java
public class GlobalVariablesExample {
public static int globalVar = 10;
}
class AnotherClass {
public void printGlobalVar() {
System.out.println("Global Variable: " + GlobalVariablesExample.globalVar);
}
public void modifyGlobalVar() {
GlobalVariablesExample.globalVar = 20;
}
}
public class Main {
public static void main(String[] args) {
AnotherClass obj = new AnotherClass();
obj.printGlobalVar();
obj.modifyGlobalVar();
obj.printGlobalVar();
}
}
In this example, globalVar is a global variable that is accessed and modified from different classes within the program.
Common Mistakes to Avoid with Global Variables
Overuse of Global Variables
One of the most common mistakes is the overuse of global variables. While global variables can be convenient, overusing them can lead to an increased risk of errors, reduced modularity, and difficulty in debugging. It is important to use global variables judiciously and only when absolutely necessary.
Lack of Encapsulation
Another common mistake is the lack of encapsulation. Failing to encapsulate global variables within classes and providing uncontrolled access can lead to unintended side effects and bugs. Always encapsulate global variables and provide controlled access through getter and setter methods.
Inadequate Documentation
Inadequate documentation of global variables is a common mistake that can lead to confusion and errors. Always document the purpose, usage, and access points of global variables to help other developers understand and maintain the code.
Global Variables vs. Local Variables
Definition and Scope
Global variables and local variables differ primarily in their scope and accessibility. Global variables are accessible from any part of the program, while local variables are confined to the scope of a method or block. This makes global variables useful for sharing data across different parts of the program, while local variables are more suitable for temporary data storage within a method or block.
Advantages and Disadvantages
Global variables offer the advantage of easy data sharing and reduced code complexity, but they also come with the disadvantages of increased risk of errors, difficulty in debugging, and reduced modularity. On the other hand, local variables offer better modularity and easier debugging, but they require more complex parameter passing for data sharing.
Best Practices
The best practice is to use local variables wherever possible and minimize the use of global variables. When using global variables, always encapsulate them within classes and provide controlled access through getter and setter methods. Proper documentation is also essential to reduce the risk of errors and improve maintainability.
Alternatives to Global Variables in Java
Parameter Passing
One of the most common alternatives to global variables is parameter passing. By passing data as parameters to methods, you can achieve data sharing without the need for global variables. This approach improves modularity and reduces the risk of errors.
Singleton Pattern
The Singleton pattern is another alternative to global variables. By creating a single instance of a class and providing global access to it, you can achieve the benefits of global variables while maintaining better control over data access and modification. Here’s an example of the Singleton pattern in Java:
java
public class Singleton {
private static Singleton instance;
public int value;
private Singleton() {
value = 10;
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
In this example, the Singleton class provides a single instance of the class that can be accessed globally, allowing for controlled data access and modification.
Best Practices for Managing Global Variables
Use Constants
Whenever possible, use constants instead of global variables. Constants are immutable and cannot be modified, reducing the risk of errors and unintended side effects. Here’s an example of using constants in Java:
java
public class Constants {
public static final int MAX_VALUE = 100;
}
In this example, MAX_VALUE is a constant that cannot be modified, ensuring data consistency and reducing the risk of errors.
Thread Safety
When using global variables in a multi-threaded environment, it is essential to ensure thread safety. This can be achieved by using synchronization techniques such as synchronized blocks or methods, volatile keywords, and thread-safe classes from the java.util.concurrent package. Here’s an example of ensuring thread safety in Java:
java
public class GlobalVariablesExample {
private static int globalVar = 10;
public static synchronized void incrementGlobalVar() {
globalVar++;
}
public static synchronized int getGlobalVar() {
return globalVar;
}
}
In this example, the synchronized keyword ensures that access to the global variable is thread-safe.
Conclusion
Global variables in Java offer a convenient way to share data across different parts of the program, but they come with their own set of challenges and risks. By understanding the nuances of global variables, including their advantages, disadvantages, and best practices, you can use them effectively and efficiently in your Java projects. Always strive to minimize the use of global variables, encapsulate them within classes, ensure proper documentation, and follow best practices for managing global variables. By doing so, you can improve the modularity, maintainability, and overall quality of your code.
Key Takeaways
Global variables in Java are accessible from any part of the program, facilitating easy data sharing.
Advantages include reduced code complexity and consistency in data access.
Disadvantages involve increased risk of errors, difficulty in debugging, and reduced modularity.
Best practices include minimizing usage, encapsulation, and proper documentation.
Alternatives like parameter passing and the Singleton pattern can reduce reliance on global variables.
Thread safety is crucial in multi-threaded environments, achievable through synchronization techniques.
FAQs
What is a global variable in Java?
A global variable in Java is a variable that is accessible from any part of the program, typically declared as a static field within a class.
How do you declare a global variable in Java?
Global variables in Java are declared using the static keyword within a class. For example:
java
public class Example {
public static int globalVar = 10;
}
What are the advantages of using global variables?
Global variables facilitate easy data sharing, reduce code complexity, and ensure consistency in data access and modification.
What are the disadvantages of using global variables?
Global variables can increase the risk of errors, make debugging difficult, and reduce the modularity of the code.
How can you minimize the use of global variables?
Minimize the use of global variables by using parameter passing, the Singleton pattern, and local variables wherever possible.
What is the Singleton pattern?
The Singleton pattern is a design pattern that restricts the instantiation of a class to a single instance and provides global access to that instance.
How do you ensure thread safety with global variables?
Ensure thread safety with global variables by using synchronization techniques such as synchronized blocks or methods, volatile keywords, and thread-safe classes from the java.util.concurrent package.
What are some best practices for managing global variables?
Best practices for managing global variables include minimizing their use, encapsulating them within classes, using constants, ensuring thread safety, and proper documentation.
Commentaires