Guide to Compilation in Java: From Code to Execution
- Gunashree RS
- Jul 6, 2024
- 5 min read
Updated: Sep 22, 2024
Introduction
Java's "write once, run anywhere" principle has made it a preferred language for developers around the world. This versatility is largely attributed to its unique compilation process, which transforms human-readable code into platform-independent bytecode. In this detailed guide, we will explore the Java compilation process, breaking down each step and explaining the significance of the Java Virtual Machine (JVM). Whether you're new to Java or an experienced developer, understanding this process is crucial for writing efficient and portable code.
Understanding the Java Compilation Process
The Java compilation process involves converting Java source code into an intermediate form known as bytecode, which is then executed by the JVM. This two-step approach ensures that Java applications can run on any device equipped with a compatible JVM, making Java a truly platform-independent language.
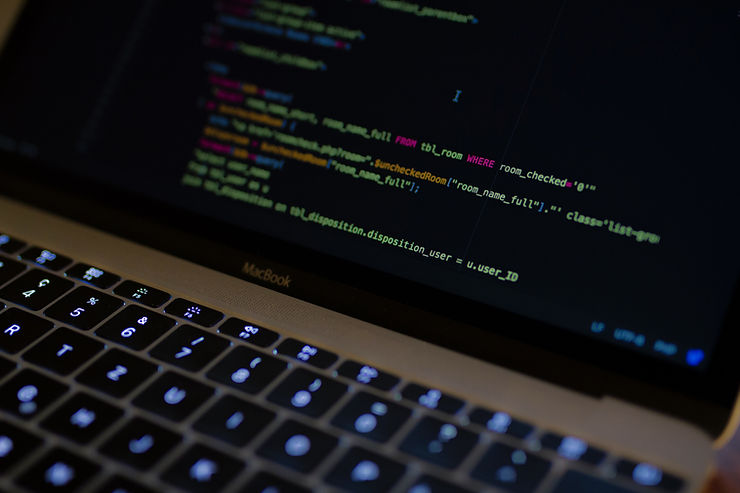
Steps in the Java Compilation Process
1. Writing Source Code
The process begins with writing Java source code in files with the .java extension. This code is written in a human-readable language and contains the logic and instructions for the application.
2. Compiling Source Code
Using the Java compiler (javac), the source code is converted into bytecode, which is stored in .class files. The compiler checks for syntax errors and performs optimizations during this stage.
Command:
bash:
javac MyClass.java |
---|
3. Generating Bytecode
Bytecode is a set of instructions that the JVM interprets and executes. It is platform-independent, meaning the same bytecode can run on any device with a compatible JVM.
Compilation vs. Interpretation in Java
Java employs both compilation and interpretation to execute programs. Understanding the differences between these processes helps in grasping the full execution mechanism of Java applications.
Compilation:
Converts Java source code into bytecode.
Done once during the development process.
Allows for code optimization.
Interpretation:
JVM interprets and executes bytecode.
Occurs each time the program is run.
Offers flexibility and dynamic behavior.
Role of the Java Virtual Machine (JVM)
The JVM is a crucial component in the Java compilation process. It loads, verifies, and executes Java bytecode, providing a runtime environment with features like memory management and security. Each platform has its own JVM implementation, ensuring that Java applications can run anywhere without modification.
Key Functions of the JVM:
Class Loading: Loads compiled .class files.
Bytecode Verification: Ensures bytecode adheres to Java's security constraints.
Execution: Interprets and executes bytecode or compiles it to native machine code using Just-In-Time (JIT) compilation.
Just-In-Time (JIT) Compilation
JIT compilation is an optimization technique used by the JVM to enhance performance. It compiles bytecode into native machine code at runtime, allowing the program to run faster.
Benefits of JIT Compilation:
Improved Performance: Converts frequently executed bytecode to native code, reducing interpretation overhead.
Adaptive Optimization: Optimizes code based on runtime profiling.
Common Tools for Java Compilation
Several tools and Integrated Development Environments (IDEs) streamline the Java compilation process, offering features like code completion, debugging, and build automation.
Popular IDEs:
Eclipse
IntelliJ IDEA
NetBeans
Build Automation Tools:
Apache Maven
Gradle
Best Practices for Java Compilation
Consistent Code Style: Use a linter to maintain consistent coding standards.
Error Handling: Implement comprehensive error handling and logging.
Modular Architecture: Structure your code into modules for better maintainability.
Continuous Integration: Use CI tools to automate testing and deployment.
Challenges in Java Compilation
Compilation Errors: Syntax and semantic errors can prevent successful compilation.
Performance Overheads: JIT compilation can introduce initial performance overhead.
Platform-Specific Issues: While Java is platform-independent, JVM-specific bugs can arise.
Future Trends in Java Compilation
Enhanced JIT Techniques: Advancements in JIT compilation for better performance.
Static Analysis Tools: Improved tools for detecting errors and optimizing code before compilation.
Cross-Compilation: Tools for compiling Java code to run on non-JVM platforms.
Conclusion
Understanding the Java compilation process is fundamental for any Java developer. From writing source code to generating bytecode and leveraging the JVM for execution, each step plays a pivotal role in creating platform-independent applications. By mastering these concepts, developers can optimize their code, troubleshoot errors more effectively, and build robust, efficient, and scalable software solutions. Continual learning and practice in Java compilation serve as the cornerstone for developing high-quality Java applications.
Key Takeaways from "The Comprehensive Guide to Compilation in Java"
Java Compilation Process: Java converts source code into bytecode, which is then executed by the JVM, enabling platform independence.
Steps in Compilation: Includes writing Java code, compiling with javac, and generating bytecode .class files.
Compilation vs. Interpretation: Java uses both to optimize performance and maintain flexibility.
Role of JVM: Crucial for loading, verifying, and executing bytecode across different platforms.
JIT Compilation: JIT optimizes bytecode into native machine code at runtime for enhanced performance.
Tools for Java Compilation: IDEs like Eclipse, and IntelliJ IDEA, and build automation tools such as Apache Maven streamline development.
Best Practices: Emphasizes consistent coding style, error handling, modular architecture, and continuous integration.
Challenges: Includes handling compilation errors, JIT overhead, and platform-specific issues.
Future Trends: Focus on enhanced JIT techniques, static analysis tools, and cross-compilation for non-JVM platforms.
Frequently Asked Questions (FAQs) Related to Java Compilation Process
What is the objective of Java compilation?
The objective of Java compilation is to convert source code written in human-readable form into bytecode, which can be executed by the Java Virtual Machine (JVM). This process ensures that the code can run on any platform with a compatible JVM.
What are the primary steps in the Java compilation process?
The primary steps include writing Java source code, compiling the code using the Java compiler (javac), which produces bytecode (.class files), and finally executing the bytecode using the JVM.
What is bytecode, and why is it essential in Java?
Bytecode is an intermediate set of instructions generated by the Java compiler that the JVM can interpret and execute. It is essential because it allows Java programs to be platform-independent, enabling them to run on any device with a compatible JVM.
Can Java code run without compilation?
No, Java code must be compiled into bytecode (.class files) before it can be executed by the JVM.
Are there any tools available to aid in the Java compilation process?
Yes, several Integrated Development Environments (IDEs) like Eclipse, IntelliJ IDEA, and NetBeans provide tools that streamline the Java compilation process, offering features like code completion, debugging, and seamless compilation. Additionally, build automation tools like Apache Maven and Gradle can automate compilation tasks.
What role does the Java Virtual Machine (JVM) play in the compilation process?
The JVM is responsible for executing Java bytecode. It translates bytecode into machine-specific instructions, allowing Java programs to run on various platforms without modification.
Comments