Guide to Cell Language: Minimalist Programming
- Gunashree RS
- Jul 25, 2024
- 4 min read
Updated: Sep 22, 2024
Introduction
In the world of programming languages, simplicity and minimalism often take a backseat to complexity and feature-rich environments. However, the Cell programming language breaks this mold by offering a minimalist, easy-to-read, and educationally focused language. Designed to demonstrate how to write a programming language, Cell is a powerful tool for both novice and experienced programmers interested in understanding the fundamental concepts of language construction. This guide will take you through the intricacies of Cell, its installation, syntax, features, and much more.
What is Cell Language?
Cell Language is a minimally simple programming language created to teach the basics of writing a programming language. It includes essential features like numbers, strings, functions, and a special "None" value, making it both a functional and educational tool.
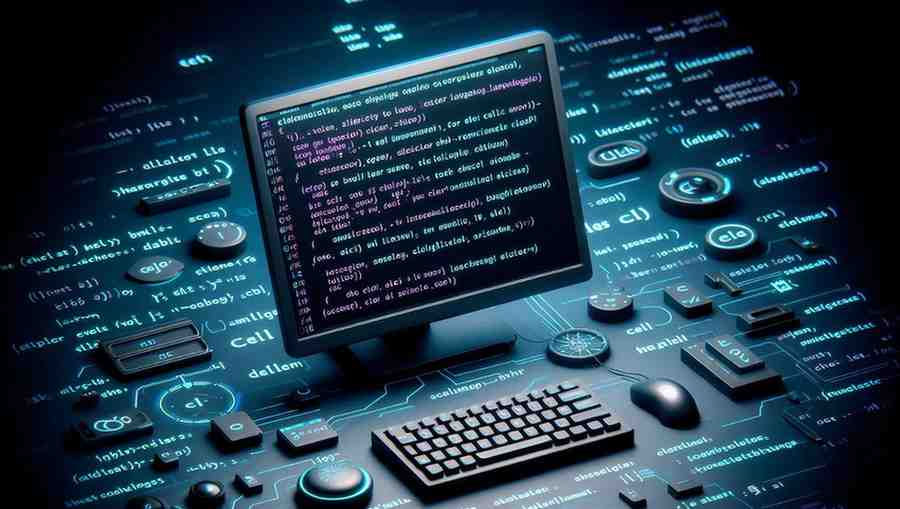
Installing Cell Language
Prerequisites
Before installing Cell, ensure you have Python 3 installed on your system. You can install Python 3 using the following command:
bash
sudo apt-get install python3 |
Installation Steps
Clone the Cell repository from GitHub:bash
git clone git@github.com:andybalaam/cell.git |
Navigate to the Cell directory:bash
cd cell |
Run the interactive environment:bash
./cell |
To run a Cell program from a file:bash
./cell filename.cell |
To run all tests:bash
make |
Key Features of Cell Language
Cell Language is designed to be as simple and complete as possible. Here are its main features:
Numbers: Floating-point numbers for arithmetic operations.
Strings: For text manipulation and output.
Functions: User-defined functions to encapsulate logic.
None Value: A special value representing the absence of value.
Understanding Cell Syntax
Basic Syntax
Cell's syntax is straightforward and minimalistic. Here is an example program in Cell:
cell
square = {:(x) x * x;}; num1 = 3; num2 = square(num1); if(equals(num1, num2), { print("num1 equals num2."); }, { print("num1 does not equal num2."); } ); |
This program defines a function to square a number, applies it, and uses an if function to compare the results.
Interactive Environment
Cell provides a REPL (Read-Eval-Print Loop) for interactive programming. Launch it by running the Cell program without arguments:
bash
./cell |
In the REPL, you can execute Cell commands directly:
cell
>>> 137 + 349; 486 >>> 5/10; 0.5 >>> 21 + 35 + 12 + 7; 75 >>> if(equals(0, 1), {"illogical";}, {"logical";}); 'logical' |
Writing Programs in Cell
Functions
Functions in Cell are defined using the {:(args) body;} syntax. Here is an example of a function that squares a number:
cell
square = {:(x) x * x;}; |
Conditional Statements
Conditional logic is handled using the if function. Here is an example:
cell
if(equals(num1, num2), { print("num1 equals num2."); }, { print("num1 does not equal num2."); } ); |
Data Structures
While Cell does not provide built-in syntax for data structures like lists or maps, you can build them using functions. For example, a simple pair data structure can be created using functions.
Building Advanced Structures in Cell
Objects
Objects in Cell can be constructed using functions, similar to how objects are created in languages like Java or C++. This allows for encapsulation and abstraction in your Cell programs.
Interpreting Cell Programs
The process of interpreting a Cell program involves several layers:
Lexer: Reads text characters and produces tokens.
Parser: Converts tokens into tree structures.
Evaluator: Executes the tree structures to produce concrete values.
Educational Resources for Cell
Cell is designed to be a learning tool. Here are some resources to help you get started:
Video Series: Detailed videos on writing a programming language.
Articles: Published articles in the Overload journal issues 145, 146, and 147.
Conference Talks: Presentations and slides from the ACCU Conference.
Conclusion
Cell Language offers a unique and educational approach to understanding the fundamentals of programming language design. Its minimalist design and simplicity make it an excellent tool for both teaching and learning. Whether you're a novice programmer or an experienced developer looking to deepen your understanding of language construction, Cell provides a clear and concise platform to explore these concepts.
Key Takeaways
Cell Language is a minimalist programming language designed for educational purposes.
It includes basic features like numbers, strings, functions, and a special "None" value.
Installation is straightforward with Python 3.
Cell supports an interactive REPL environment.
Functions and conditional logic are easy to implement.
Advanced structures can be built using Cell's functional capabilities.
Numerous educational resources are available to help you learn and understand Cell.
FAQs
What is Cell Language?
Cell Language is a minimalist programming language designed for educational purposes to demonstrate how to write a programming language.
How do I install Cell Language?
You can install Cell by cloning the GitHub repository and running the provided scripts. Ensure you have Python 3 installed on your system.
What are the main features of Cell Language?
Cell Language includes numbers, strings, functions, and a special "None" value.
How do I write a function in Cell Language?
Functions are defined using the {:(args) body;} syntax. For example, square = {:(x) x * x;};.
Does Cell Language support conditional statements?
Yes, Cell uses the if function for conditional logic.
Can I use Cell for advanced data structures?
Yes, while Cell does not have built-in syntax for data structures, you can create them using functions.
What resources are available for learning Cell Language?
There are video series, articles, and conference talks available for learning Cell.
Is Cell Language suitable for beginners?
Yes, Cell is designed to be simple and educational, making it suitable for beginners.
Comments