Guide to Code Generators: Automate Development
- Gunashree RS
- Jul 13, 2024
- 5 min read
Updated: Aug 8, 2024
Introduction
In the ever-evolving landscape of software development, efficiency and productivity are paramount. One tool that has significantly contributed to these goals is the code generator. Code generators automate the creation of code, reducing manual effort and minimizing errors. This guide will explore the intricacies of code generators, their benefits, popular tools, and best practices. Whether you're a seasoned developer or a novice, understanding code generators can revolutionize your workflow.
What are Code Generators?
Definition and Purpose
A code generator is a tool that automatically generates source code based on predefined inputs and templates. Its primary purpose is to streamline repetitive coding tasks, ensuring consistency and accuracy while saving time.
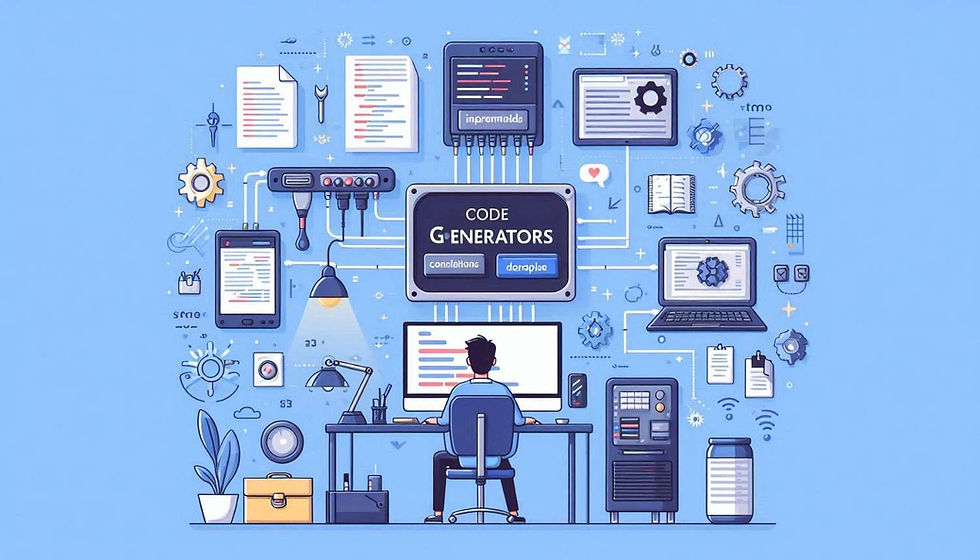
How Code Generators Work
Code generators work by taking input parameters, such as a data model or configuration file, and using them to produce source code. This process involves predefined templates and rules that dictate how the code should be structured.
Benefits of Using Code Generators
Increased Productivity
By automating repetitive tasks, code generators allow developers to focus on more complex and creative aspects of coding. This boosts overall productivity and accelerates project timelines.
Consistency and Standardization
Code generators ensure that the code adheres to predefined standards and conventions, resulting in consistent and maintainable codebases. This is particularly beneficial in large teams or projects with multiple contributors.
Reduced Errors
Manual coding is prone to human error. Code generators mitigate this risk by automating the creation process, leading to fewer bugs and issues in the generated code.
Popular Code Generators and Their Use Cases
Postman Code Generators
Postman provides a powerful set of code generators that convert Postman SDK Request Objects into code snippets in various programming languages. This is particularly useful for API testing and development.
List of Supported Code Generators in Postman
C: libcurl
C#: HttpClient, RestSharp
cURL: cURL
Dart: http
Go: Native
HTTP: HTTP
Java: OkHttp, Unirest
JavaScript: Fetch, jQuery, XHR
Kotlin: OkHttp
NodeJs: Axios, Native, Request, Unirest
Objective-C: NSURLSession
OCaml: Cohttp
PHP: cURL, Guzzle, pecl_http, HTTP_Request2
PowerShell: RestMethod
Python: http.client, Requests
R: httr, RCurl
Rust: Reqwest
Ruby: Net
Shell: Httpie, wget
Swift: URLSession
Other Popular Code Generators
Swagger Codegen: Generates API client libraries from OpenAPI specifications.
Yeoman: A scaffolding tool that helps kickstart new projects with a set of templates and best practices.
JHipster: Combines Spring Boot and Angular to generate complete web applications.
Using Code Generators in Your Development Workflow
Getting Started with Postman Code Generators
Installing Postman Code Generators
To install Postman code generators as your dependency, use npm:
sh
$ npm install postman-code-generators |
To clone the repository locally:
sh
Prerequisites
Ensure you have NodeJS (>= v8) installed. Download NodeJS from the official site.
Basic Usage
Postman code generators expose three main functions: getLanguageList, getOptions, and convert.
Example Usage
Get Language List:javascript
var codegen = require('postman-code-generators'), supportedCodegens = codegen.getLanguageList(); console.log(supportedCodegens); |
Get Options:
javascript
var codegen = require('postman-code-generators'), language = 'nodejs', variant = 'Request'; codegen.getOptions(language, variant, function (error, options) { if (error) { // handle error } console.log(options); }); |
Convert Request:
javascript
var codegen = require('postman-code-generators'), sdk = require('postman-collection'), request = new sdk.Request('https://www.google.com'), language = 'nodejs', variant = 'request', options = { indentCount: 3, indentType: 'Space', trimRequestBody: true, followRedirect: true }; codegen.convert(language, variant, request, options, function (error, snippet) { if (error) { // handle error } console.log(snippet); }); |
Best Practices for Using Code Generators
Customizing Generated Code
While code generators provide a great starting point, it's often necessary to customize the generated code to fit specific project requirements. Ensure that the generated code adheres to your project's coding standards and integrates well with existing codebases.
Regular Updates and Maintenance
Keep your code generators and templates updated to benefit from the latest features and bug fixes. Regular maintenance ensures compatibility with newer versions of programming languages and frameworks.
Thorough Testing
Always test the generated code thoroughly. Automated code generation can sometimes introduce subtle issues that need to be identified and resolved through rigorous testing.
Conclusion
Code generators are invaluable tools that can significantly enhance the efficiency and quality of your software development process. By automating repetitive tasks, ensuring consistency, and reducing errors, they free up developers to focus on more complex and creative aspects of coding. Whether you're using Postman Code Generators for API testing or other popular tools for different use cases, embracing code generation can lead to more productive and maintainable projects.
Key Takeaway
Definition and Purpose: Code generators automate code creation based on predefined inputs and templates, enhancing efficiency and reducing manual effort.
Benefits: They increase productivity by allowing developers to focus on complex tasks, ensure code consistency, and minimize errors in software projects.
Popular Tools: Postman Code Generators, Swagger Codegen, Yeoman, and JHipster are widely used for generating API clients, scaffolding projects, and integrating frameworks.
Using Postman Code Generators: Install via npm, use functions like getLanguageList, getOptions, and convert to generate code snippets tailored to different programming languages and configurations.
Best Practices: Customize generated code to fit project requirements, regularly update templates for latest features, and conduct thorough testing to ensure quality and compatibility.
Applications: Suitable for various projects but may require customization for highly specialized tasks that demand unique implementations.
Conclusion: Embracing code generators streamlines development workflows, improves code quality, and allows teams to innovate effectively in software projects.
FAQs
What is a code generator?
A code generator is a tool that automatically produces source code based on predefined templates and inputs, streamlining repetitive coding tasks.
Why should I use a code generator?
Code generators increase productivity, ensure consistency, and reduce errors by automating repetitive coding tasks.
What are some popular code generators?
Popular code generators include Postman Code Generators, Swagger Codegen, Yeoman, and JHipster.
How do I get started with Postman Code Generators?
Install the Postman Code Generators package via npm, ensure you have NodeJS installed, and use the provided functions to generate code snippets.
Can I customize the code generated by a code generator?
Yes, generated code can and should be customized to fit specific project requirements and coding standards.
Are code generators suitable for all types of projects?
While code generators are beneficial for many projects, they may not be suitable for highly specialized or unique tasks that require custom implementations.
Comments