Cross-Platform Applications: Guide to ElectronJS Examples
- Gunashree RS
- Aug 13, 2024
- 12 min read
Introduction
In today's software development landscape, the ability to create cross-platform applications has become more important than ever. Developers are constantly seeking ways to build software that works seamlessly across different operating systems—Windows, macOS, and Linux—without needing to rewrite code for each platform.
ElectronJS has emerged as a popular solution, enabling developers to build desktop applications using familiar web technologies like JavaScript, HTML, and CSS.
ElectronJS powers some of the most popular applications in the world, from communication tools like Slack to code editors like Visual Studio Code. Its flexibility and ease of use make it a go-to choice for developers looking to create robust, cross-platform applications.
This guide will take you through a series of inspiring ElectronJS examples, showcasing how various applications are built using ElectronJS. Whether you're a seasoned developer or just getting started with ElectronJS, these examples will provide valuable insights into how to leverage ElectronJS to build your own cross-platform desktop applications.
What is ElectronJS?
ElectronJS is an open-source framework that allows developers to build desktop applications using web technologies. Created by GitHub, ElectronJS combines the Chromium rendering engine and the Node.js runtime, enabling applications to be built using HTML, CSS, and JavaScript. The result is a single codebase that can run across multiple platforms, significantly simplifying the development process.
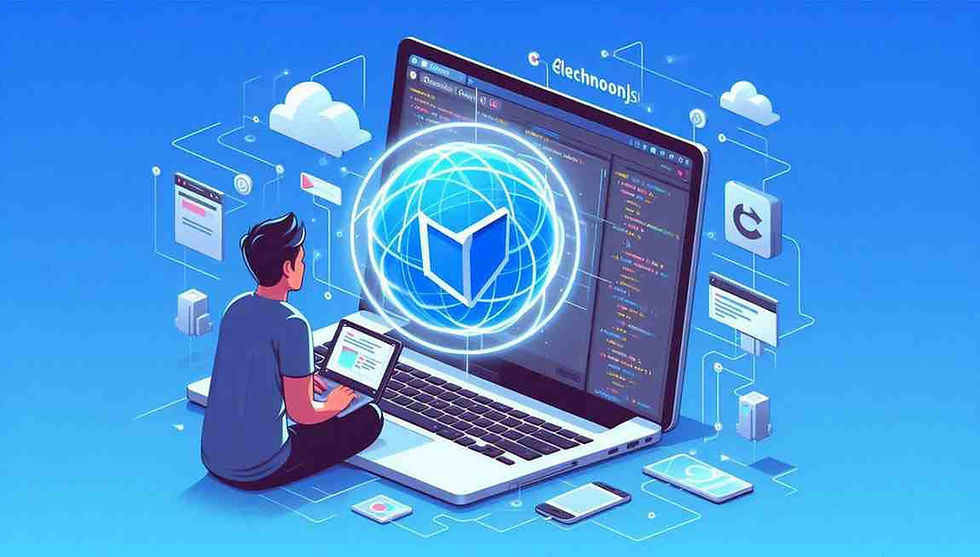
Key Features of ElectronJS
Cross-Platform Development: Write once, run anywhere. ElectronJS applications run on Windows, macOS, and Linux without the need for platform-specific code.
Web Technologies: ElectronJS uses the web technologies you're already familiar with—HTML, CSS, and JavaScript—making it accessible to a wide range of developers.
Rich Ecosystem: With access to Node.js modules, ElectronJS provides a vast array of libraries and tools to extend functionality.
Auto-Updating: ElectronJS supports automatic updates, ensuring users always have the latest version of your application.
Community Support: Backed by a strong community and widely used in the industry, ElectronJS benefits from extensive documentation, tutorials, and third-party tools.
Why Use ElectronJS?
ElectronJS is the framework of choice for many developers due to its versatility and ability to simplify cross-platform development. By using web technologies, it lowers the barrier to entry for developers who may not have experience with native desktop application development. Additionally, ElectronJS allows for rapid prototyping and iteration, making it ideal for startups and established companies alike.
Getting Started with ElectronJS
Before diving into examples, it’s essential to understand how to get started with ElectronJS. Setting up a basic ElectronJS application is straightforward, and you can have a functional app running in minutes.
Step 1: Setting Up Your Environment
To begin, you need to have Node.js installed on your system. ElectronJS relies on Node.js for backend logic and package management. If you don't have Node.js installed, you can download it from the official Node.js website.
Once Node.js is installed, you can set up a new ElectronJS project by following these steps:
Create a new directory for your project:
bash
mkdir my-electron-app
cd my-electron-app
Initialize a new Node.js project:
bash
npm init
Follow the prompts to create a package.json file.
Install Electron as a development dependency:
npm install --save-dev electron
Step 2: Creating Your First ElectronJS Application
Now that you have Electron installed, you can create a basic ElectronJS application.
Create a main.js file. This file will act as the main entry point for your application:
javascript
const { app, BrowserWindow } = require('electron');
function createWindow () {
const win = new BrowserWindow({
width: 800,
height: 600,
webPreferences: {
nodeIntegration: true
}
});
win.loadFile('index.html');
}
app.whenReady().then(createWindow);
app.on('window-all-closed', () => {
if (process.platform !== 'darwin') {
app.quit();
}
});
app.on('activate', () => {
if (BrowserWindow.getAllWindows().length === 0) {
createWindow();
}
});
Create an index.html file to be loaded by Electron:
html
<!DOCTYPE html>
<html>
<head>
<title>Hello Electron</title>
</head>
<body>
<h1>Hello, Electron!</h1>
</body>
</html>
Update your package.json to include a start script:
json
"scripts": {
"start": "electron ."
}
Run your application:
bash
npm start
You should now see a simple window with the text "Hello, Electron!". This basic setup is the foundation upon which more complex ElectronJS applications are built.
Inspiring ElectronJS Examples
Now that you have a basic understanding of ElectronJS, let’s dive into some real-world examples. These examples showcase the versatility of ElectronJS and demonstrate how it can be used to build powerful, cross-platform applications.
1. Visual Studio Code
Visual Studio Code (VS Code) is one of the most well-known ElectronJS applications. Developed by Microsoft, VS Code is a lightweight but powerful code editor that supports a wide range of programming languages and extensions.
Key Features:
Cross-Platform: Runs on Windows, macOS, and Linux.
Extensible: Supports a vast library of extensions, enabling developers to customize the editor to their needs.
Integrated Terminal: Provides a built-in terminal, allowing developers to run commands directly within the editor.
Git Integration: Built-in Git support, making it easier to manage version control within the editor.
Why It’s a Great Example: VS Code showcases the power of ElectronJS by offering a seamless experience across different operating systems. Its performance and responsiveness demonstrate how ElectronJS can be used to build complex applications without compromising on speed or functionality.
2. Slack
Slack is a popular collaboration and communication platform used by teams around the world. The desktop version of Slack is built using ElectronJS, providing users with a consistent experience across all platforms.
Key Features:
Real-Time Messaging: Supports instant messaging, file sharing, and video calls.
Integrations: Integrates with various third-party services like Google Drive, Trello, and GitHub.
Custom Workflows: Allows users to automate tasks with custom workflows.
Notifications: Provides real-time notifications, ensuring that users stay updated.
Why It’s a Great Example: Slack’s use of ElectronJS highlights its capability to handle real-time communication and large-scale applications. Despite being a web-based technology, Slack provides a native-like experience with responsive design and smooth performance.
3. Figma
Figma is a powerful design tool that allows teams to collaborate on UI/UX projects in real-time. Figma's desktop application, built with ElectronJS, brings the full functionality of its web app to a native desktop environment.
Key Features:
Real-Time Collaboration: Multiple users can work on the same design simultaneously.
Cross-Platform: Works seamlessly across Windows, macOS, and Linux.
Prototyping: Allows designers to create interactive prototypes.
Design Systems: Supports the creation and management of design systems for consistency across projects.
Why It’s a Great Example: Figma’s ElectronJS application demonstrates how complex, web-based tools can be effectively transitioned to the desktop, offering a more integrated experience for users. The application maintains high performance while enabling advanced design capabilities.
4. Discord
Discord is a communication platform primarily used by gaming communities but has since expanded to other groups. Discord’s desktop app is built with ElectronJS, providing features such as voice chat, video calls, and community management tools.
Key Features:
Voice and Video Calls: Supports high-quality voice and video communication.
Community Servers: Allows users to create and manage their own communities with channels and roles.
Screen Sharing: Users can share their screens during calls, making it ideal for collaborative gaming or working sessions.
Integrations: Discord integrates with various gaming platforms and other third-party services.
Why It’s a Great Example: Discord’s ElectronJS app is an excellent example of how the framework can be used to build a rich, interactive application with real-time communication features. Despite its complexity, the app remains performant across all platforms.
5. GitHub Desktop
GitHub Desktop is a desktop application that simplifies the use of Git and GitHub. Built with ElectronJS, it offers an intuitive interface for managing repositories, making it easier for developers to commit changes, manage branches, and collaborate with others.
Key Features:
Simple Interface: Provides a user-friendly interface for managing Git repositories.
Branch Management: Makes it easy to create, switch, and merge branches.
GitHub Integration: Direct integration with GitHub, allowing users to manage pull requests and issues from within the app.
Commit History: Visualizes commit history, making it easier to understand project progression.
Why It’s a Great Example: GitHub Desktop showcases how ElectronJS can be used to build tools that simplify complex processes like version control. Its cross-platform support ensures that developers can use the same tools regardless of their operating system.
6. Trello
Trello is a popular project management tool that uses boards, lists, and cards to help teams organize tasks and collaborate effectively. The desktop version of Trello is built using ElectronJS, bringing the web-based app’s functionality to the desktop.
Key Features:
Task Management: Allows users to create and manage tasks with cards and lists.
Collaboration: Teams can work together in real-time, with features like commenting and file sharing.
Customization: Users can customize boards with backgrounds, stickers, and power-ups.
Notifications: Provides notifications to keep users informed about task updates and deadlines.
Why It’s a Great Example: Trello’s ElectronJS app is an example of how web-based productivity tools can be adapted to the desktop environment. The application retains the intuitive interface of the web app while offering the convenience of a native desktop experience.
7. Notion
Notion is an all-in-one workspace that combines notes, tasks, databases, and collaboration tools. Notion’s desktop application, built with ElectronJS, provides a versatile platform for personal organization and team collaboration.
Key Features:
Note-Taking: Allows users to create and organize notes with rich text formatting.
Database Management: Supports the creation of databases for tracking tasks, projects, and more.
Team Collaboration: Teams can collaborate in real-time, with shared workspaces and commenting features.
Custom Templates: Users can create and share custom templates for repetitive tasks.
Why It’s a Great Example: Notion’s ElectronJS app demonstrates the framework’s ability to handle complex, multifunctional applications. The app’s smooth performance and extensive feature set make it a powerful tool for both personal and professional use.
8. WordPress Desktop
WordPress Desktop is an application that allows users to manage their WordPress sites directly from their desktop. Built with ElectronJS, it provides a streamlined experience for writing, editing, and publishing content without needing to open a web browser.
Key Features:
Content Management: Users can create, edit, and manage posts and pages from within the app.
Offline Support: The app allows users to work offline, with changes syncing automatically when reconnected.
Multi-Site Management: Users can manage multiple WordPress sites from a single application.
Notifications: Provides real-time notifications for comments, likes, and other site activities.
Why It’s a Great Example: WordPress Desktop is an excellent example of how ElectronJS can be used to bring a web-based content management system to the desktop, offering a more focused and efficient way to manage websites.
9. WhatsApp Desktop
WhatsApp Desktop is the desktop version of the popular messaging app WhatsApp. Built with ElectronJS, it mirrors the functionality of the mobile app, allowing users to send and receive messages, media, and voice notes from their desktop.
Key Features:
Messaging: Supports text messaging, voice notes, and media sharing.
Voice and Video Calls: Allows users to make voice and video calls from their desktop.
Real-Time Notifications: Provides notifications for new messages and calls.
Multi-Device Support: Syncs with the mobile app, allowing users to switch between devices seamlessly.
Why It’s a Great Example: WhatsApp Desktop showcases how ElectronJS can be used to extend the functionality of a popular mobile app to the desktop, providing users with a consistent experience across devices.
10. Spotify Desktop
Spotify Desktop is the desktop version of the widely-used music streaming service Spotify. Built using ElectronJS, the app provides access to Spotify’s vast music library, playlists, and personalized recommendations.
Key Features:
Music Streaming: Users can stream millions of songs, albums, and playlists.
Personalized Recommendations: Provides music recommendations based on listening history.
Offline Playback: Premium users can download music for offline listening.
Cross-Platform: Syncs with the mobile app, allowing users to switch between devices without losing their place.
Why It’s a Great Example: Spotify Desktop is a prime example of how ElectronJS can be used to deliver a rich multimedia experience on the desktop, with smooth performance and a user-friendly interface.
Advanced ElectronJS Techniques
As you become more familiar with ElectronJS, you may want to explore advanced techniques to enhance your applications further. These techniques can help you optimize performance, improve security, and add new functionality.
Optimizing Performance in ElectronJS Applications
While ElectronJS is powerful, it’s essential to optimize performance to ensure that your applications run smoothly, especially when handling resource-intensive tasks.
Use BrowserWindow Wisely
The BrowserWindow object is central to ElectronJS applications, but it can consume significant resources. To optimize performance:
Minimize the Number of BrowserWindows: Only create new windows when necessary. Consider using tabs or sections within a single window instead of multiple windows.
Manage Memory Usage: Monitor and manage memory usage by unloading or minimizing windows that are not in use.
Enable Hardware Acceleration
ElectronJS supports hardware acceleration, which can improve performance for graphics-intensive applications. Ensure that hardware acceleration is enabled:
javascript
const { app } = require('electron');
app.disableHardwareAcceleration(); // Disable if needed for specific use cases
In most cases, you should keep hardware acceleration enabled, but you can disable it if it causes issues on specific systems.
Improving Security in ElectronJS Applications
Security is crucial, especially for applications that handle sensitive data. ElectronJS provides several tools to enhance security.
Use Context Isolation
Context isolation ensures that the renderer process (where your app’s UI runs) is isolated from the main process. This reduces the risk of cross-site scripting (XSS) attacks:
javascript
const win = new BrowserWindow({
webPreferences: {
contextIsolation: true
}
});
Disable Node.js Integration in Renderer
By default, ElectronJS allows Node.js integration in the renderer process. For security reasons, it’s often advisable to disable this:
javascript
const win = new BrowserWindow({
webPreferences: {
nodeIntegration: false
}
});
Disabling Node.js integration reduces the attack surface, making your application more secure.
Adding Native Features with ElectronJS
ElectronJS allows you to extend your applications with native features, providing a more integrated experience on each platform.
System Tray Integration
ElectronJS supports system tray integration, allowing your application to run in the background with a tray icon.
javascript
const { app, Tray, Menu } = require('electron');
let tray = null;
app.whenReady().then(() => {
tray = new Tray('path/to/icon.png');
const contextMenu = Menu.buildFromTemplate([
{ label: 'Item1', type: 'radio' },
{ label: 'Item2', type: 'radio' }
]);
tray.setToolTip('This is my application.');
tray.setContextMenu(contextMenu);
});
This feature is useful for applications that need to run continuously or offer quick access to features without opening the main window.
Custom Native Menus
ElectronJS allows you to create custom native menus for your application, offering a more consistent experience across platforms.
javascript
const { Menu } = require('electron');
const template = [
{
label: 'File',
submenu: [
{ label: 'New', click: () => { console.log('New File'); } },
{ label: 'Open', click: () => { console.log('Open File'); } },
]
}
];
const menu = Menu.buildFromTemplate(template);
Menu.setApplicationMenu(menu);
Custom menus can enhance usability and make your application feel more integrated into the user’s operating system.
Conclusion
ElectronJS has revolutionized the way developers build cross-platform desktop applications. By enabling the use of familiar web technologies like JavaScript, HTML, and CSS, ElectronJS lowers the barrier to entry for desktop development while offering the flexibility and power needed to create complex, feature-rich applications.
The examples covered in this guide—from Visual Studio Code to Spotify Desktop—demonstrate the versatility and robustness of ElectronJS. These applications highlight how ElectronJS can be used to build everything from simple tools to large-scale, enterprise-grade software.
As you explore ElectronJS further, you’ll find that it’s not just a framework for building apps; it’s a platform that empowers developers to create seamless, integrated experiences across multiple operating systems. Whether you’re building your first ElectronJS app or looking to optimize an existing one, the techniques and examples provided here will serve as a valuable resource on your journey.
Key Takeaways
ElectronJS is a powerful framework for building cross-platform desktop applications using web technologies like JavaScript, HTML, and CSS.
Real-world examples such as Visual Studio Code, Slack, and Figma demonstrate ElectronJS’s versatility and capability to handle complex applications.
Optimizing performance and improving security are crucial for ensuring that your ElectronJS applications run smoothly and securely.
Advanced features like system tray integration and custom native menus can enhance the user experience and make your applications feel more integrated into the user’s operating system.
FAQs
What is ElectronJS?
ElectronJS is an open-source framework that allows developers to build cross-platform desktop applications using web technologies like JavaScript, HTML, and CSS. It combines the Chromium rendering engine and Node.js runtime to enable web applications to run as native desktop apps.
Why is ElectronJS popular for cross-platform development?
ElectronJS is popular because it simplifies cross-platform development by allowing developers to write a single codebase that works on Windows, macOS, and Linux. This reduces development time and costs while providing a consistent user experience across all platforms.
Can ElectronJS applications be optimized for performance?
Yes, ElectronJS applications can be optimized for performance through various techniques such as minimizing the number of BrowserWindow instances, managing memory usage, enabling hardware acceleration, and using context isolation for security.
Are ElectronJS applications secure?
While ElectronJS provides tools to enhance security, such as context isolation and disabling Node.js integration in the renderer process, it’s important for developers to follow best practices to mitigate security risks, especially when handling sensitive data.
What are some well-known ElectronJS applications?
Some well-known ElectronJS applications include Visual Studio Code, Slack, Figma, Discord, GitHub Desktop, Trello, Notion, WordPress Desktop, WhatsApp Desktop, and Spotify Desktop.
How does ElectronJS handle cross-platform compatibility?
ElectronJS handles cross-platform compatibility by using the Chromium rendering engine and Node.js runtime, which work consistently across Windows, macOS, and Linux. This allows developers to write code once and have it run on all supported platforms.
Can I integrate native features into my ElectronJS application?
Yes, ElectronJS allows you to integrate native features such as system tray icons, custom native menus, and notifications, providing a more integrated experience for users on each platform.
What are the challenges of using ElectronJS?
Some challenges of using ElectronJS include managing performance, ensuring security, and handling large application sizes due to the inclusion of Chromium and Node.js. However, these challenges can be mitigated with proper optimization and best practices.
Comentarios