Introduction
In today's fast-paced software development environment, ensuring the reliability and robustness of JavaScript code is more important than ever. With countless lines of code written daily, maintaining a stable codebase becomes a critical task, and one of the most effective ways to achieve this is through comprehensive testing. Enter Jest, a powerful testing framework that has become a favorite among developers for its simplicity, flexibility, and rich feature set. Paired with npm, the ubiquitous Node Package Manager, Jest offers a seamless testing experience that helps developers maintain code quality with ease.
This guide will take you on a journey to mastering npm Jest, providing detailed insights into its installation, configuration, and advanced features. Whether you're just starting with JavaScript testing or looking to refine your testing strategy, this guide is designed to equip you with the knowledge and tools necessary to elevate your testing game.
What is npm Jest?
Jest is a delightful JavaScript testing framework developed by Facebook, known for its zero-configuration setup, built-in assertions, and rich mocking features. It integrates seamlessly with npm, the package manager for Node.js, which is widely used to install, manage, and run JavaScript dependencies. Together, npm Jest provides a robust solution for testing JavaScript applications, ensuring that your code is bug-free, efficient, and ready for production.
In this guide, we'll dive deep into npm Jest, covering everything from installation to advanced testing techniques, and even troubleshooting common issues. By the end of this guide, you'll be well-versed in using npm Jest to create reliable and maintainable JavaScript applications.
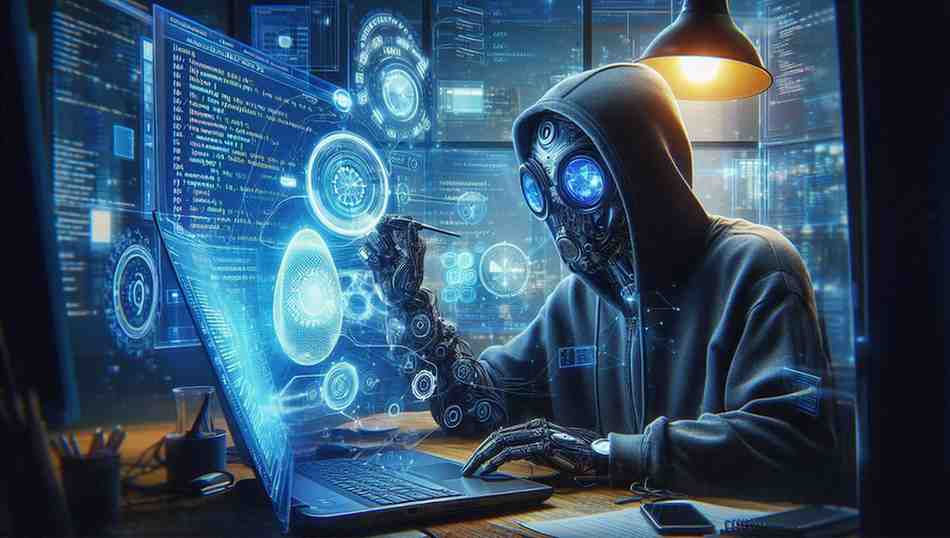
Installing Jest with npm
Before we can start writing tests, we need to install Jest in our project. Let's begin with the installation process.
Step 1: Setting Up Jest
To install Jest, you can use npm, which is the default package manager for Node.js. Run the following command in your project's root directory:
bash
npm install --save-dev jest
This command installs Jest as a development dependency, ensuring that it doesn't get included in your production build. After installation, Jest will be added to your node_modules directory, and you can start writing tests.
Step 2: Configuring Jest in package.json
Next, you'll need to add a test script to your package.json file. This script tells npm to use Jest as the test runner. Here's an example:
json
{
"scripts": {
"test": "jest"
}
}
With this configuration, you can now run your tests by executing the following command:
bash
npm test
This will invoke Jest, which will look for test files in your project and execute them.
Step 3: Writing Your First Test
Let's create a simple test to see Jest in action. Create a file named sum.test.js in your project and add the following code:
javascript
function sum(a, b) {
return a + b;
}
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
In this example, we define a sum function that adds two numbers, and then we write a test case to check if the function works correctly. The expect function is used to make assertions about the output of the sum function.
Now, run the test using npm:
bash
npm test
If everything is set up correctly, you should see the following output:
bash
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (5ms)
Congratulations! You've just written and run your first test with npm Jest. This is just the beginning—let's explore more advanced features and configurations that Jest offers.
Advanced Testing Features of Jest
Jest isn't just about running simple tests; it comes packed with powerful features that can handle complex testing scenarios. Let's dive into some of these advanced features.
Mastering Mock Functions
Mocking is a crucial feature in Jest that allows you to replace parts of your system under test with mock objects, making assertions about how they have been used. This is particularly useful for isolating your test environment from external dependencies, such as APIs or databases.
Creating a Mock Function for an API Call
Let's say you have a function that fetches data from an external API. Here's how you can mock that function using Jest:
javascript
// api.js
export const fetchData = () => {
// Imagine this fetches data from an external API
};
// api.test.js
jest.mock('./api'); // Mocking the actual fetchData function
import { fetchData } from './api';
test('mocks an API call', async () => {
fetchData.mockResolvedValue('data received');
const data = await fetchData();
expect(data).toBe('data received');
});
In this example, the fetchData.mockResolvedValue('data received') line simulates a successful API call, allowing you to test your function without actually making a network request. This approach is particularly useful when testing components or functions that depend on external services.
Asynchronous Testing Made Easy
Asynchronous code is common in JavaScript, but it can be tricky to test. Fortunately, Jest provides simple syntax to handle asynchronous operations in your tests.
Testing a Function that Returns a Promise
Let's create a function that returns a promise and test it using Jest:
javascript
function fetchUser() {
return new Promise((resolve) => {
setTimeout(() => resolve({ username: 'jestUser' }), 200);
});
}
test('fetches a user', async () => {
const user = await fetchUser();
expect(user.username).toBe('jestUser');
});
In this test, we're using async/await to handle the promise returned by fetchUser. This makes the test code clean and easy to read, while also ensuring that the function behaves as expected when the promise is resolved.
Capturing UI Changes with Snapshot Testing
Snapshot testing is a feature in Jest that allows you to ensure your UI does not change unexpectedly. It works by saving a snapshot of your UI component and comparing it against future snapshots.
Creating a Snapshot Test for a React Component
Here's how you can create a snapshot test for a React component:
javascript
import React from 'react';
import renderer from 'react-test-renderer';
import MyComponent from './MyComponent';
test('UI snapshot test', () => {
const component = renderer.create(<MyComponent />).toJSON();
expect(component).toMatchSnapshot();
});
This code creates a snapshot of MyComponent and checks if it matches the saved snapshot. If the UI changes, the test will fail, prompting you to review the changes to ensure they were intentional.
Snapshot testing is particularly useful for components with complex UIs or when you're refactoring code. It helps catch unintended changes that could affect the user experience.
Testing for Edge Cases and Error Handling
Jest also makes it easy to test for edge cases and ensure that your code handles errors gracefully.
Testing for Errors
Let's create a test case that checks if a function throws an error when given invalid input:
javascript
function divide(a, b) {
if (b === 0) {
throw new Error('Cannot divide by zero');
}
return a / b;
}
test('throws an error when dividing by zero', () => {
expect(() => divide(1, 0)).toThrow('Cannot divide by zero');
});
In this example, we use the toThrow matcher to check if the divide function throws the correct error when attempting to divide by zero. Testing for edge cases like this ensures that your code is robust and can handle unexpected inputs.
Integrate Jest for Powerful Testing
Integrating Jest with other tools and technologies can further enhance your testing strategy. Let's explore how to integrate Jest with Babel, Webpack, and Puppeteer.
Using Babel for ES6 Support
Modern JavaScript projects often use ES6 features, which may not be fully supported in all environments. To ensure compatibility, you can use Babel to transpile ES6 code to ES5, making it easier to run tests in any environment.
Installing Babel with Jest
First, install the necessary Babel packages:
bash
npm install --save-dev babel-jest @babel/core @babel/preset-env
Next, create a .babelrc file in your project's root directory with the following configuration:
json
{
"presets": ["@babel/preset-env"]
}
This setup allows Jest to use Babel for transpiling your code, ensuring that your tests run smoothly regardless of the JavaScript features used in your project.
Enhancing with Webpack
Webpack is a popular module bundler for JavaScript applications, and integrating it with Jest can optimize the testing of bundled code.
Configuring Jest to Work with Webpack
Create a jest.config.js file in your project and add the following configuration:
javascript
module.exports = {
transform: {
'^.+\\.jsx?$': 'babel-jest'
},
moduleNameMapper: {
'\\.(css|jpg|png)$': '<rootDir>/fileMock.js'
}
};
This configuration tells Jest how to handle files that Webpack would normally process, such as CSS or image files. It ensures that your tests run correctly even in projects that rely on Webpack for asset management.
Puppeteer for End-to-End Testing
For comprehensive testing that covers user interactions and browser environments, integrating Puppeteer with Jest is a powerful solution. Puppeteer is a Node library that provides a high-level API to control Chrome or Chromium.
Setting Up Puppeteer with Jest
First, install Puppeteer:
bash
npm install --save-dev puppeteer
Then, you can write an end-to-end test like this:
javascript
const puppeteer = require('puppeteer');
test('user flow test', async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Perform actions like click, type, etc.
await browser.close();
});
This code snippet illustrates a basic end-to-end test where Puppeteer opens a browser, navigates to a page, and interacts with it. Integrating Puppeteer with Jest allows you to simulate real user interactions, providing valuable insights into how users experience your application.
Overcoming Jest with npm Challenges
While Jest is a powerful tool, it’s not without its challenges. Let’s explore some common issues developers face when using Jest with npm and how to overcome them.
Tackling Jest’s Watch Mode Woes
Jest’s watch mode is designed to automatically run tests related to files that have changed since the last commit. However, it can sometimes behave unpredictably, failing to detect changes or continuously rerunning tests.
Solution: Adjusting the Watch Script
One way to ensure reliable watch mode behavior is to modify your npm script:
json
"scripts": {
"test:watch": "jest --watchAll"
}
This script tells Jest to watch all files for changes, not just those modified since the last commit. It’s a simple adjustment that can make Jest’s watch mode more predictable and effective.
Handling ES6 Imports
Jest may struggle with ES6 imports, leading to syntax errors during test runs. Integrating Babel, as discussed earlier, is crucial, but here’s a specific solution for ensuring Jest processes ES6 imports correctly.
Solution: Using Babel Plugin for CommonJS
Install the necessary Babel plugin:
bash
npm install --save-dev @babel/plugin-transform-modules-commonjs
Then, update your .babelrc file to include the plugin:
json
{
"plugins": ["@babel/plugin-transform-modules-commonjs"]
}
This configuration ensures that Jest can properly handle ES6 imports, allowing your tests to run without syntax errors.
Resolving Module Dependencies
In complex projects, Jest may have trouble resolving module dependencies, especially when dealing with custom paths or external modules.
Solution: Mapping Module Paths
Use Jest’s moduleNameMapper configuration to map custom paths to their actual locations in your project:
javascript
// jest.config.js
module.exports = {
moduleNameMapper: {
'^@components/(.*)$': '<rootDir>/src/components/$1',
'^@utils/(.*)$': '<rootDir>/src/utils/$1'
}
};
This configuration helps Jest locate and correctly import modules, ensuring that your tests run smoothly across different parts of your application.
The Essence of JavaScript Testing
Testing is an essential aspect of software development, particularly in JavaScript, where dynamic and loosely typed code can easily lead to bugs. Understanding the importance of testing and how tools like Jest and npm can help you maintain a reliable codebase is crucial for any developer.
Why Testing Matters
Testing isn’t just about catching bugs—it’s about ensuring that your code works as intended under various conditions. For JavaScript, a language used extensively on both the client and server sides, robust testing practices are essential to maintaining a stable and performant codebase.
Jest: A Testing Framework for JavaScript
Jest is more than just a testing tool—it’s a complete testing framework that simplifies the process of writing and running tests. With features like zero-configuration setup, built-in assertions, and powerful mocking capabilities, Jest is the ideal choice for testing JavaScript applications.
NPM: The Backbone of JavaScript Project Management
npm plays a crucial role in managing JavaScript projects, providing a reliable way to install dependencies, run scripts, and manage project versions. When combined with Jest, npm becomes a powerful tool for managing and executing your tests.
The Symbiosis of Jest and npm
The combination of Jest and npm offers a seamless testing experience. Jest provides the testing framework, while npm handles the dependencies and scripts necessary to run those tests. Together, they ensure that your JavaScript projects are reliable, maintainable, and ready for production.
Expanding Jest’s Horizons
As your project grows, maintaining code quality becomes increasingly important. Jest’s scalable architecture and robust feature set make it an excellent choice for large-scale applications. Let’s explore how Jest can be integrated into continuous integration (CI) environments and how it supports test-driven development (TDD).
Leveraging Jest in Large-Scale Applications
Jest is designed to handle large codebases with ease. Its ability to run tests in parallel and its efficient snapshot testing make it a great fit for large-scale projects.
Integrating Jest into Your CI/CD Pipeline
Continuous integration and continuous deployment (CI/CD) pipelines are critical for maintaining code quality in large projects. By integrating Jest into your CI/CD pipeline, you can automatically run tests every time code is pushed to your repository.
Here’s an example of a Jest test script in a GitHub Actions workflow:
yaml
name: Run Jest Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v1
with:
node-version: '14'
- name: Run Jest
run: npm test
This configuration ensures that your tests are automatically run and passed before any code is merged, reducing the likelihood of bugs in production.
Test-Driven Development with Jest
Test-driven development (TDD) is a development approach where tests are written before the code. Jest’s fast feedback loop and easy-to-read syntax make it an ideal choice for TDD, encouraging better design and more maintainable code.
Writing Tests Before Code
In a TDD workflow, you write tests that define the desired functionality before writing the actual code. Here’s an example:
javascript
test('TDD example with Jest', () => {
const result = myFunctionToTest();
expect(result).toBe(true);
});
This test defines the expected behavior of myFunctionToTest before the function is implemented. By writing the test first, you ensure that the function meets the specified requirements.
Conclusion
In this comprehensive guide, we’ve explored the powerful combination of npm Jest for testing JavaScript applications. From the initial setup and configuration to advanced features like mocking, asynchronous testing, and snapshot testing, we’ve covered everything you need to master Jest in your projects.
We’ve also looked at how to integrate Jest with tools like Babel, Webpack, and Puppeteer to create a robust and comprehensive testing suite. Additionally, we’ve discussed common challenges developers face when using Jest with npm and provided practical solutions to overcome them.
As you continue to develop your JavaScript projects, leveraging the full potential of npm Jest will ensure that your code remains reliable, maintainable, and ready for production. Whether you’re working on a small personal project or a large-scale application, mastering Jest will significantly enhance your testing strategy and improve the overall quality of your code.
Key Takeaways
Jest is a powerful and flexible testing framework for JavaScript, offering features like mocking, snapshot testing, and parallel test execution.
npm plays a crucial role in managing Jest and other dependencies, making it easier to run tests and maintain your project.
Advanced testing techniques, such as using Babel for ES6 support and Puppeteer for end-to-end testing, can enhance your testing strategy.
Overcoming common challenges with Jest, such as issues with watch mode and ES6 imports, ensures a smoother testing experience.
Integrating Jest into your CI/CD pipeline and adopting Test-Driven Development practices can improve code quality and streamline your development workflow.
FAQs
What is Jest, and why should I use it?
Jest is a JavaScript testing framework developed by Facebook. It is widely used because of its ease of use, zero-configuration setup, and rich feature set, including built-in assertions, mocking capabilities, and snapshot testing. Jest helps ensure that your code is reliable, maintainable, and bug-free.
How do I install Jest in my project?
You can install Jest using npm by running the command npm install --save-dev jest. This installs Jest as a development dependency. After installation, you can add a test script in your package.json file and run tests using the command npm test.
Can Jest be used with modern JavaScript features like ES6?
Yes, Jest can be used with ES6 and other modern JavaScript features. To ensure compatibility, you can integrate Babel with Jest, which transpiles ES6 code to ES5. This allows you to write and test modern JavaScript code while maintaining broad compatibility.
How does Jest handle asynchronous code?
Jest makes it easy to test asynchronous code using the async/await syntax or by returning promises. Jest’s API is designed to handle asynchronous operations seamlessly, allowing you to write clean and readable tests for functions that involve callbacks, promises, or async/await.
What is snapshot testing in Jest?
Snapshot testing is a feature in Jest that captures the rendered output of a component and compares it against a saved snapshot. This helps ensure that the UI does not change unexpectedly. If the UI changes, the test will fail, prompting a review of the changes.
How can I integrate Jest with CI/CD pipelines?
You can integrate Jest into CI/CD pipelines by including it in your CI configuration files. For example, in a GitHub Actions workflow, you can set up a job that runs an npm test to execute your Jest tests automatically on every push or pull request, ensuring that your code passes all tests before being merged.
What are some common challenges when using Jest, and how can I overcome them?
Common challenges include issues with Jest’s watch mode, handling ES6 imports, and resolving module dependencies. These can be addressed by adjusting npm scripts, integrating Babel with Jest, and configuring module path mappings in Jest’s configuration.
Can Jest be used for end-to-end testing?
While Jest is primarily a unit testing framework, it can be used for end-to-end testing when integrated with tools like Puppeteer. Puppeteer allows you to automate interactions with a web browser, enabling you to test user flows and interactions as part of your Jest test suite.
Further Resources for Mastering Jest and npm
To deepen your understanding of Jest and npm, and to explore more advanced topics, consider the following resources:
Jest Official Documentation: The primary source for all things Jest, from basic setup to advanced configurations.
Using npm as a Build Tool: An insightful article on leveraging npm scripts to streamline your development workflow.
Test-Driven Development for JavaScript Developers: A guide to adopting TDD practices in JavaScript projects.
CI/CD with Jest and GitHub Actions: Learn how to integrate Jest into your CI/CD pipeline using GitHub Actions.
Snapshot Testing in Jest: Detailed documentation on how to use Jest’s snapshot testing feature.
Advanced Jest Mocking Techniques: A guide to advanced mocking techniques in Jest.
Puppeteer Documentation: Official documentation for Puppeteer, covering everything you need to know about browser automation.
Webpack Documentation: Learn more about Webpack and how it integrates with Jest for testing bundled code.
Comments