Guide to R Cores: Maximize Performance in R
- Gunashree RS
- Jul 20, 2024
- 5 min read
Updated: Aug 8, 2024
Introduction
R, a powerful programming language for statistical computing and graphics, is widely used for data analysis, machine learning, and scientific research. As datasets grow larger and analyses become more complex, optimizing performance becomes crucial. One effective way to boost performance is by leveraging multiple cores of a CPU. This guide will explore the concept of R cores, how to check CPU usage, and strategies to maximize computational efficiency.
Understanding R Cores
What Are R Cores?
Cores are the individual processing units within a CPU. Modern CPUs often have multiple cores, enabling parallel processing of tasks. In R programming, utilizing multiple cores can significantly speed up data processing and analysis tasks.
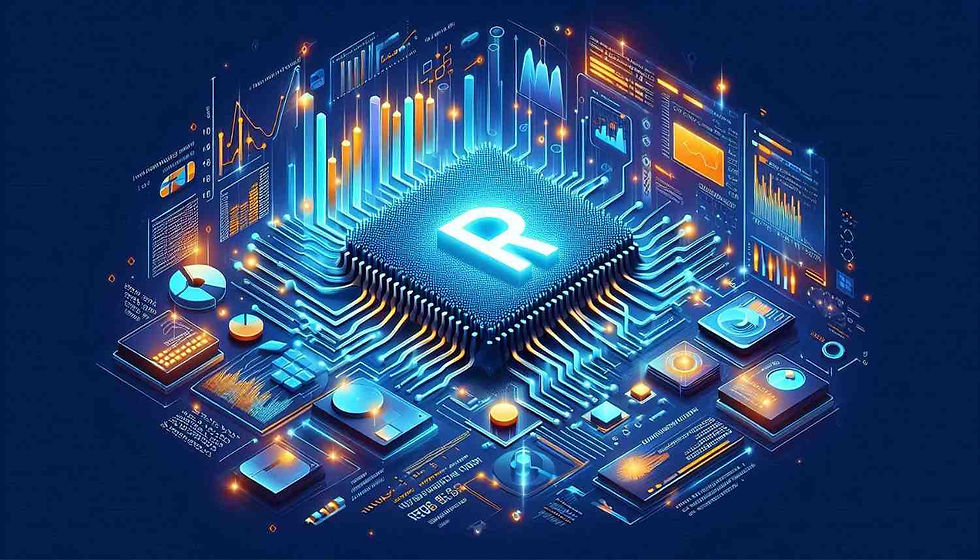
Why Use Multiple Cores in R?
Using multiple cores allows for parallel execution of tasks, reducing computation time. This is especially beneficial for large datasets and computationally intensive operations, such as simulations, bootstrapping, and machine learning algorithms.
The Basics of Parallel Processing
Parallel processing involves dividing a task into smaller sub-tasks that can be executed simultaneously across multiple cores. In R, several packages facilitate parallel processing, enabling efficient use of available CPU resources.
Checking Available Cores and CPU Usage
How to Check Available Cores in R
To determine the number of cores available on your system, you can use the parallel package in R. Here’s a simple way to check:
r
library(parallel) numCores <- detectCores() print(numCores) |
This code snippet loads the parallel package and uses the detectCores function to find the number of available cores.
Monitoring CPU Usage in R
Monitoring CPU usage helps you understand the computational load and optimize performance. The parallel package provides functions to manage and monitor parallel tasks:
r
This example creates a cluster using all available cores, evaluates system information, and then stops the cluster.
Using the future Package for CPU Monitoring
The future package offers a user-friendly approach to parallel processing and CPU usage monitoring:
r
library(future) plan(multiprocess) availableCores() |
This code sets up a multiprocess plan and checks the available cores.
Utilizing R Cores for Parallel Processing
Popular R Packages for Parallel Processing
Several R packages are designed for parallel processing, each with unique features and advantages:
parallel: Part of the base R distribution, offering functions for parallel execution.
foreach: Provides a simple syntax for parallel loops.
future: Simplifies parallel processing with a consistent API.
doParallel: Works with foreach to execute tasks in parallel.
Example: Parallel Processing with foreach
The foreach package is widely used for parallel loops. Here’s an example of using foreach with doParallel:
r
library(foreach) library(doParallel) cl <- makeCluster(detectCores() - 1) registerDoParallel(cl) results <- foreach(i = 1:10) %dopar% { sqrt(i) } stopCluster(cl) print(results) |
This code sets up a cluster, registers it for parallel processing, performs a parallel loop to calculate square roots, and stops the cluster.
Example: Parallel Processing with future
The future package provides an easy-to-use framework for parallel processing:
r
library(future) plan(multiprocess) results <- future_lapply(1:10, sqrt) print(results) |
This code sets up a multiprocess plan and performs a parallel lapply to calculate square roots.
Best Practices for Efficient Parallel Processing
Balancing Load Across Cores
Efficient parallel processing requires balancing the computational load across available cores. Uneven distribution can lead to some cores being underutilized while others are overburdened.
Avoiding Common Pitfalls
Overhead: Parallel processing introduces overhead from task management and data transfer. Ensure the benefits outweigh the overhead.
Memory Usage: Be mindful of memory usage, as parallel tasks can consume significant resources.
Synchronization: Avoid excessive synchronization, which can negate the benefits of parallel processing.
Profiling and Optimization
Profiling your code helps identify bottlenecks and optimize performance. Use R profiling tools such as profvis to analyze and improve your parallel processing code.
Advanced Techniques
Dynamic Cluster Management
Dynamic cluster management involves adjusting the number of cores used based on the computational load. The future package supports dynamic cluster resizing:
r
library(future) plan(cluster, workers = 2:4) |
Nested Parallelism
Nested parallelism allows for parallel execution within parallel tasks. This is useful for complex workflows but requires careful management to avoid excessive overhead.
GPU Acceleration
For tasks requiring significant computational power, consider using GPUs (Graphics Processing Units). The gpuR package in R facilitates GPU-accelerated computations.
Practical Applications of R Cores
Data Analysis
Parallel processing can significantly speed up data analysis tasks such as data cleaning, transformation, and aggregation.
Machine Learning
Machine learning algorithms, particularly those involving large datasets and complex models, benefit greatly from parallel processing.
Simulations and Bootstrapping
Simulations and bootstrapping, which often involve repetitive computations, are ideal candidates for parallel processing.
Conclusion
Leveraging multiple cores in R can dramatically enhance the performance of your data analysis and computational tasks. By understanding how to check available cores, monitor CPU usage, and implement parallel processing with various R packages, you can optimize your workflows and achieve faster results. Whether you're working with large datasets, complex machine learning models, or intensive simulations, efficient use of R cores is a valuable skill for any R programmer.
Key Takeaways
Understand R cores: Learn the basics of cores and their role in parallel processing.
Check available cores: Use the parallel package to determine the number of available cores.
Monitor CPU usage: Employ parallel and future packages for efficient CPU usage monitoring.
Utilize parallel processing: Implement parallel processing with packages like foreach and future.
Balance computational load: Ensure even distribution of tasks across cores for optimal performance.
Profile and optimize: Use profiling tools to identify bottlenecks and optimize your code.
Advanced techniques: Explore dynamic cluster management, nested parallelism, and GPU acceleration.
Practical applications: Apply parallel processing to data analysis, machine learning, and simulations.
FAQs
How can I check the number of available cores in R?
You can use the parallel package and the detectCores function to check the number of available cores.
What are the benefits of using multiple cores in R?
Using multiple cores enables parallel processing, reducing computation time for large datasets and complex tasks.
Which R packages are best for parallel processing?
Popular packages include parallel, foreach, future, and doParallel.
How do I monitor CPU usage in R?
You can monitor CPU usage using the parallel and future packages to manage and evaluate parallel tasks.
What is the foreach package used for?
The foreach package is used for parallel loops, enabling efficient execution of repetitive tasks across multiple cores.
How do I avoid common pitfalls in parallel processing?
Avoid pitfalls by balancing the computational load, managing memory usage, and minimizing synchronization overhead.
Can I use GPUs for parallel processing in R?
Yes, the gpuR package allows for GPU-accelerated computations, providing significant performance improvements for certain tasks.
What are nested parallelism and dynamic cluster management?
Nested parallelism involves parallel execution within parallel tasks, while dynamic cluster management adjusts the number of cores used based on the computational load.
Comments